Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial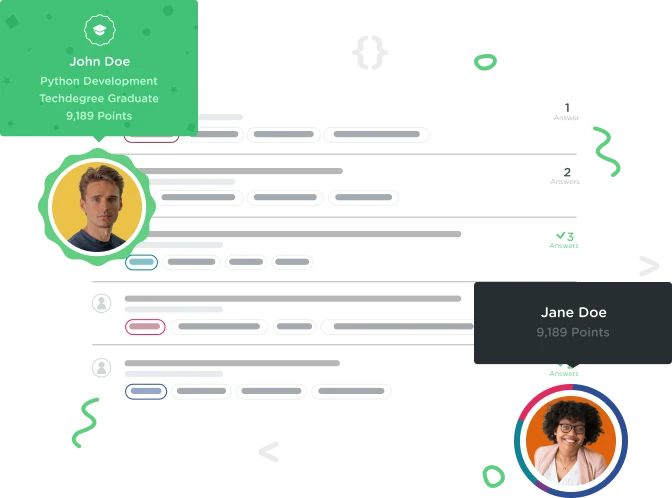

Keith Cox
Courses Plus Student 1,291 PointsDynamically changing background color Android Java
Goal is to change the background color of mealLayout.
Not sure how to get past this challenge.... Please explain changes and why the changes are made.... Thank you.
import android.os.Bundle; import android.widget.TextView;
public class MealActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_meal);
TextView foodLabel = (TextView) findViewById(R.id.foodTextView);
TextView drinkLabel = (TextView) findViewById(R.id.drinkTextView);
RelativeLayout mealLayout = (RelativeLayout) findViewById(R.id.mealLayout);
mealLayout.setBackgroundColor(Color.RED);
}
}
3 Answers

Kate Hoferkamp
5,205 PointsYou have the correct code, just the wrong color. It wants you to set the background color to Green not red.
mealLayout.setBackgroundColor(Color.GREEN);
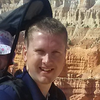
Rob Mount
8,658 PointsI'm not sure what you're asking. Did you also import RelativeLayout? if in Eclipse, you can manage your imports automatically by pressing CTRL+SHIFT+O.
I created a project that meets what you've presented and the background changes to red from onCreate(). Are you interested in how to change the color based on user interaction or to continuously change as the activity remains running? If you just want the background to change continuously automatically, here's an option:
(Note, this method will create a background thread as a timer)
import java.util.Random;
import java.util.Timer;
import java.util.TimerTask;
import android.app.Activity;
import android.graphics.Color;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.RelativeLayout;
import android.widget.TextView;
public class MainActivity extends Activity {
//Move your declarations here if you intend on using them after the onCreate() method
private TextView foodLabel;
private TextView drinkLabel;
private RelativeLayout mealLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Inflate your objects
foodLabel = (TextView) findViewById(R.id.food);
drinkLabel = (TextView) findViewById(R.id.drink);
mealLayout = (RelativeLayout) findViewById(R.id.layout);
//We change the color to RED for the first time as the program loads
mealLayout.setBackgroundColor(Color.RED);
//Create the timer object which will run the desired operation on a schedule or at a given time
Timer timer = new Timer();
//Create a task which the timer will execute. This should be an implementation of the TimerTask interface.
//I have created an inner class below which fits the bill.
MyTimer mt = new MyTimer();
//We schedule the timer task to run after 1000 ms and continue to run every 1000 ms.
timer.schedule(mt, 1000, 1000);
}
//An inner class which is an implementation of the TImerTask interface to be used by the Timer.
class MyTimer extends TimerTask {
public void run() {
//This runs in a background thread.
//We cannot call the UI from this thread, so we must call the main UI thread and pass a runnable
runOnUiThread(new Runnable() {
public void run() {
Random rand = new Random();
//The random generator creates values between [0,256) for use as RGB values used below to create a random color
//We call the RelativeLayout object and we change the color. The first parameter in argb() is the alpha.
mealLayout.setBackgroundColor(Color.argb(255, rand.nextInt(256), rand.nextInt(256), rand.nextInt(256) ));
}
});
}
}
Hope this helps.

Kate Hoferkamp
5,205 PointsHe is doing a code challenge, like in Treehouse, not eclipse.
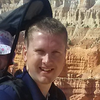
Rob Mount
8,658 PointsGotcha, sorry. I haven't done that challenge. I've found some of those challenges to be rather picky in their result expectations. GRR!
Have a good one.

Keith Cox
Courses Plus Student 1,291 PointsThanks to Rob and Kate.... I appreciate you being there. :)

Andreas Robichaux
2,426 PointsIm trying to make something where I have different links and depending on the link it loads into an imageView below the header called subBanner into a solid color, rather than putting an image file to load. Could I achieve the same thing using this code
webColor.setBackgroundColor(Color."#C41E72");
in the eventclickListener of my first activity?? and then when the second activity loads it will pass in that color into the imageview?
Keith Cox
Courses Plus Student 1,291 PointsKeith Cox
Courses Plus Student 1,291 PointsThanks Kate.... I seem to got stuck in the training text and got a mental block on the actual request.... :)
Kate Hoferkamp
5,205 PointsKate Hoferkamp
5,205 PointsYeah I hate when that happens! Glad you got it though!