Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial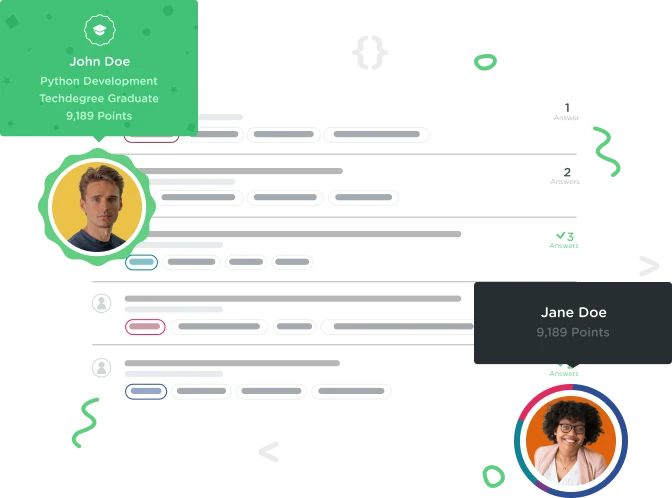

blckphoenix8
2,946 PointsEven Odd Loop Code
The code I wrote for this exercise runs properly in workspace but the exercise says I'm wrong and can't continue. What is it looking for that isn't in my code? Thanks.
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start != 0:
num = random.randint(1, 99)
if even_odd(num):
print("{} is even.".format(num))
else:
print("{} is odd.".format(num))
start -= 1
3 Answers
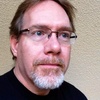
Chris Freeman
Treehouse Moderator 68,441 PointsThe checker doesn't like the period at the end of the print strings.
print("{} is even".format(num))
print("{} is odd".format(num))

Byron Rodriguez
Courses Plus Student 3,771 PointsThank you for your response Chris. I see what I was doing wrong. I was checking if "even_odd(num) != 0". I didn't realize that the function would return True or False.

Evan Waterfield
3,240 PointsCan someone explain the start aspect and return not num % 2? I know that's vague but I'm having a hard time with these challenges. I understand the num statement, the if, and else.
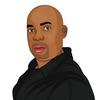
Quinton Gordon
10,985 PointsHey Evan,
The start variable is being using the count the number of loops. So for every completed loop the value is start is being decreased by 1 and when the value is 0 the loop will stop due to start no longer being "truthy".
The return statement is also a matter of truth logic. So the function checks to see if a number is even by dividing it by 2. Any even number divided by 2 leaves a remainder of 0. The problem the 'not' is solving is that 0 is a "falsey" statement. So without the not the function would be responding that the number is not an even when it is. So we inverse the response.
Byron Rodriguez
Courses Plus Student 3,771 PointsByron Rodriguez
Courses Plus Student 3,771 PointsI keep getting "Bummer Try again!". I copied and pasted the code above which was the same as the code I wrote but still getting the error. This is frustrating!
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsMy print statements are meant to replace your print statements. Here is the full code: