Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial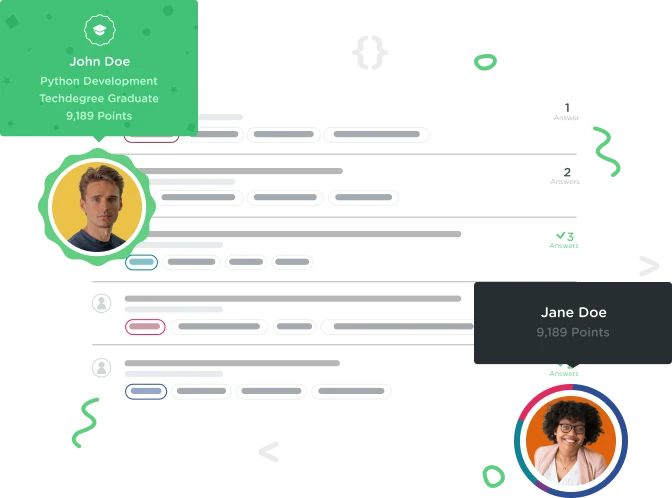
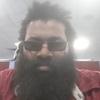
Christopher Sea
3,726 PointsFinal alert showing ALL questions are CORRECT, even if ALL questions are INCORRECT. Not sure why.
Here is my code.
// Alert Program BEGIN.
alert('Let\'s play the Dragon Ball Z Trivia Game!');
// Log Program BEGIN.
console.log('BEGIN Program.');
// Alert Instructions.
alert('There are 5 questions.');
alert('5 CORRECT answers= You get ALL 7 Dragon Balls.');
alert('3-4 CORRECT answers= You get 5 Dragon Balls.');
alert('1-2 CORRECT answers= You only get 2 Dragon Balls.');
alert('0 CORRECT answers= You don\'t get any Dragon Balls.');
// Log Provide Instructions.
console.log('Provide Instructions.');
var score = 0;
// Ask questionOne.
var questionOne = false;
questionOne = prompt('Who was the 1st human to successfully climb to the top of Korin\'s Tower?');
// Log questionOne.
console.log(questionOne);
document.write('<br><br><br><h2>1. Who was the 1st human to successfully climb to the top of Korin\'s Tower?</h2>');
// Test if player is CORRECT, then respond.
if (questionOne.toUpperCase() === 'MASTER ROSHI' || questionOne.toUpperCase() === 'ROSHI') {
score += 1;
document.write('<br><h2><b>' + questionOne + ' is the CORRECT answer!</b></h2>');
console.log('questionOne= CORRECT');
} else {
document.write('<br><h2><b>' + questionOne + ' is the INCORRECT answer.</b></h2>');
console.log('questionOne= INCORRECT');
}
// Ask questionTwo.
var questionTwo = false;
questionTwo = prompt('How many members were in the Ginyu Force?');
// Log questionTwo.
console.log(questionTwo);
document.write('<br><br><br><h2>2. How many members were in the Ginyu Force?</h2>');
// Test if player is CORRECT, then respond.
if (questionTwo === '5' || questionTwo.toUpperCase() === 'FIVE') {
score += 1;
document.write('<br><h2><b>' + questionTwo + ' is the CORRECT answer!</b></h2>');
console.log('questionTwo= CORRECT');
} else {
document.write('<br><h2><b>' + questionTwo + ' is the INCORRECT answer.</b></h2>');
console.log('questionTwo= INCORRECT');
}
// Ask questionThree.
var questionThree = false;
questionThree = prompt('What was the name of Goku\'s father?');
// Log questionThree.
console.log(questionThree);
document.write('<br><br><br><h2>3. What was the name of Goku\'s father?</h2>');
// Test if player is CORRECT, then respond.
if (questionThree.toUpperCase() === 'BARDOK') {
score += 1;
document.write('<br><h2><b>' + questionThree + ' is the CORRECT answer!</b></h2>');
console.log('questionThree= CORRECT');
} else {
document.write('<br><h2><b>' + questionThree + ' is the INCORRECT answer.</b></h2>');
console.log('questionThree= INCORRECT');
}
// Ask questionFour.
var questionFour = false;
questionFour = prompt('What is the title of the 1st Dragon Ball Z Opening Theme Song?');
// Log questionFour.
console.log(questionFour);
document.write('<br><br><br><h2>4. What is the title of the 1st Dragon Ball Z Opening Theme Song?</h2>');
// Test if player is CORRECT, then respond.
if (questionFour.toUpperCase() === 'CHA-LA HEAD-CHA-LA' || questionFour.toUpperCase() === 'CHA LA HEAD CHA LA') {
score += 1;
document.write('<br><h2><b>' + questionFour + ' is the CORRECT answer!</b></h2>');
console.log('questionFour= CORRECT');
} else {
document.write('<br><h2><b>' + questionFour + ' is the INCORRECT answer.</b></h2>');
console.log('questionFour= INCORRECT');
}
// Ask questionFive.
var questionFive = false;
questionFive = prompt('What is the name of the writer of the Dragonball manga?');
// Log questionFive.
console.log(questionFive);
document.write('<br><br><br><h2>5. What is the name of the writer of the Dragonball manga?</h2>');
// Test if player is CORRECT, then respond.
if (questionFive.toUpperCase() === 'AKIRA TORIYAMA') {
score += 1;
document.write('<br><h2><b>' + questionFive + ' is the CORRECT answer!</b></h2>');
console.log('questionFive= CORRECT');
} else {
document.write('<br><h2><b>' + questionFive + ' is the INCORRECT answer.</b></h2>');
console.log('questionFive= INCORRECT');
}
// Player Score
if (score = 5) {
alert('You answered all ' + score + ' questions correctly. \ You get ALL 7 Dragon Balls.');
} else if (score >= 3) {
alert('You answered ' + score + ' questions correctly. \ You get 5 Dragon Balls.');
} else if (score >= 1) {
alert('You only answered ' + score + ' questions correctly. \ You only get 2 Dragon Balls.');
} else {
alert('You didn\'t answer any of the questions correctly. \ You don\'t get any Dragon Balls.');
}
// Alert Program END.
alert('Thanks for playing.');
// Log Program END.
console.log('END Program.');
2 Answers

Tijo Thomas
9,737 PointsHi Christopher,
The problem is how you initialize(assign a value) all of your question variables. All of your question variables follow the format:
var questionOne = false; // You initialized the variable to a boolean(true or false) data type.
On the next line you then assign that variable to the input of a window prompt:
questionOne = prompt('Who was the 1st human to successfully climb to the top of Korin\'s Tower?');
The problem is that you have initialized the questionOne variable to a boolean data type(true or false) and then you try to use the prompt() method to assign the user input to the questionOne variable. The prompt() method returns a value with a string data type. You are trying to assign a string value to a variable that has been initialized as a boolean data type, which will not work. You can change the data type of a variable, but that's beyond where you are in your learning at this point.
Instead, what you should do is initialize the questionOne variable as the prompt.
var questionOne = prompt('Who was the 1st human to successfully climb to the top of Korin\'s Tower?');
This way, questionOne will contain a string, and as a result the conditionals in your if statements should evaluate correctly. The more you learn the more experience you'll have with data types so keep learning.
Just a note: you are logging each question to the console, but aren't doing anything it with in the console. If you have code that doesn't have a purpose, remove it.
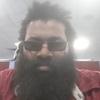
Christopher Sea
3,726 PointsAfter looking at The Conditional Challenge Solution, I saw something else I was doing wrong.
This:
if (score = 5)
should have been this:
if (score === 5)
When I changed to === the page began working properly.
Christopher Sea
3,726 PointsChristopher Sea
3,726 PointsThanks for the response. I removed "questionXXXXX = false;" from each question it's still doing the same thing. It will show that each answer is incorrect on the page, but the final alert still shows that all of the questions were answered correctly. I tried it in a different browser, and it's not working there either.