Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial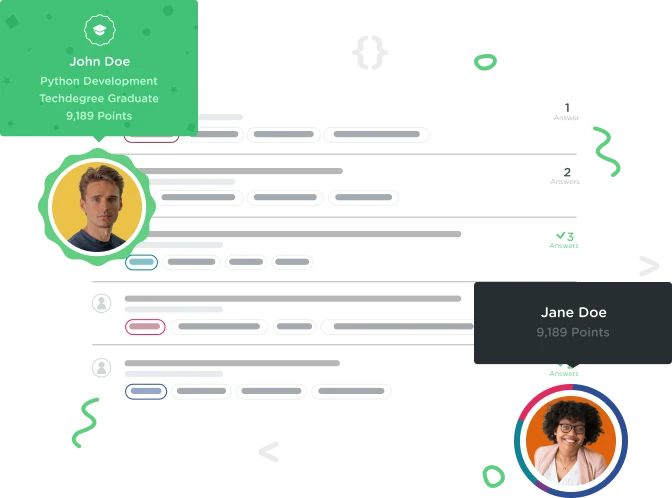
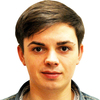
Mažvydas Brikas
6,766 PointsFrog starts moving without any keyboard input
Then I click game play button my frog starts move forward instantly, without any keyboard input. Then I click one of keyboard arrow button, frog changes direction accordingly and moves to that direction everlastingly. What I am doing wrong? How to stop frog’s unnecessary everlasting movement? My code:
using UnityEngine;
using System.Collections;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
private float turningSpeed = 20f;
private Rigidbody playerRigidbody;
// Use this for initialization
void Start () {
// Gather components from Player Game Oblect
playerAnimator = GetComponent<Animator>();
playerRigidbody = GetComponent<Rigidbody> ();
}
// Update is called once per frame
void Update () {
// Gather input from the keyboard
moveHorizontal = Input.GetAxisRaw("Horizontal");
moveVertical = Input.GetAxisRaw("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate () {
// If the player's movement vector does not equal zero...
if (movement != Vector3.zero) {
//...then create a target rotation based on the movement vector...
Quaternion targetRotation = Quaternion.LookRotation(movement, Vector3.up);
//...and craete another rotation that moves from current rotation to the target rotation...
Quaternion newRotation = Quaternion.Lerp (playerRigidbody.rotation, targetRotation, turningSpeed * Time.deltaTime);
//...and change the player's rotation to the new incremental rotation...
playerRigidbody.MoveRotation(newRotation);
// ...then play the jump animation.
playerAnimator.SetFloat ("Speed", 3f);
} else {
// Otherwise, don't play the jump animation.
playerAnimator.SetFloat ("Speed", 3f);
}
}
}
2 Answers
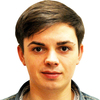
Mažvydas Brikas
6,766 PointsThanks for your help Larry Passantino! My mistake was in that last line, then I changed to (“Speed”, 0f) everything works fine :)
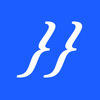
Alan Mattanó
Courses Plus Student 12,188 PointsI remember one time I have a similar problem. I was using the keyboard and I forgot that my joystick was attach to the USB and not well calibrated. Since Input.GetAxis...."Horizontal" the joystick was giving input to the game.
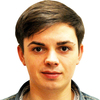
Mažvydas Brikas
6,766 PointsMy first thought was that should be a keyboard/mouse problem or with windows drivers, but like almost always I found out I left simple stupid mistake in my code :)
Larry Passantino
2,307 PointsLarry Passantino
2,307 PointsI believe the problem may be with your last line of code.
playerAnimator.SetFloat ("Speed", 3f);
In the else statement, you should set the speed to 0f and that should take care of your problem.