Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial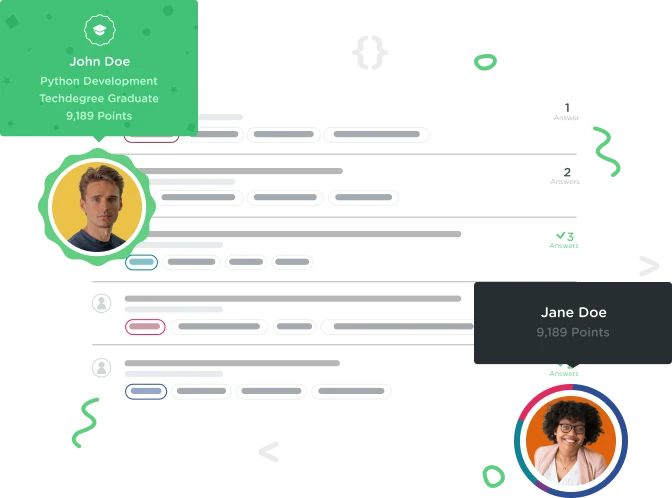

Jake Robinson
32,210 PointsFailure: unable to find link "New todo_list"
Stuck here! any help would be greatly appreciated. I thought it was a simple fix but still having an issue with it. Thanks!!
Failures:
1) Creating todo lists redirects to the todo list index page on success
Failure/Error: click_link "New todo_list"
Capybara::ElementNotFound:
Unable to find link "New todo_list"
# ./spec/features/todo_lists/create_spec.rb:6:in `block (2 levels) in <top (required)>'
.rspec
--color
todo_lists_controller.rb
class TodoListsController < ApplicationController
before_action :set_todo_list, only: [:show, :edit, :update, :destroy]
# GET /todo_lists
# GET /todo_lists.json
def index
@todo_lists = TodoList.all
end
# GET /todo_lists/1
# GET /todo_lists/1.json
def show
end
# GET /todo_lists/new
def new
@todo_list = TodoList.new
end
# GET /todo_lists/1/edit
def edit
end
# POST /todo_lists
# POST /todo_lists.json
def create
@todo_list = TodoList.new(todo_list_params)
respond_to do |format|
if @todo_list.save
format.html { redirect_to @todo_list, notice: 'Todo list was successfully created.' }
format.json { render :show, status: :created, location: @todo_list }
else
format.html { render :new }
format.json { render json: @todo_list.errors, status: :unprocessable_entity }
end
end
end
# PATCH/PUT /todo_lists/1
# PATCH/PUT /todo_lists/1.json
def update
respond_to do |format|
if @todo_list.update(todo_list_params)
format.html { redirect_to @todo_list, notice: 'Todo list was successfully updated.' }
format.json { render :show, status: :ok, location: @todo_list }
else
format.html { render :edit }
format.json { render json: @todo_list.errors, status: :unprocessable_entity }
end
end
end
# DELETE /todo_lists/1
# DELETE /todo_lists/1.json
def destroy
@todo_list.destroy
respond_to do |format|
format.html { redirect_to todo_lists_url, notice: 'Todo list was successfully destroyed.' }
format.json { head :no_content }
end
end
private
# Use callbacks to share common setup or constraints between actions.
def set_todo_list
@todo_list = TodoList.find(params[:id])
end
# Never trust parameters from the scary internet, only allow the white list through.
def todo_list_params
params.require(:todo_list).permit(:title, :description)
end
end
rake routes
Prefix Verb URI Pattern Controller#Action
todo_lists GET /todo_lists(.:format) todo_lists#index
POST /todo_lists(.:format) todo_lists#create
new_todo_list GET /todo_lists/new(.:format) todo_lists#new
edit_todo_list GET /todo_lists/:id/edit(.:format) todo_lists#edit
todo_list GET /todo_lists/:id(.:format) todo_lists#show
PATCH /todo_lists/:id(.:format) todo_lists#update
PUT /todo_lists/:id(.:format) todo_lists#update
DELETE /todo_lists/:id(.:format) todo_lists#destroy

Jake Robinson
32,210 PointsMaciej Czuchnowski does this help?
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsPlease publish your rspec file, view of this page, controller and result of rake routes command. Use Markdown to make it more readable.