Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial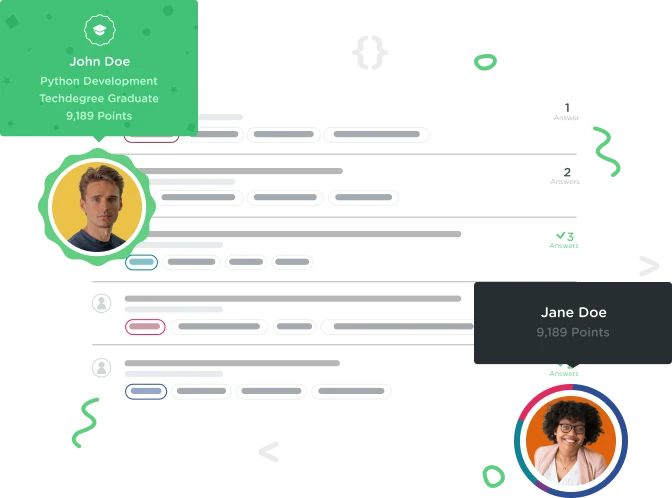

Thomas Dobson
7,511 PointsExtensions: String and Int addition
**Challenge Task 1 of 1
Your task is to extend the String type and add a method that lets you add an integer value to the string. For example: "1".add(value: 2) If the operation is possible, return an integer value. If the string does not contain an integer and you cannot add to it then return nil.**
Can anyone explain to me what I am doing wrong here? This extension makes sense in my head, but the compiler is giving me grief. I cant seem to get around converting the original string to an Int value in order to add the value.
The error I am getting
Playground execution failed: error: WorkSPace.xcplaygroundpage:10:20: error: type of expression is ambiguous without more context
return Int(self) += value
^~~~~~~~~
// Enter your code below
extension String
{
var isInt: Bool
{
return Int(self) != nil
}
}
extension String
{
func add(value: Int) -> Int?
{
if self.isInt
{
return Int(self) += value
}
else
{
return nil
}
}
}
var testString = "2"
testString.add(value: 5)
2 Answers
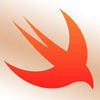
Jeff McDivitt
23,970 PointsHi Thomas -
Here is what I did not sure if you have the extension in there twice in your actual code; that may be causing the problem
extension String {
func add(value: Int) -> Int?{
guard let convertedNumber = Int(self) else{
return nil
}
return convertedNumber + value
}
}
"1".add(value: 3)
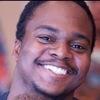
Clinton Johnson
28,714 Pointsextension String {
func add(value: Int) -> Int? {
guard let instanceOfValueIntoStringEqualsItself = Int(self) else {
return nil
}
return instanceOfValueIntoStringEqualsItself + value
}
}
"1".add(value: 2)
Thomas Dobson
7,511 PointsThomas Dobson
7,511 PointsThe first extension is just a "Can this string be converted to an Integer Bool". I called it as the determining factor on my if statement.
Also, way to make use guard; never even crossed my mind.
I was able to make it work with:
But I had to force unwrap Int(self) which I know to be a no no.
I also had a second solution that I think I like better overall ::