Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial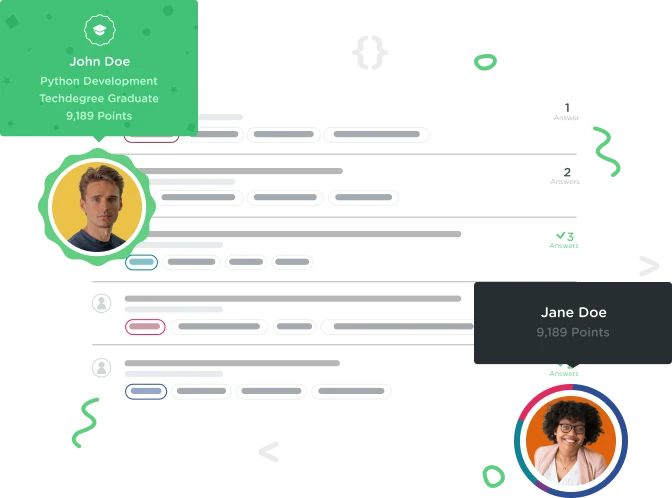

Robert Kooyman
4,927 Pointsfor loop stops at 10
Why is my for loop not repeated 100 times?
var html = ''; var red; var green; var blue; var rgbColor; function getRandomColor = Math.floor(Math.random() * 256 );
for (i=1; i<=100; i+=1) { red, green, blue = getRandomColor; rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')'; html += '<div style="background-color:' + rgbColor + '"></div>'; }
document.write(html);
1 Answer
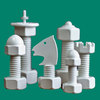
Steven Parker
241,955 Points
Your loop repeats 100 times. But you have some other issues:
//------------------------------------- instead of this: ----------------------------------------
function getRandomColor = Math.floor(Math.random() * 256 ); // not a valid function definition!
//------------------------------------- do one of THESE: ----------------------------------------
function getRandomColor() { return Math.floor(Math.random() * 256); } // but this is
var getRandomColor = function(){ return Math.floor(Math.random() * 256); } // and so is this
I left the name "getRandomColor" but it actually gets a random number. It takes three of these numbers to make up a color. But then when it gets used:
//------------------------------------- instead of this: ----------------------------------------
// this does not assign red or green
red, green, blue = getRandomColor; // and this doesn't call the function
//----------------------------------------- do THIS: --------------------------------------------
red = getRandomColor(); // this calls the function and assigns the result
green = getRandomColor(); // each color gets a different number
blue = getRandomColor();
And finally, to make sure the div's are visible:
//------------------------------------- instead of this: ----------------------------------------
html += '<div style="background-color:' + rgbColor + '"></div>';
//----------------------------------------- do THIS: --------------------------------------------
// this shows the count and gives the div some height so it can be seen
html += '<div style="background-color:' + rgbColor + '">' + i + '</div>';
Siddhant Mehta
Courses Plus Student 478 PointsSiddhant Mehta
Courses Plus Student 478 PointsHey Robert, I think you should call getRandomColor function seperately on each var red,green & blue. Check out my code :-