Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial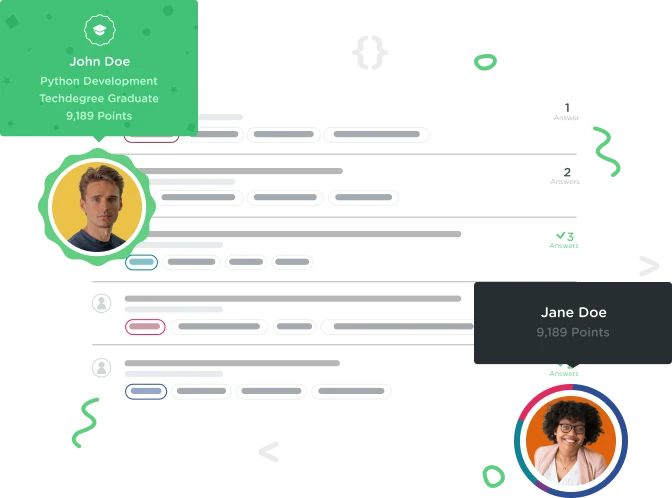

Christopher Roy
Courses Plus Student 1,325 Pointsfor loops with temps
When the variables in an array do not change by a fixed numerical value (i.e. 100 -> 90 = -10, but 90 -> 99 = +9) how do you tell the counter in the for loop what to change by after the loop runs?
Additionally, I am getting an error message saying that it can not evaluate the console.log command.
Any help would be greatly appreciated, thank you!
var temperatures = [100,90,99,80,70,65,30,10];
for (i = 100; i < temperatures.list; i += i) {
console.log( temperatures[ i ] );
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
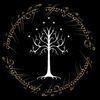
jacinator
11,936 PointsThis code will pass the challenge. i
is tracking the index in the list, not the value.
var temperatures = [100,90,99,80,70,65,30,10];
for (i = 0; i < temperatures.length; i++) {
console.log( temperatures[ i ] );
};

Bryan Peters
11,996 PointsKeep in mind that "i" in your code represents the position index within the array. So each time you should just increment it by 1 to visit every item in the array. if you increment it by 2 you would be skipping every-other element in the array. Your code has it starting at 100 and incrementing by i, so first it visits temperatures[100] which doesnt exist (there are only 8 items in the array, and you are attempting to get the item in the 100th position, hence the error.
You are also using temperatures.list instead of temperatures.length
So instead, start at position 0 and increment by 1, until you reach the length of the array.
for (i = 0; i < temperatures.length; i += 1) {
console.log( temperatures[i] );
}

Jason Anello
Courses Plus Student 94,610 Pointschanged from comment to answer