Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial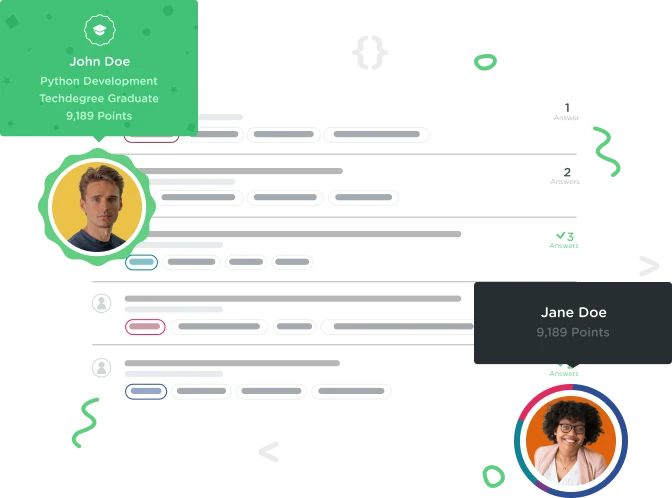

Kevin Wehmueller
10,116 Points'Else if' clause overriding 'if' clause where it shouldn't
Hi all, I had a great time coming up with a solution to this challenge. However, I'm running into a slight problem, described in the comment multi-line comment below:
/*
An ASOIAF-themed Quiz!
Users will be asked five questions
Each question has one correct answer, and one nearly correct answer which will prompt the user to try again
Each correct answer should increase the counter by one and print a message to the document
Each incorrect answer should print a message to the document
At the end of the quiz, a message should be printed to the document ranking the user with one of four awards:
5 - The crown of the King of the Andals, the Rhoynar, and the First Men
3-4 - The crown of the Kings in the North
1-2 - The crown of Salt and Rock
0 - No award! Try again next time!
Have fun, and leave me feedback in comments!
***One problem I'm experiencing: the if clause within the 'Try Again' if clauses in questions 2-5 isn't running; i.e., it's being overridden by the else if clauses
If the user only has 1 question correct, the alert will still read 'So far, you have gotten 1 answers right' rather than 'you have gotten 1 answer right'. Any thoughts on a fix?
*/
var correctGuess = false;
var answersRight = 0;
// Question 1
var questionOne = prompt( 'Welcome to the ultimate ASOIAF Quiz! Your first question is: How many direwolves \do the Stark children receive?' );
if ( parseInt(questionOne) === 6 || questionOne.toUpperCase() === 'SIX' ) {
correctGuess = true;
answersRight += 1;
alert('You got the first answer right! So far, you have gotten ' + answersRight + ' answer right!');
document.write('1. You got that answer right!<br>');
} else if ( parseInt(questionOne) === 5 || questionOne.toUpperCase() === 'FIVE' ) {
var guessOneAgain = prompt('Common mistake! Why don\'t you try again? Hint: I\'m counting Jon\'s direwolf');
if ( parseInt(guessOneAgain) === 6 || guessOneAgain.toUpperCase() === 'SIX' ) {
correctGuess = true;
answersRight += 1;
alert('You got the first answer right! So far, you have gotten ' + answersRight + ' answer right!');
document.write('1. You got that answer right!<br>');
} else {
document.write ('1. Sorry, you got that answer wrong!<br>');
}
} else {
document.write ('1. Sorry, you got that answer wrong!<br>');
}
// Question 2
var questionTwo = prompt( 'Next question! Where is the seat of House Martell?' );
if ( questionTwo.toUpperCase() === 'SUNSPEAR' ) {
correctGuess = true;
answersRight += 1;
alert('You got the second answer right! So far, you have gotten ' + answersRight + ' answers right!');
document.write('2. You got that answer right!<br>');
} else if ( questionTwo.toUpperCase() === 'HIGHGARDEN' ) {
var guessTwoAgain = prompt( 'I said "Martell," not "Tyrell"! Guess again!' );
if ( guessTwoAgain.toUpperCase() === 'SUNSPEAR' ) {
correctGuess = true;
answersRight += 1;
if ( answersRight === 1 ) {
alert('You got the second answer right! So far, you have gotten ' + answersRight + ' answer right!');
document.write('2. You got that answer right!<br>');
} else if ( answersRight >= 2 ) {
alert('You got the second answer right! So far, you have gotten ' + answersRight + ' answers right!');
document.write('2. You got that answer right!<br>');
}
} else {
document.write ('2. Sorry, you got that answer wrong!<br>');
}
} else {
document.write ('2. Sorry, you got that answer wrong!<br>');
}
// Question 3
var questionThree = prompt( 'What is the Ghiscari word for "Mother"?' );
if ( questionThree.toUpperCase() === 'MHYSA' ) {
correctGuess = true;
answersRight += 1;
alert('You got the third answer right! So far, you have gotten ' + answersRight + ' answers right!');
document.write('3. You got that answer right!<br>');
} else if ( questionThree.toUpperCase() === 'DRANE') {
var guessThreeAgain = prompt( 'You speak excellent Dothraki, but how\'s your Ghiscari? Try again!' );
if ( guessThreeAgain.toUpperCase() === 'MHYSA' ) {
correctGuess = true;
answersRight += 1;
if ( answersRight === 1 ) {
alert('You got the third answer right! So far, you have gotten ' + answersRight + ' answer right!');
document.write('3. You got that answer right!<br>');
} else if ( answersRight >= 2 ) {
alert('You got the third answer right! So far, you have gotten ' + answersRight + ' answers right!');
document.write('3. You got that answer right!<br>');
}
} else {
document.write ('3. Sorry, you got that answer wrong!<br>');
}
} else {
document.write ('3. Sorry, you got that answer wrong!<br>');
}
// Question 4
var questionFour = prompt( 'Who is known as "The King Beyond the Wall"?' );
if ( questionFour.toUpperCase() === 'MANCE RAYDER' ) {
correctGuess = true;
answersRight += 1;
alert('You got the fourth answer right! So far, you have gotten ' + answersRight + ' answers right!');
document.write('4. You got that answer right!<br>');
} else if ( questionFour.toUpperCase() === 'THE NIGHT KING' ) {
var guessFourAgain = prompt( 'Well, you\'re not wrong, but let\'s aim for a little more human and a little less genocidal.' );
if ( guessFourAgain.toUpperCase() === 'MANCE RAYDER' ) {
correctGuess = true;
answersRight += 1;
if ( answersRight === 1 ) {
alert('You got the fourth answer right! So far, you have gotten ' + answersRight + ' answer right!');
document.write('4. You got that answer right!<br>');
} else if ( answersRight >= 2 ) {
alert('You got the fourth answer right! So far, you have gotten ' + answersRight + ' answers right!');
document.write('4. You got that answer right!<br>');
}
} else {
document.write ('4. Sorry, you got that answer wrong!<br>');
}
} else {
document.write ('4. Sorry, you got that answer wrong!<br>');
}
// Question 5
var questionFive = prompt( 'In what year did Aegon I Targaryen\'s reign begin? Hint: BC and AC' );
if ( questionFive.toUpperCase() === '1 AC' ) {
correctGuess = true;
answersRight += 1;
alert('You got the fifth answer right! So far, you have gotten ' + answersRight + ' answers right!');
document.write('5. You got that answer right!<br>');
} else if ( questionFive.toUpperCase() === '0 AC' ) {
var guessFiveAgain = prompt( 'Almost! The Wars of Conquest began in 2 BC and lasted two years, but that\'s not the "beginning" of Aegon\'s reign.' );
if ( guessFiveAgain.toUpperCase() === '1 AC' ) {
correctGuess = true;
answersRight += 1;
if ( answersRight === 1 ) {
alert('You got the fifth answer right! So far, you have gotten ' + answersRight + ' answer right!');
document.write('5. You got that answer right!<br><br>');
} else if ( answersRight >= 2 ) {
alert('You got the fifth answer right! So far, you have gotten ' + answersRight + ' answers right!');
document.write('5. You got that answer right!<br><br>');
}
} else {
document.write ('5. Sorry, you got that answer wrong!<br><br>');
}
} else {
document.write ('5. Sorry, you got that answer wrong!<br><br>');
}
//Quiz Summary
if ( answersRight === 5 ) {
document.write('You got every question right! You get the crown of the King of the Andals, the Rhoynar, and the First Men!');
} else if ( answersRight === 3 || answersRight === 4 ) {
document.write('You <em>almost</em> had it! You get the crown of the Kings in the North!');
} else if ( answersRight === 1 || answersRight === 2 ) {
document.write('You can do better! You get the crown of Salt and Rock \(but really it\'s just made of driftwood\)!');
} else {
document.write('Try harder next time! Remember, in the game of thrones, you win or you die!');
}
7 Answers

Erik Nuber
20,629 Pointsnot odd, I figured it out...
The code is running as you have it...however...
var questionThree = prompt( 'What is the Ghiscari word for "Mother"?' );
if ( questionThree.toUpperCase() === 'MHYSA' ) {
correctGuess = true;
answersRight += 1;
alert('You got the third answer right! So far, you have gotten ' + answersRight + ' answers right!');
document.write('3. You got that answer right!<br>');
} else if ( questionThree.toUpperCase() === 'DRANE') {
var guessThreeAgain = prompt( 'You speak excellent Dothraki, but how\'s your Ghiscari? Try again!' );
if ( guessThreeAgain.toUpperCase() === 'MHYSA' ) {
correctGuess = true;
answersRight += 1;
if ( answersRight === 1 ) {
alert('You got the third answer right! So far, you have gotten ' + answersRight + ' answer right!');
document.write('3. You got that answer right!<br>');
} else if ( answersRight >= 2 ) {
alert('You got the third answer right! So far, you have gotten ' + answersRight + ' answers right!');
document.write('3. You got that answer right!<br>');
}
} else {
document.write ('3. Sorry, you got that answer wrong!<br>');
}
} else {
document.write ('3. Sorry, you got that answer wrong!<br>');
}
I tried it over and over and noticed that if I got the wrong answer the first couple of times and then put the try again answer it worked properly for this question. If I put in the right answer to begin with I got the incorrect spelling.
If you look at your code it is working exactly that way.
Notice that if I put in "MHYSA" as the answer it says alert("with answers")
however if i guess Drane and then Mhysa to get it right it has a different alert.
So it has to do with which order you answer in.
This could be solved by adding in a function that you pass answersRight too and then gives an alert
function checkAnswer(answerRight) {
if (parseInt(answerRight) === 1) {
alert('You got the answer right! So far, you have gotten ' + answersRight + ' answer right!');
} else if (answersRight > 1) {
alert('You got the answer right! So far, you have gotten ' + answersRight + ' answers right!');
}
}
var questionThree = prompt( 'What is the Ghiscari word for "Mother"?' );
if ( questionThree.toUpperCase() === 'MHYSA' ) {
correctGuess = true;
answersRight += 1;
checkAnswer(answersRight);
document.write('3. You got that answer right!<br>');
} else if ( questionThree.toUpperCase() === 'DRANE') {
var guessThreeAgain = prompt( 'You speak excellent Dothraki, but how\'s your Ghiscari? Try again!' );
if ( guessThreeAgain.toUpperCase() === 'MHYSA' ) {
correctGuess = true;
answersRight += 1;
if ( answersRight === 1 ) {
checkAnswer(answersRight); document.write('3. You got that answer right!<br>');
} else if ( answersRight >= 2 ) {
checkAnswer(answersRight);
document.write('3. You got that answer right!<br>');
}
} else {
document.write ('3. Sorry, you got that answer wrong!<br>');
}
} else {
document.write ('3. Sorry, you got that answer wrong!<br>');
}

Erik Nuber
20,629 Pointsvar questionNumber = 0; //added to top with other variables
function checkAnswer(answerRight) {
if (parseInt(answerRight) === 1) {
alert('You got the answer right! So far, you have gotten ' + answersRight + ' answer right!');
} else if (answersRight > 1) {
alert('You got the answer right! So far, you have gotten ' + answersRight + ' answers right!');
}
}
function rightAnswer(questionNumber) {
document.write(questionNumber + '. You got that answer right!<br>');
}
function wrongAnswer(questionNumber) {
document.write(questionNumber + '. You got that answer wrong!<br>');
}
var questionThree = prompt( 'What is the Ghiscari word for "Mother"?' );
if ( questionThree.toUpperCase() === 'MHYSA' ) {
correctGuess = true;
questionNumber += 1;
answersRight += 1;
checkAnswer(answersRight);
rightAnswer(questionNumber);
} else if ( questionThree.toUpperCase() === 'DRANE') {
var guessThreeAgain = prompt( 'You speak excellent Dothraki, but how\'s your Ghiscari? Try again!' );
if ( guessThreeAgain.toUpperCase() === 'MHYSA' ) {
correctGuess = true;
answersRight += 1;
if ( answersRight === 1 ) {
checkAnswer(answersRight);
rightAnswer(questionNumber);
} else if ( answersRight >= 2 ) {
checkAnswer(answersRight);
rightAnswer(questionNumber);
}
} else {
wrongAnswer(questionNumber);
}
} else {
wrongAnswer(questionNumber);
}
cleaned it up a bit more to use functions. Not necessary but a bit more DRY.

Kevin Wehmueller
10,116 PointsThanks for all your help!

Erik Nuber
20,629 PointsI just noticed that I used both answerRight and answersRight in the function...that would have to be cleaned so only one is used if you decide to go this route.

Erik Nuber
20,629 PointsThe problem is that you are adding +1 to to answers right so it is increasing in number you need to check for some other condition.
In other words, it will work for whichever question you get right first after that answerRight is >1 so will not be === to 1 and won't work.

Kevin Wehmueller
10,116 PointsI want it to work only once, just in case a user gets the first (or first two, three, etc.) questions wrong. Unfortunately it's not even working for the first question a user gets right. I'm sure there's a better way to do this, but for now I suspect it could work.

Erik Nuber
20,629 PointsYou will find a cleaner way of doing this when you get to arrays and objects. A two dimensional array or an array of objects would clean up your code a lot. There is no reason it wouldn't work as you have it. I will look thru again and see, I notice that I was actually wrong as you make the comparison each time on correctAnswer based on a running total.

Erik Nuber
20,629 PointsOk, I just verified in the console, I tried getting answers right, wrong and for the try again. It works. I ran each snippet and then ran the entire thing and it appears to be working.

Kevin Wehmueller
10,116 PointsNo matter at which point I enter my first correct answer, I will only ever see the result of the else if clause:
if ( answersRight === 1 ) {
alert('You got the fourth answer right! So far, you have gotten ' + answersRight + ' answer right!');
document.write('4. You got that answer right!<br>');
} else if ( answersRight >= 2 ) {
alert('You got the fourth answer right! So far, you have gotten ' + answersRight + ' answers right!');
document.write('4. You got that answer right!<br>');
The difference between the if and else if clauses is only a single letter, but the alert "You got the xth answer right! So far, you have gotten 1 answers right" makes my eye twitch.
No matter which question I'm answering, the first one I get right should always say "answer," not "answers"

Erik Nuber
20,629 Pointslet me try again...I didn't notice that I was looking for overall working...but yeah that is a difference...

Erik Nuber
20,629 PointsJust did it again and watched for that. Missed the first two questions and got the next two correct and it was properly worded without the s and then with the s.

Erik Nuber
20,629 Pointstried a couple more times and, if I miss the first three and get the fourth right I get the wrong statement...

Kevin Wehmueller
10,116 PointsI've tried it every which way and I get the wrong statement every time. Quite odd.