Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial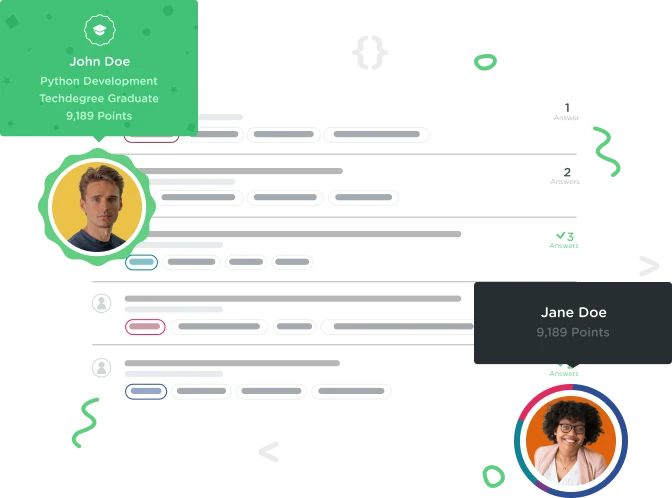
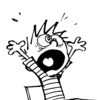
stevenstabile
9,763 PointsFeedback on my quiz.js code
I'm pretty new to JavaScript. Any feedback on my quiz game would be greatly appreciated. It looks very organized to me but it also took more lines of code than the teacher's example so I'd like to know anybody's thoughts...
var score = 0;
var correct1 = false;
var correct2 = false;
var correct3 = false;
var correct4 = false;
var correct5 = false;
var question1 = prompt("What is the capital of NJ?");
var question2 = prompt("What is the capital of NY?");
var question3 = prompt("What is worth one cent?");
var question4 = prompt("In what state is Charleston?");
var question5 = prompt("What color is the sky?");
var answer1 = "trenton";
var answer2 = "albany";
var answer3 = "penny";
var answer4 = "sc";
var answer5 = "blue";
if(question1.toLowerCase() === answer1) {
correct1 = true;
score +=1;
}
if(question2.toLowerCase() === answer2) {
correct2 = true;
score +=1;
}
if(question3.toLowerCase() === answer3) {
correct3 = true;
score +=1;
}
if(question4.toLowerCase() === answer4) {
correct4 = true;
score +=1;
}
if(question5.toLowerCase() === answer5) {
correct5 = true;
score +=1;
}
document.write("<p>You answered " + score + " questions correctly.</p>");
if(score === 0) {
document.write("<p>I'm sorry you didn't get a medal this time.</p>");
}
if(score === 5) {
document.write("<p>Congrats, you win a gold medal!!!</p>");
}
if(score === 3 || score === 4) {
document.write("<p>Congrats, you win a silver medal!</p>");
}
if(score === 1 || score === 2) {
document.write("<p>You win a bronze medal.</p>");
}
```javascript
2 Answers

LaVaughn Haynes
12,397 PointsLooks good to me. The only suggestions I would make for you at this point would be to get rid of the correctX variables since you don't use them. Also, use else if for your score output instead of multiple if's. This is because if one test passes there is no need to perform the other tests. Right now, even if the score is 0, it will still run the other 3 tests even though there is no way they will pass.
var score = 0;
var question1 = prompt("What is the capital of NJ?");
var question2 = prompt("What is the capital of NY?");
var question3 = prompt("What is worth one cent?");
var question4 = prompt("In what state is Charleston?");
var question5 = prompt("What color is the sky?");
var answer1 = "trenton";
var answer2 = "albany";
var answer3 = "penny";
var answer4 = "sc";
var answer5 = "blue";
if(question1.toLowerCase() === answer1) {
score +=1;
}
if(question2.toLowerCase() === answer2) {
score +=1;
}
if(question3.toLowerCase() === answer3) {
score +=1;
}
if(question4.toLowerCase() === answer4) {
score +=1;
}
if(question5.toLowerCase() === answer5) {
score +=1;
}
document.write("<p>You answered " + score + " questions correctly.</p>");
if(score === 5) {
document.write("<p>Congrats, you win a gold medal!!!</p>");
}else if(score === 4 || score === 3) {
document.write("<p>Congrats, you win a silver medal!</p>");
}else if(score === 2 || score === 1) {
document.write("<p>You win a bronze medal.</p>");
}else{
document.write("<p>I'm sorry you didn't get a medal this time.</p>");
}
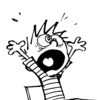
stevenstabile
9,763 Points@LaVaughn Haynes Thanks for the advice! I updated my code and got rid of all the extraneous variables. I also added the else if's like you mentioned. How does this look??
var score = 0;
var totalQuestions = 5;
var question1 = prompt("What is the capital of NJ?");
var question2 = prompt("What is the capital of NY?");
var question3 = prompt("What is worth one cent?");
var question4 = prompt("In what state is Charleston?");
var question5 = prompt("What color is the sky?");
var answer1 = "trenton";
var answer2 = "albany";
var answer3 = "penny";
var answer4 = "sc";
var answer5 = "blue";
if(question1.toLowerCase() === answer1) {
score +=1;
}
if(question2.toLowerCase() === answer2) {
score +=1;
}
if(question3.toLowerCase() === answer3) {
score +=1;
}
if(question4.toLowerCase() === answer4) {
score +=1;
}
if(question5.toLowerCase() === answer5) {
score +=1;
}
document.write("<p>You answered " + score + " out of " + totalQuestions + " questions correctly.</p>");
if(score === 5) {
document.write("<p>Congrats, you win a gold medal!!!</p>");
}
else if(score === 3 || score === 4) {
document.write("<p>Congrats, you win a silver medal!</p>");
}
else if(score === 1 || score === 2) {
document.write("<p>You win a bronze medal.</p>");
}
else {
document.write("<p>I'm sorry you didn't get a medal this time.</p>");
}
```javascript