Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial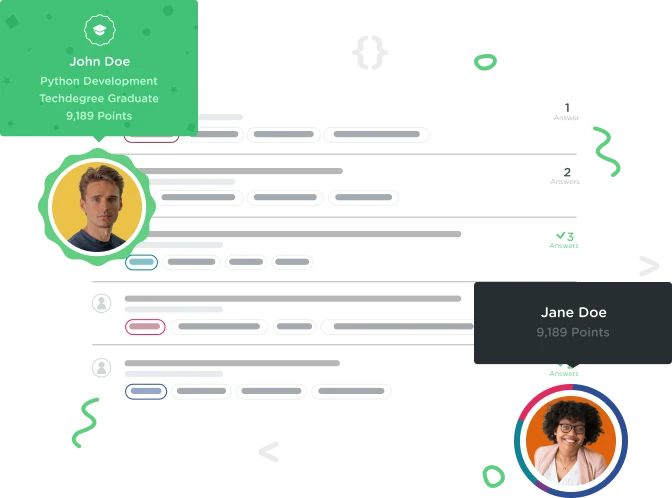
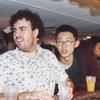
Brent Liang
Courses Plus Student 2,944 PointsEven or odd game index error
import random
def even_odd(num):
return not num % 2
even_odd()
start = 5
while start is True:
ran_num = random.randint(1,99)
try:
even_odd(ran_num)
if True:
print("{} is odd".format(ran_num))
else:
print("{} is even".format(ran_num))
start -= 1
3 Answers
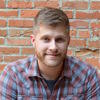
Peter Lawless
24,404 PointsYou got a few things going on here. First, I see you call even_odd with no argument in line 5. I expect that would raise an error.
Next you assign the variable "start" a value of 5. That's good, but then the condition for your while loop is "while start is True". First, I don't think you want to use the 'is' keyword here. Use conditional operators like '==', '>', '<', etc. Second, since start is an integer, it cannot be a boolean value (True or False) at the same time. That being said, integers can be 'truthy' or 'falsy', but that is another topic.
Next, you have a 'try:' statement with no 'except:' statement to follow. I don't think that's necessary here since you know that ran_num is an integer and even_odd() is designed to accept integers. There should be no chance for error to raise through that function. The omission of an "except" will raise an error, also.
Next, you need to assign the output of even_odd(ran_num) to a variable so you can actually assess its value. Otherwise, the function runs, and the output goes nowhere. Something like:
x = even_odd(ran_num)
if x:
print("{} is even".format(ran_num))
else:
print("{} is odd".format(ran_num))
It seems your conditions were backwards, too. The function even_odd() will return True for even numbers and False for odd numbers.
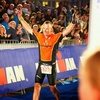
Steve Hunter
57,712 PointsWe seem to be on the same page with those responses!
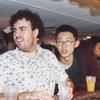
Brent Liang
Courses Plus Student 2,944 PointsHey Peter, thanks so much for your help.
It works.
Have a wonderful day!
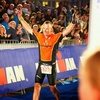
Steve Hunter
57,712 PointsHi Brent,
I think you have the even/odd outputs the wrong way round? If even_odd()
returns True
the number is even. You have that outputting the opposite.
Also, remove the line where the function is called just after it is defined - you don't want even_odd()
just sitting there.
That should get you through - let me know if not.
Steve.
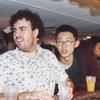
Brent Liang
Courses Plus Student 2,944 PointsHey Steve, thanks for such a professional/succinct answer!
I would've chosen yours as the best answer. I really would. But Peter has a cooler last name :)
Thanks so much!!
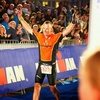
Steve Hunter
57,712 PointsHey, no worries. I don't need the points!
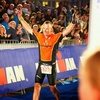
Steve Hunter
57,712 PointsThere's a couple of other points too ...
You don't want to try
anything - just test the returned value of even_odd
in the if
statement.
And the while
loop doesn't like is True
as its condition. Change that to testing is start
is greater than zero. You end up with this:
while start > 0:
ran_num = random.randint(1, 99)
if even_odd(ran_num):
print("{} is even".format(ran_num))
else:
print("{} is odd".format(ran_num))
start -= 1
That should now work for you.
Steve.
Brent Liang
Courses Plus Student 2,944 PointsBrent Liang
Courses Plus Student 2,944 PointsKeeps getting index error in the last line?