Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial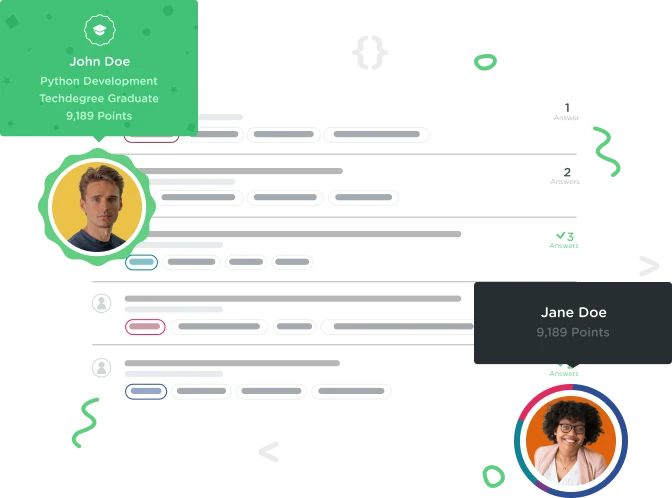

Kevin Lankford
1,983 Pointsexample saying my code is returning the wrong results
I've created several different permutations of this code but I'm not finding success. In my initial solution I created:
def add(a,b):
return float(a + b)
but I seem to be getting a ValueError saying a multi decimal is not working. That prompted me to add an exception and write the code like so:
def add(a,b):
try:
return float(a + b)
except ValueError:
print("invalid input")
I'm not quite understanding why it's telling me add is returning the wrong results if its converting it into a float. Any help is appreciated!
def add(a,b):
try:
c = float(a + b)
except ValueError:
print("invalid input")
else:
return c
3 Answers
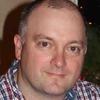
jonlunsford
15,480 PointsKevin: The task may be looking for an integer number instead of a float. Try something like this.... it seemed to work fine for me.
def add(num1, num2):
return num1 + num2

Kevin Lankford
1,983 Pointsthe next step of the task is specifically asking for the arguments to be casted into floats before they are returned. At first I wrote this:
def add(a,b):
return float(a + b)
But that did not work, it said the value (perhaps that is was tested with) was not correct (it had multiple decimals (like 234.45.1). I then tried this:
try: c = float(a + b) except ValueError: print("invalid input")
else:
return c
To send an exception for any invalid input. With this I get the error: 'add` returned the wrong results.

Julian Garcia
18,380 Points1) try converting numbers to float one by one try:
a=float(a)
b=float(b)
then sum both in else block.
2) In except block they ask for return None: Try this:
except ValueError:
return None
Instead:
except ValueError:
print("invalid input")
jonlunsford
15,480 Pointsjonlunsford
15,480 PointsKevin: The task may be looking for an integer number instead of a float. Try something like this.... it seemed to work fine for me.