Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial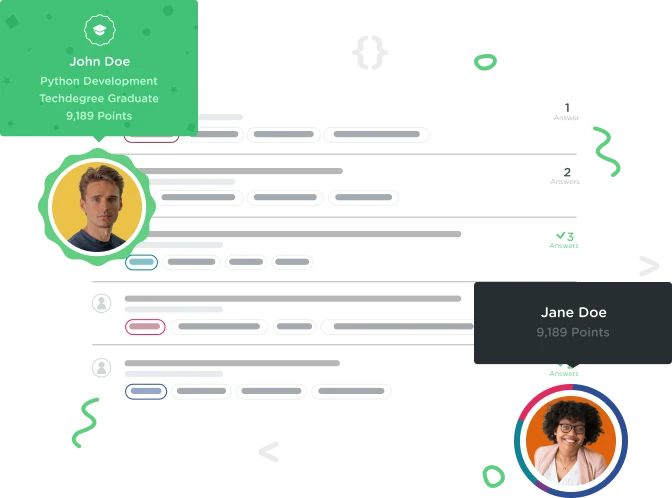
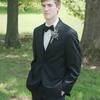
Jamison Imhoff
12,460 PointsDungeon game syntax error
SEE below for updated post
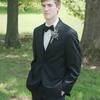
Jamison Imhoff
12,460 Pointsi have now finished part 2 and this is the updated code:
import random
CELLS = [(0, 0), (0, 1), (0, 2),
(1, 0), (1, 1), (1, 2),
(2, 0), (2, 1), (2, 2)]
def get_locations():
monster = random.choice(CELLS)
door = random.choice(CELLS)
start = random.choice(CELLS)
if monster == door or monster == start or door ==start:
return monster, door, start
def move_player(player, move):
x, y = player
if move == 'LEFT':
y -= 1
elif move == 'RIGHT':
y+= 1
elif move == 'UP':
x -= 1
elif move == 'DOWN':
x += 1
return x, y
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
if player[1] == 0:
moves.remove('LEFT')
if player[1] == 2:
moves.remove('RIGHT')
if player[0] == 0:
moves.remove('UP')
if player[0] == 2:
moves.remove('DOWN')
return moves
def draw_map():
print(' _ _ _')
tile = '|{}'
for idx, cell in enumerate(CELLS):
if idx in [0, 1, 3, 4, 6, 7]:
if cell == player:
print(tile.format('x'), end='')
else:
print(tile.format('_'), end='')
else:
if cell == player:
print(tile.format('x|'))
else:
print(tile.format('_|'))
monster, door, player = get_locations()
print("Welcome to the dungeon!")
while True:
moves = get_moves(player)
print("You're currently in room {}".format(player))
draw_map(player)
print("You can move".format(moves))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
if move in moves:
player = move_player(player, move)
else:
print("** Walls are hard, stop walking into them! **")
continue
if player == door:
print("You Escaped!")
break
elif player == monster:
print("You were eaten by the grue!")
break
now I am getting syntax error :
Traceback (most recent call last):
File "dungeon.py", line 60, in <module>
monster, door, player = get_locations()
TypeError: 'NoneType' object is not iterable
not really sure what to do...???
4 Answers

Thomas Kirk
5,246 PointsTake player out of draw_map(player), it doesn't take any arguments.
Also, below that, you are missing {} in:
print("You can move ".format(moves))
As is, it will just print 'You can move', without giving the options.
That ought to do it!
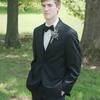
Jamison Imhoff
12,460 Pointsthank you so much. that fixed it! it works perfectly now!

Thomas Kirk
5,246 PointsI noticed in the code you posted, that there is a missing closing parenthesis on the line above where all your issues are arising:
print("You're currently in room {}".format(player)
This line needs a second ')' to close the print function.
Could this be the culprit?
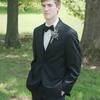
Jamison Imhoff
12,460 Pointstried that still error... now i have posted the complete code and it is giving a different error... not sure why
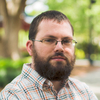
Kenneth Love
Treehouse Guest TeacherYour get_locations()
function is wrong. If any of the things are the same (the line with all of the ==
s), you should return the function again. Outside of that, if they're not the same, you return the values that you randomly chose.
def get_locations():
# stuff here for getting random locations
if monster == door or monster == player or player == door:
return get_locations()
return monster, door, player
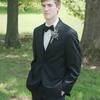
Jamison Imhoff
12,460 Pointsi changed this but now I am getting a different error
import random
CELLS = [(0, 0), (0, 1), (0, 2),
(1, 0), (1, 1), (1, 2),
(2, 0), (2, 1), (2, 2)]
def get_locations():
monster = random.choice(CELLS)
door = random.choice(CELLS)
start = random.choice(CELLS)
if monster == door or monster == player or player == door:
return get_locations()
return monster, door, player
def move_player(player, move):
x, y = player
if move == 'LEFT':
y -= 1
elif move == 'RIGHT':
y+= 1
elif move == 'UP':
x -= 1
elif move == 'DOWN':
x += 1
return x, y
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
if player[1] == 0:
moves.remove('LEFT')
if player[1] == 2:
moves.remove('RIGHT')
if player[0] == 0:
moves.remove('UP')
if player[0] == 2:
moves.remove('DOWN')
return moves
def draw_map():
print(' _ _ _')
tile = '|{}'
for idx, cell in enumerate(CELLS):
if idx in [0, 1, 3, 4, 6, 7]:
if cell == player:
print(tile.format('x'), end='')
else:
print(tile.format('_'), end='')
else:
if cell == player:
print(tile.format('x|'))
else:
print(tile.format('_|'))
monster, door, player = get_locations()
print("Welcome to the dungeon!")
while True:
moves = get_moves(player)
print("You're currently in room {}".format(player))
draw_map(player)
print("You can move".format(moves))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
if move in moves:
player = move_player(player, move)
else:
print("** Walls are hard, stop walking into them! **")
continue
if player == door:
print("You Escaped!")
break
elif player == monster:
print("You were eaten by the grue!")
break
the error now is:
Traceback (most recent call last):
File "dungeon.py", line 61, in <module>
monster, door, player = get_locations()
File "dungeon.py", line 13, in get_locations
if monster == door or monster == player or player == door:
NameError: name 'player' is not defined
I dont understand...I thought I was following the video accurately, but these errors keep popping up >_<

Thomas Kirk
5,246 PointsYou need to change:
start = random.choice(CELLS)
to
player = random.choice(CELLS)
I believe Kenneth changes everything from start to player at some point during the videos.
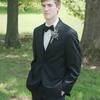
Jamison Imhoff
12,460 Pointsok THANK YOU. I missed that I guess. Sometimes it's hard to keep up with the changes because the videos are pretty fast moving... also when you look over something its easy to miss stuff... That suggestion fixed that error BUT now there is ANOTHER error >_>
i know right... sorry I really must have messed this up lol but now when I run the file I get :
Welcome to the dungeon!
You're currently in room (0, 1)
Traceback (most recent call last):
File "dungeon.py", line 69, in <module>
draw_map(player)
TypeError: draw_map() takes 0 positional arguments but 1 was given
Jamison Imhoff
12,460 PointsJamison Imhoff
12,460 PointsUPDATE:
I tried to just make the line :
print("You can move")
and it still said there was a syntax error???
so i deleted the line, and then the next line was syntax error??? so I deleted THAT line and then the " move = input("> ") " is giving syntax error?!?!?
WHAT IS HAPPENING? I am so confused now.