Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial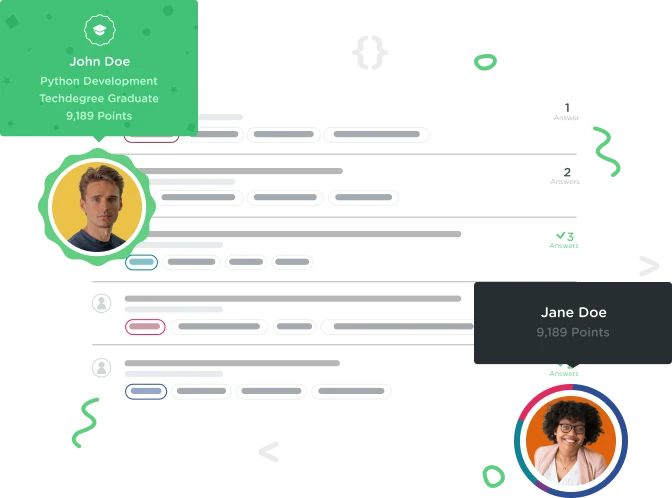

Rachel Hutchings
4,293 PointsFor loop with Array Challenge
I am having a tough time really understanding/completing this Challenge. The task was to "Use a for or while loop to iterate through the values in the temperatures array from the first item -- 100 -- to the last -- 10. Inside the loop, log the current array value to the console." For loops, easy. console.log, easy. Though trying to get the right thing to print in the log is not as easy. I keep getting an error that "my code took too long to load", which isn't very helpful. If I take out the for loop completely it loads fine. Not really sure what I'm completely messing up here... I tried to add a function around the for loop like I did in a workspace following along with the video but that didn't seem needed. ?
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i < temperatures.length; i -= 10) {
console.log(temperatures());
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
4 Answers
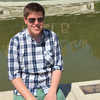
Ben Schroeder
22,818 PointsInstead of this...
console.log(temperatures());
... you want to be logging this:
console.log(temperatures[i]);
Should also mention, you don't want to decrement i by 10 each time you run through the loop. In this instance, the variable i refers to the index (or position) of the array item, not the item itself. So you don't want to pay attention to the values in the array, but the index of those values.
temperatures[0] = 100
temperatures[1] = 90
temperatures[2] = 99
etc.
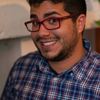
Salmen Bejaoui
8,017 Pointsvar temperatures = [100,90,99,80,70,65,30,10];
for(var i = 0; i < temperatures.length; i++) {
console.log(temperatures[i]);
}
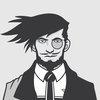
edinjusupovic
5,664 PointsYou were really close. I'm going to break down the code so it makes it easier to understand.
var temperatures = [100,90,99,80,70,65,30,10];
Here we create the variable temperatures with an array consisting of the numbers 100,90,99,80,70,65,30,10. As you likely know through your learning, arrays have a value. We can use that value to call or use a specific array. Additionally, arrays always start at the "index value" of zero. This means that the first value is always zero.
For example, say if we wish to call the number 100 from the temperatures array. We do the following;
var temperatures = [100,90,99,80,70,65,30,10];
console.log( temperatures[0] );
This would now return 100.
To understand this challenge we must understand the inner workings of the for loop.
for ( 'initial variable declared' ; 'what we test against'; 'what we add if tested against is true')
for (var i = 0;
We associate the variable "i" with the number zero.
for (var i = 0; i < temperatures.length;
We tell the for loop that if the "i" variable is LESS THAN (with the "<" operator) that we want to move onto the next instruction in the sequence. The "temperatures.length" automatically finds out how many values there are in the temperatures array - in a sense, it automatically counts that there are 8 numbers in the temperatures array and uses that.
for (var i = 0; i < temperatures.length; i += 1)
This will then tell the loop to add 1 to the i variable and execute the code below.
for (var i = 0; i < temperatures.length; i += 1) {
console.log(temperatures[i]);
}
The loop keeps going until the variable i is no longer less than what the length of the temperatures array is, in this case 8.
I hope this has been helpful.
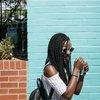
Taqwa R
1,852 PointsI don't understand why we are adding just 1 to each loop. Should we be adding or subtracting 10 from each loop if we want to console to log the numbers by increments of 10?

Jaspal Singh
13,525 Pointshi
after doing the coding as you mentioned above still it is showing the following message
There was an error with your code: TypeError: 'undefined' is not an object (evaluating 'console.log._args[i]')
pls help
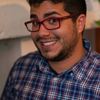
Salmen Bejaoui
8,017 Pointsconsole.log is a function. So you have to open the parenthese console.log(_args[i]). From your error, seems you put a dot after console.log.
Marius Unknown
12,925 PointsMarius Unknown
12,925 PointsA third 'i' in for loop should be incremented by 1 so you should write not ' i -= 10 ', but 'i += 1' or just 'i++'. Also temperatures in console.log should be written 'temperatures[i]'.