Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial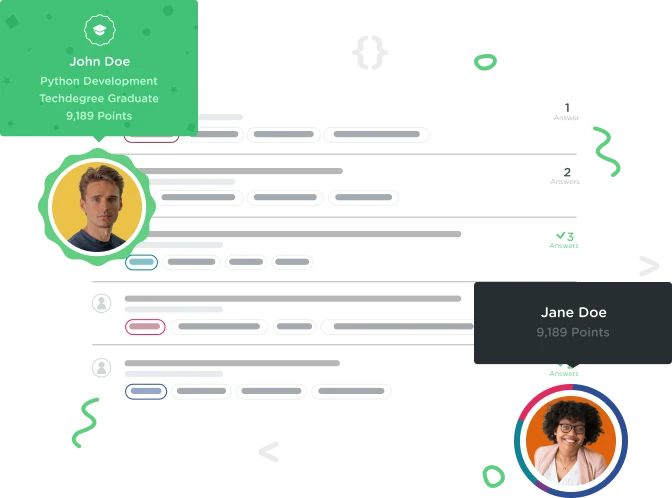
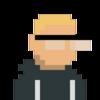
Everton Carneiro
15,994 PointsEvery player must have a unique id or the ids must follow the number of players in the array?
I always like trying to build the code before see the teacher solution and in the case of handleAddPlayer method, I did like this:
handleAddPlayer = (name) => {
this.setState({
players: [
...this.state.players,
{
name: name,
score: 0,
id: this.state.players.length + 1
}
]
});
}
This way, I make sure that the player id will be always the last item of the array. But seeing the teacher's solution, I notice that he did in a way that all players have a unique id, because if you delete two players before add one, the id will remain 5 for the added player. My question is: it`s a requirement that the players have unique ids in this case?
3 Answers

tomd
16,701 PointsI mean in the real world, yes. You want objects to have unique ID's. In databases id's auto-increment. If something gets deleted, another object doesn't take its place.

KRIS NIKOLAISEN
54,970 PointsRemoving a player is based on id so yes they need to be unique.
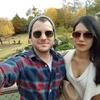
Jamie Gobeille
Full Stack JavaScript Techdegree Graduate 19,573 PointsYes. In react, every object needs to have its own id or react will throw a warning. I believe it does this so the framework can differentiate and keep tabs on each item's state.
I wasn't a fan of Guil's solution for making the unique id so here is my solution! Each time a player is added to the array, the lastPlayerId variable will get the id of the last item in the array. Then when the id is set, it adds 1 to the id. This way the numbers don't increment in an odd manner and the id will always be unique.
addPlayer = (name) => {
let lastPlayerId = this.state.players.slice(-1)[0].id;
this.setState({
players: [
...this.state.players,
{
name,
score: 0,
id: (lastPlayerId += 1),
},
],
});
};
Everton Carneiro
15,994 PointsEverton Carneiro
15,994 PointsI got it. Thank you!