Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial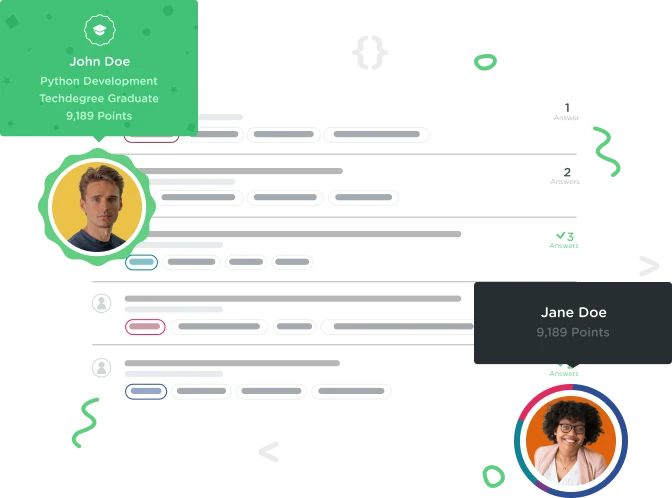
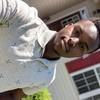
Jamaru Rodgers
3,046 PointsFinding teacher w/ max num of course
Maximum headache achieved! lol Please help! I've been doing this code for about 2 hours by myself because I wanted to figure this out, but I've tried everything I know to do..
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(arg):
count = len(arg)
return count
def num_courses(arg):
total = 0
for teacher in arg.values():
for course in teacher:
total += 1
return total
def courses(arg):
course_list = []
for teacher in arg.values():
for course in teacher:
course_list.append(course)
return course_list
def most_courses(arg):
max_count = 0
correct_list = []
for teacher in arg.keys():
if len(teacher) > max_count:
max_count = len(teacher)
for teacher in arg.keys():
if len(teacher) == max_count:
correct_list.append(teacher)
return correct_list
1 Answer

Pete P
7,613 Pointsif len(teacher) > max_count:
This piece of code above is checking the length of the key, rather than the length of the list that the key corresponds to. To check the length of the list of courses it should look like this:
if len(arg[teacher]) > max_count:
Also, make sure you are returning just the name of the teacher with the most courses, not a list with name(s).
Your code could be changed to look something like:
def most_courses(arg):
max_count = 0
correct_teacher = '' # Note: this is not a list
for teacher in arg.keys():
if len(arg[teacher]) > max_count:
# update max_count
# update correct_teacher
return correct_teacher
Hopefully these hints help. If not, write back and let me know where you're getting stuck.
Jamaru Rodgers
3,046 PointsJamaru Rodgers
3,046 PointsThe only error it's throwing is that I'm not getting all the teacher names. And yes, I know I used arg.keys() instead of .values(), but it was saying that same error before when I was.