Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial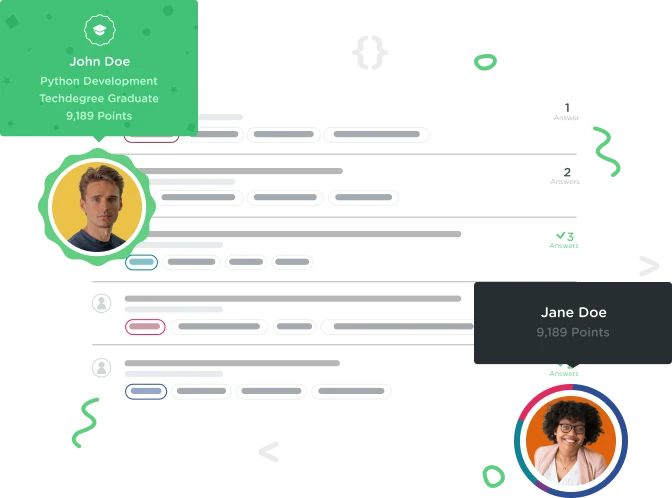

Scott Bradshaw
1,331 PointsFailure/Error: expect(page).to have_content("Milk") expected to find text "Milk" in ""
I have checked everything multiple times and still getting this error. Can anyone please help?
Adding todo lists is successful with valid content
Failure/Error: expect(page).to have_content("Milk")
expected to find text "Milk" in ""
# ./spec/features/todo_items/create_spec.rb:20:in `block (3 levels) in <top (required)>'
# ./spec/features/todo_items/create_spec.rb:19:in `block (2 levels) in <top (required)>'
Here is my code:
require 'spec_helper'
describe "Adding todo lists" do
let!(:todo_list) { TodoList.create(title: "Grocery list", description: "Groceries") }
def visit_todo_list(list)
visit "/todo_lists"
within "#todo_list_#{list.id}" do
click_link "List Items"
end
end
it "is successful with valid content" do
visit_todo_list(todo_list)
click_link "New Todo Item"
fill_in "Content", with: "Milk"
click_button "Save"
expect(page).to have_content("Added todo list item")
within("ul.todo_items") do
expect(page).to have_content("Milk")
end
end
end

Scott Bradshaw
1,331 PointsSorry for not posting the code for the view. Here it is:
<h1><%= @todo_list.title %></h1>
<ul class="todo_items">
<% @todo_list.todo_items.each do |todo_item| %>
<li><%= todo_item.content %></li>
<% end %>
</ul>
<p>
<%= link_to "New Todo Item", new_todo_list_todo_item_path %>
</p>
When I test it locally I get back a dot for the UL but nothing is saved beside it. It looks like this:
Added todo list item.
Grocery List
.
New Todo Item
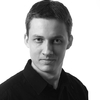
Maciej Czuchnowski
36,441 PointsOK, please post the controller for todo items as well.

Scott Bradshaw
1,331 Pointscontroller:
class TodoItemsController < ApplicationController
def index
@todo_list = TodoList.find(params[:todo_list_id])
end
def new
@todo_list = TodoList.find(params[:todo_list_id])
@todo_item = @todo_list.todo_items.new
end
def create
@todo_list = TodoList.find(params[:todo_list_id])
@todo_item = @todo_list.todo_items.new(todo_item_params)
if @todo_item.save
flash[:success] = "Added todo list item."
redirect_to todo_list_todo_items_path
else
flash[:error] = "There was a problem adding that todo list item."
render action: :new
end
end
private
def todo_item_params
params[:todo_item].permit[:content]
end
end
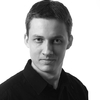
Maciej Czuchnowski
36,441 PointsOK, I don't see anything obviously wrong. I can try running your code locally if you post it on github and link it here. That's the last resort :)

Scott Bradshaw
1,331 PointsMaciej, thanks for helping me with this. Here is my github link: https://github.com/rscottbradshaw/Todo-List
2 Answers
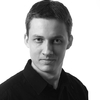
Maciej Czuchnowski
36,441 PointsHere's your problem, todo_items controller, line 25. Your permit has [] brackets and should have () brackets, like this:
params[:todo_item].permit(:content)

Scott Bradshaw
1,331 PointsYou are amazing!! Thank you so much for taking the time to help me with this. I have spent most of my day trying to figure that out.
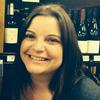
Sara Greer
16,032 PointsIn case anyone else has this problem and their todo_items_controller.rb line 25 already looked like this:
params[:todo_item].permit(:content)
Check line 13:
@todo_item = @todo_list.todo_items.new(todo_item_params)
My code was missing (todo_item_params)
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsYou have to show us more code. For example, the view. Also, please do the test manually and tell me what you see when you follow the steps from the spec. Does it show any Milk?