Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial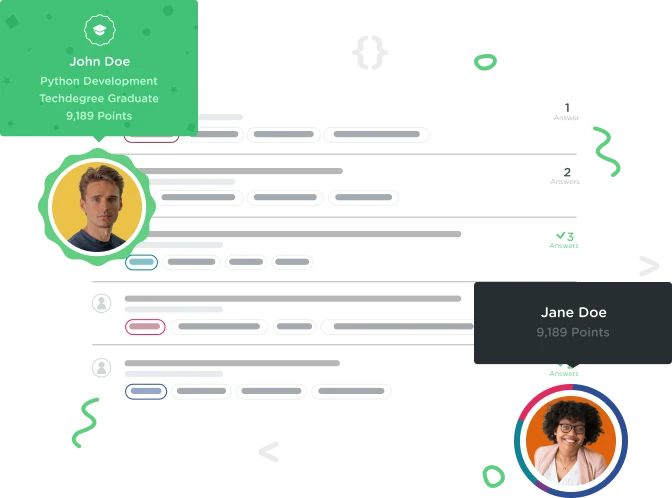
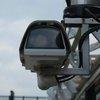
Bruce Röttgers
18,211 PointsError: 'Too many values to unpack'
On line 6 (for loop) it says too many values to unpack, so this is probably the false route, but I don't really get it what I have as other options,
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(itera_1, itera_2):
buffer = []
for first, second in itera_1, itera_2:
buffer.append((first, second))
return buffer
1 Answer

Kent Åsvang
18,823 PointsThere is a lot of cool solutions to this. I would use the zip-function myself:
def combo(a, b):
return list(zip(a, b))
The zip function takes two iterables, and returns an iterable of tuples with the corresponding values. You can read about it here zip-function
Bruce Röttgers
18,211 PointsBruce Röttgers
18,211 PointsThe challenge shouldn't be solved using zip() (and can't)
Kent Åsvang
18,823 PointsKent Åsvang
18,823 PointsI'm not a paying student, so I can't look into the challenge and didn't know you weren't allowed to use the zip-function. Anyway, here is another solution you could try:
Hope this helped then. Sorry for the initial mistake. And in case you wan't a pretty oneliner, you could use this: