Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial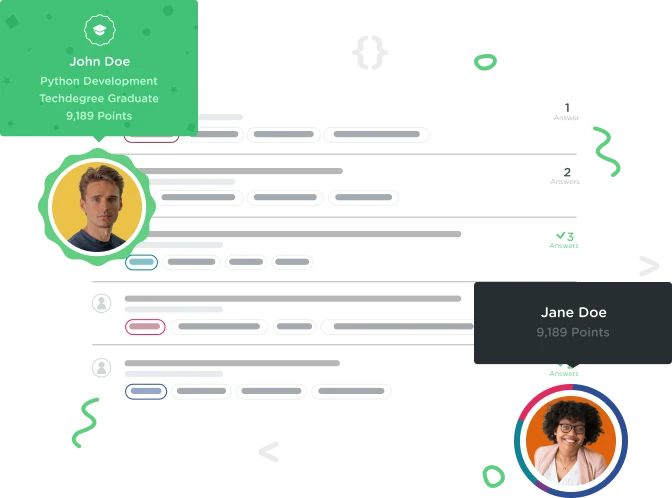

Whitney Kenny
445 PointsError while running the app I'm getting a error while running the app "unfortunately Fun facts has stopped"
package com.example.whitney.funfacts;
import android.os.Bundle; import android.support.design.widget.FloatingActionButton; import android.support.design.widget.Snackbar; import android.support.v7.app.AppCompatActivity; import android.support.v7.widget.Toolbar; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.TextView;
public class FunFactsActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
final TextView factLabel = (TextView) findViewById(R.id.Facttextview);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String fact = "Ostriches can run faster than horses";
factLabel.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
FloatingActionButton fab = (FloatingActionButton) findViewById(R.id.fab);
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Snackbar.make(view, "Replace with your own action", Snackbar.LENGTH_LONG)
.setAction("Action", null).show();
}
});
}
6 Answers
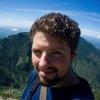
Garrett Carver
14,681 PointsHi Whitney,
I would first try removing this code, since I believe you have the action bar removed now:
setSupportActionBar(toolbar);
FloatingActionButton fab = (FloatingActionButton) findViewById(R.id.fab);
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Snackbar.make(view, "Replace with your own action", Snackbar.LENGTH_LONG)
.setAction("Action", null).show();
}
});
If that doesn't work, I would skip ahead a bit to where debugging is explained in this section
If you are still having trouble, ask me again and I could take a look at your project files.
Dont give up!
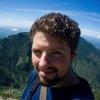
Garrett Carver
14,681 PointsDo you see any red marks or anything in your code? If so, mouse over it and it should pop up something explaining the problem. If you select it, and hit alt+ enter, it should give you some possible solutions. Can you try that and post it here if you learn anything new?
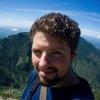
Garrett Carver
14,681 PointsOne thing I just noticed when I compared your code to the project files:
@Override
public void onClick(View v) {
should be:
@Override
public void onClick(View view) {

Whitney Kenny
445 PointsOkay this is the error after undoing everything , hopefully you can see it . The error is on the line for the button It says cannot resolve showfactbutton.. When I click alt+enter my options are as followed : create id value resource , create field and rename
TextView factLabel = (TextView) findViewById(R.id.Facttextview);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
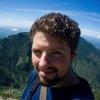
Garrett Carver
14,681 Pointstry deleting (R.id.showFactButton)
and retype it. Let the autocorrect select the correct id.
Hope that works!

Whitney Kenny
445 PointsWow thank you very much I got it running again! , I will try to figure out the other steps now
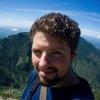
Garrett Carver
14,681 PointsSweet! I found that Android Studio will always link to your objects properly if you use the Auto correct feature. So use it as much as possible! Happy coding!
PS- Could you give my answer an up-vote?
Cheers, Garrett

Whitney Kenny
445 PointsOkay I will edit that
Whitney Kenny
445 PointsWhitney Kenny
445 PointsThank you but unfortunately that did not work package com.example.whitney.funfacts;
import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.TextView;
public class FunFactsActivity extends AppCompatActivity {