Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial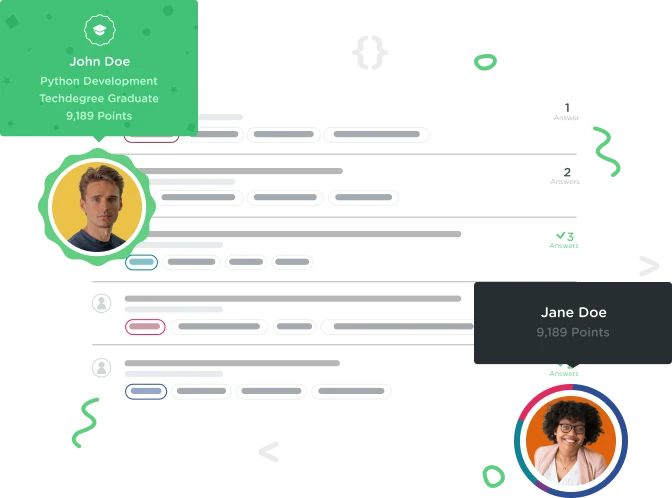
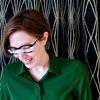
Vanessa Van Gilder
7,034 PointsFeedback appreciated for my teachers code challenge. Passed but probably overly complicated.
So this took me a while (especially most_courses) but I did it...but looking at other people's code on here I think I maybe I made it overly complicated? I have a tendency to do that so I would really appreciate any feedback!
def num_teachers(teach_dict):
return len(teach_dict)
def num_courses(teach_dict):
course_num = 0
for teacher in teach_dict:
for course in teach_dict[teacher]:
course_num += 1
return course_num
def courses(teach_dict):
class_list = []
for teacher in teach_dict:
for course in teach_dict[teacher]:
class_list.append(course)
return class_list
def most_courses(teach_dict):
most = 0
for teacher,courses in teach_dict.items():
if len(courses) > most:
most = len(courses)
name = teacher
return name
def stats(teacher_dict):
list_of_lists = []
for teacher,courses in teach_dict.items():
single_list = [teacher, len(courses)]
list_of_lists.append(single_list)
return list_of_lists
2 Answers

andren
28,558 PointsI think most of your code is quite good, your most_courses
code is pretty much identical to how I would have coded it. There are a myriad of ways of solving most issues when it comes to programming, some which will be shorter than others, but making the solution as short as possible is not always better.
Shorter code is not guaranteed to be faster than a longer variant, and it can often be harder to read and understand it in the future. So you should not feel bad just because your solution is slightly longer than some of the other answers on this forum. As long as the code is readable and doesn't do a lot of unnecessary stuff you are fine.
That said if I had to come up with some complaints about your code it would mostly be the nested loop that you use in the num_courses
and courses
function. Looping is a relatively expensive operation that doesn't scale well (the more items the longer it takes) and this becomes even more true when you place a loop within another loop.
It can also easily become hard to keep track of what is happening when you nest loops under each other. Because of that you should generally try to stay away from nested loops whenever you can. Here is an example of the two functions that are written without a nested loop:
def num_courses(teach_dict):
course_num = 0
for courses in teach_dict.values(): # Only loop through the values
course_num += len(courses) # Use the `len` function to get the number of courses
return course_num
def courses(teach_dict):
class_list = []
for courses in teach_dict.values(): # Only loop through the values
class_list += courses # Append the courses array directly to the class_list array
return class_list
That is how I would have written those functions. Just as a note though I'm not saying you should never nest loops, there are certainly instances where doing so is the only logical solution, but you should always look at the code and make sure that a nested loop really is the most efficient solution you can come up with.
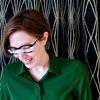
Vanessa Van Gilder
7,034 PointsThanks andren! I really appreciate you taking the time to break everything down!

andren
28,558 PointsNo problem, it can be difficult when you are first starting out with coding, there's a lot of new concepts to learn and lots of ways to do stuff so it is normal to be a bit overwhelmed.
If you have any other programming questions then feel free to post them in a reply, I try to answer anything I can.
Good luck with the rest of your coding.
Vanessa Van Gilder
7,034 PointsVanessa Van Gilder
7,034 PointsThanks andren! That makes a lot more sense. I guess it's something I will get better at with time and experience. I have a question though--could you explain this a little more?
class_list += courses
I know that
class_list.append(courses)
doesn't work in this case because you end up with lists inside of the list.
andren
28,558 Pointsandren
28,558 PointsYeah it definitively is something you'll get better at as you spend more time working on coding and get to see more examples of how others have solved similar problems.
As for that code snippet, when you use
+=
on a list you end up extending it rather than appending to it. Soclass_list += courses
is actually equivalent toclass_list.extend(courses)
rather thanclass_list.append(courses)
.The
extend
method takes a list in as an argument and adds all of the items of that list to the end of the other list, rather than adding the entire list as one item like theappend
method does.