Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial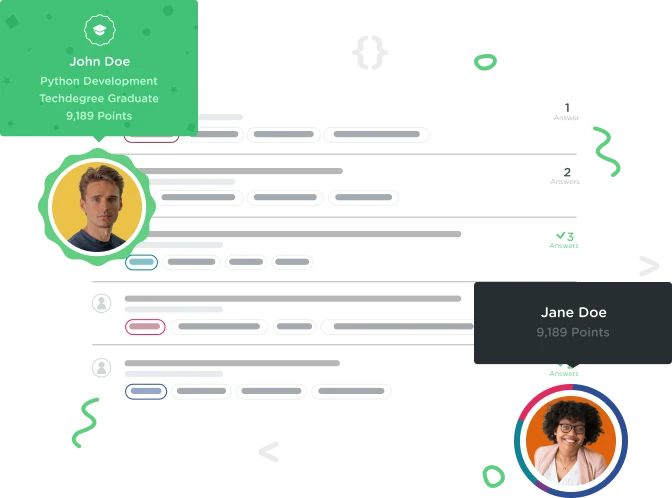

Kyle Brown
5,314 Pointserror when todolist.count eq(0)
error message: http://gyazo.com/05e7d8a267e3199783f1ea35bf1c6802
code: http://gyazo.com/8eba564bb532e4b5776320cb495b1802
localhost display: http://gyazo.com/bbf0d067cb5873e9dfc7b8edf84db0e0
expect(Todolist.count).to eq(0)
error: uninitialized constant Todolist
i have tried many variations of the "constant" todolist but none work. TodoLists todolists Todolists Todo_lists todo_lists
Todo_list todo_list etc...
5 Answers
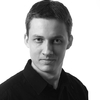
Maciej Czuchnowski
36,441 PointsOK, so there are a few things wrong with your spec (and possibly code itself), these are the first two that will help you with the problem described:
Line 15:
expect(TodoIist.count).to eq(0)
I know that with some fonts this looks like L
in the word "list", but is actually a capital i
. You can notice this with serif fonts (like above). Change it to this:
expect(Todolist.count).to eq(0)
Line 27:
expect(TodoList.count).to eq(0)
You have capital L, but you need lower case l, because you did not follow Jason's code precisely before when generating scaffolds, so from now on every time he writes TodoList
, you have to write Todolist
.
Now you will start getting different failures and hopefully these are covered in the videos.
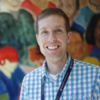
Brandon Barrette
20,485 PointsSo you need to use camel case. So instead of Todolist, you need:
expect(TodoList.count).to eq(0)
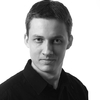
Maciej Czuchnowski
36,441 PointsHe seems to be using model name without the camel case (without underscore in controller name).

Kyle Brown
5,314 Pointsapp/models/todolist.rb file
class Todolist < ActiveRecord::Base
validates :title, presence: true
end
spec/models/todolist_spec.rb
require 'rails_helper'
RSpec.describe Todolist, :type => :model do
pending "add some examples to (or delete) #{__FILE__}"
end
i haven't done anything in the controller but
require 'rails_helper'
# This spec was generated by rspec-rails when you ran the scaffold generator.
# It demonstrates how one might use RSpec to specify the controller code that
# was generated by Rails when you ran the scaffold generator.
#
# It assumes that the implementation code is generated by the rails scaffold
# generator. If you are using any extension libraries to generate different
# controller code, this generated spec may or may not pass.
#
# It only uses APIs available in rails and/or rspec-rails. There are a number
# of tools you can use to make these specs even more expressive, but we're
# sticking to rails and rspec-rails APIs to keep things simple and stable.
#
# Compared to earlier versions of this generator, there is very limited use of
# stubs and message expectations in this spec. Stubs are only used when there
# is no simpler way to get a handle on the object needed for the example.
# Message expectations are only used when there is no simpler way to specify
# that an instance is receiving a specific message.
RSpec.describe TodolistsController, :type => :controller do
# This should return the minimal set of attributes required to create a valid
# Todolist. As you add validations to Todolist, be sure to
# adjust the attributes here as well.
let(:valid_attributes) {
skip("Add a hash of attributes valid for your model")
}
let(:invalid_attributes) {
skip("Add a hash of attributes invalid for your model")
}
# This should return the minimal set of values that should be in the session
# in order to pass any filters (e.g. authentication) defined in
# TodolistsController. Be sure to keep this updated too.
let(:valid_session) { {} }
describe "GET index" do
it "assigns all todolists as @todolists" do
todolist = Todolist.create! valid_attributes
get :index, {}, valid_session
expect(assigns(:todolists)).to eq([todolist])
end
end
describe "GET show" do
it "assigns the requested todolist as @todolist" do
todolist = Todolist.create! valid_attributes
get :show, {:id => todolist.to_param}, valid_session
expect(assigns(:todolist)).to eq(todolist)
end
end
describe "GET new" do
it "assigns a new todolist as @todolist" do
get :new, {}, valid_session
expect(assigns(:todolist)).to be_a_new(Todolist)
end
end
describe "GET edit" do
it "assigns the requested todolist as @todolist" do
todolist = Todolist.create! valid_attributes
get :edit, {:id => todolist.to_param}, valid_session
expect(assigns(:todolist)).to eq(todolist)
end
end
describe "POST create" do
describe "with valid params" do
it "creates a new Todolist" do
expect {
post :create, {:todolist => valid_attributes}, valid_session
}.to change(Todolist, :count).by(1)
end
it "assigns a newly created todolist as @todolist" do
post :create, {:todolist => valid_attributes}, valid_session
expect(assigns(:todolist)).to be_a(Todolist)
expect(assigns(:todolist)).to be_persisted
end
it "redirects to the created todolist" do
post :create, {:todolist => valid_attributes}, valid_session
expect(response).to redirect_to(Todolist.last)
end
end
describe "with invalid params" do
it "assigns a newly created but unsaved todolist as @todolist" do
post :create, {:todolist => invalid_attributes}, valid_session
expect(assigns(:todolist)).to be_a_new(Todolist)
end
it "re-renders the 'new' template" do
post :create, {:todolist => invalid_attributes}, valid_session
expect(response).to render_template("new")
end
end
end
describe "PUT update" do
describe "with valid params" do
let(:new_attributes) {
skip("Add a hash of attributes valid for your model")
}
it "updates the requested todolist" do
todolist = Todolist.create! valid_attributes
put :update, {:id => todolist.to_param, :todolist => new_attributes}, valid_session
todolist.reload
skip("Add assertions for updated state")
end
it "assigns the requested todolist as @todolist" do
todolist = Todolist.create! valid_attributes
put :update, {:id => todolist.to_param, :todolist => valid_attributes}, valid_session
expect(assigns(:todolist)).to eq(todolist)
end
it "redirects to the todolist" do
todolist = Todolist.create! valid_attributes
put :update, {:id => todolist.to_param, :todolist => valid_attributes}, valid_session
expect(response).to redirect_to(todolist)
end
end
describe "with invalid params" do
it "assigns the todolist as @todolist" do
todolist = Todolist.create! valid_attributes
put :update, {:id => todolist.to_param, :todolist => invalid_attributes}, valid_session
expect(assigns(:todolist)).to eq(todolist)
end
it "re-renders the 'edit' template" do
todolist = Todolist.create! valid_attributes
put :update, {:id => todolist.to_param, :todolist => invalid_attributes}, valid_session
expect(response).to render_template("edit")
end
end
end
describe "DELETE destroy" do
it "destroys the requested todolist" do
todolist = Todolist.create! valid_attributes
expect {
delete :destroy, {:id => todolist.to_param}, valid_session
}.to change(Todolist, :count).by(-1)
end
it "redirects to the todolists list" do
todolist = Todolist.create! valid_attributes
delete :destroy, {:id => todolist.to_param}, valid_session
expect(response).to redirect_to(todolists_url)
end
end
end
sorry i'm new to this site but thanks for the help.

Kyle Brown
5,314 Pointscode
require 'rails_helper'
describe "Creating todo lists" do
it "redirects to the todolist index page on success" do
visit "/todolists"
click_link "New Todolist"
expect(page).to have_content("New todolist")
fill_in "Title", with: "my todo list"
fill_in "Description", with: "this is what im doing today."
click_button "Create Todolist"
expect(page).to have_content("my todo list")
end
it "displays an error when the todo list has no title" do
expect(Todolist.count).to eq(0)
visit "/todolists"
click_link "New Todolist"
expect(page).to have_content("New todolist")
fill_in "Title", with: "my todo list"
fill_in "Description", with: "this is what im doing today."
click_button "Create Todolist"
expect(page).to have_content("my todo list")
expect(page).to have_content("error")
expect(Todolist.count).to eq(0)
visit "/todolists"
expect(page).to_not have_content("this is what im doing today.")
end
end

Kyle Brown
5,314 Pointsok i add the project on github and the camel case didnt work https://github.com/bricknovax/todo
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsPlease show more code: your model and controller at least. It would be preferable to paste the code here (formatting using Markdown) or even put it on github and link here.