Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial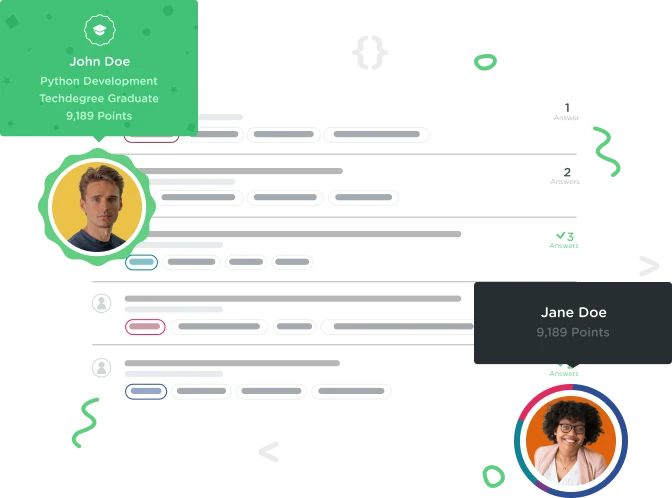
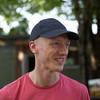
Robert Tyree
13,335 PointsExtra Credit Solution
Wanted to get a little more practice with JavaScript, especially adding objects to a new array, so went ahead and geeked out on the bonus challenge he mentions at the end of video.
Just starting with JavaScript, so I'm sure the code could be more elegant. But sharing in case it's helpful to anyone!
//Asks question until 'quit' or cancel
var query;
while (true) {
query = prompt ('What student? Quit to exit');
if (query === null || query.toLowerCase() === 'quit'){
break;
} else {
searcher(query);
}
}
//Searches for student name and sends matches to msgBuild
function searcher(query){
var currentStudent;
var foundStudent = [];
for (var i = 0; i < students.length; i += 1) {
currentStudent = students[i];
if (query === currentStudent.name){
foundStudent.push(currentStudent);
}
}
if (query !== '' && foundStudent.length === 0){
alert(query + ' is not in our roster!');
}else {
msgBuild(foundStudent);
}
}
//Builds message and sends message to print
function msgBuild(studentMatch) {
var htmlMsg = '';
for (var i = 0; i < studentMatch.length; i += 1) {
htmlMsg += '<h1> Name: ' + studentMatch[i].name + '</h1>';
htmlMsg += '<p> Track: ' + studentMatch[i].track + '</p>';
htmlMsg += '<p> Achievements: ' + studentMatch[i].achievements + '</p>';
htmlMsg += '<p> Points: ' + studentMatch[i].points + '</p>';
}
print(htmlMsg);
if (studentMatch.length > 1){
alert("We've got more than one student named " + studentMatch[0].name + "!");
}
}
//Prints message by inserting into HTML
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsYou'd probably want to 'normalise' the query by converting both it and the names you're checking it against to either all lowercase or uppercase, that way it won't matter if the user doesn't capitalise the name correctly.
As an extension of that, you could make use of the indexOf method for strings so you can return partial matches (e.g. 'rob' would return your name).
Note that if you were doing this, you'd probably want to move the alert for multiple results back to your searcher
function and change it to indicate that there are multiple students matching the user's query, not the particular name.
Otherwise, good use of functions to separate the code! :)
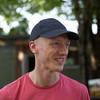
Robert Tyree
13,335 PointsThanks Iain! Really appreciate the pointers.
Dashmiho Dosadku
Courses Plus Student 417 PointsDashmiho Dosadku
Courses Plus Student 417 PointsWhy are you complicating things? The extra solution is just adding "+" symbol to function print