Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial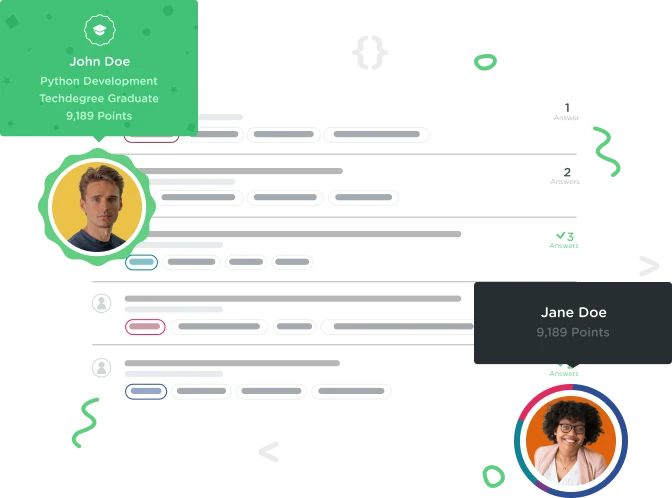

fahad lashari
7,693 PointsFor any one that's confused, here is my solution. If any one as any improvments, I would greatly appreciate the feedback
def function():
list_items = []
item = ""
#1*Run the script to start using it
while item!="DONE":
item = input('Add a task to be done: \n')
#2*Enter the word DONE in all caps to quit the programme
if item=="DONE":
#4*One I quit, I want the app to show me everything that's on my list
print(list_items)
break
else:
#3*put new things into the list, one at a time
list_items.append(item)
function()
3 Answers

Stephan L
17,821 PointsLooks good, I also thought of using the while loop to monitor for the "DONE" condition. One reason I wouldn't do it - and someone, anyone, please jump in and tell me if I'm way off base here, but having the script continuously running a while loop the entire time the program is running isn't the most efficient use of memory. Really, what you're looking for is a particular "flag" to let the program know to do something else. You did that too, with your if statement, but you can actually use just the if statement without the loop running constantly.
What I ended up doing was encapsulating everything into a function, add_item(). It asks for input and places that input into a variable, input_item. If input_item == "DONE", the function prints the list and exits the function. Else, it appends input_item to a list, items, and then iterates through items, printing each item. It then runs the function again (I wondered if in Python, like in Javascript, functions can run themselves, and luckily they can!)
So here's my code, basically mine behaves the same as yours, but it doesn't need a while loop to run:
items = []
def add_item():
input_item = input("Enter an item ")
if "DONE" in input_item:
for item in items:
print(item)
print("Thanks! List complete")
else:
items.append(input_item)
add_item()
add_item()

Nevo Goldman
1,727 Pointsitem_list = [] while(True): item = input("new item to the list ") if item == "DONE": break item_list.append(item) print(item_list)
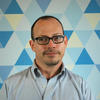
Robert Hernandez
9,357 PointsGood work!
Feedback, as requested:
- Try to give clear, descriptive names to all functions/methods, even ones coded for exercises, i.e., add_to_grocery_list() vs function()
- Consider using the .upper() string method instead of hardcoding "DONE", as this would allow users to enter text in any case without 'breaking' the program
- It would help the user if you printed some instructions
I hope this was helpful. Keep it up!
fahad lashari
7,693 Pointsfahad lashari
7,693 PointsWow that's really smart. I personally hate having to use loops for everything but it seems though that the use of loops is highly encouraged and that knowledge is repeatidly reinforced everytime we build a programme. Good to see you took a different approach. I will most definitely try to take this approach in the future programmes I code. Constantly using loops for everything to me seems like a broken approach. A programme should run when needed and as needed. I just wasn't sure how that could be done without a loop. But I can see that you've called the 'add_item' function twice. Once to initialise the whole programme and once more to continue running unless the user quits. Clever.
Thanks for the help
Kind regards,
Fahad
fahad lashari
7,693 Pointsfahad lashari
7,693 PointsHi Stephen,
Just wanted to say thanks for introducing me to this other side of achieving loop type functionality without a loop. I just created this programme without a while loop and only one for loop for printing.
Also I have not yet implemented any functionality that stops the user from type incorrect Values e.g. if you type in a String where the programme asks for a index value, the programme will just throw an error, I will be adding this later. Also I have not added the functionality to added multiple items at once as I forgot, you can add it in as you wish.
EDIT: just added the functionality of adding more than one item with a loop. Still can't figure out how to implement a 'strip()' function without using a loop. If you know, do let me know.
Please test it out in your workspaces and let me know what you think
Kind regards
Fahad