Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial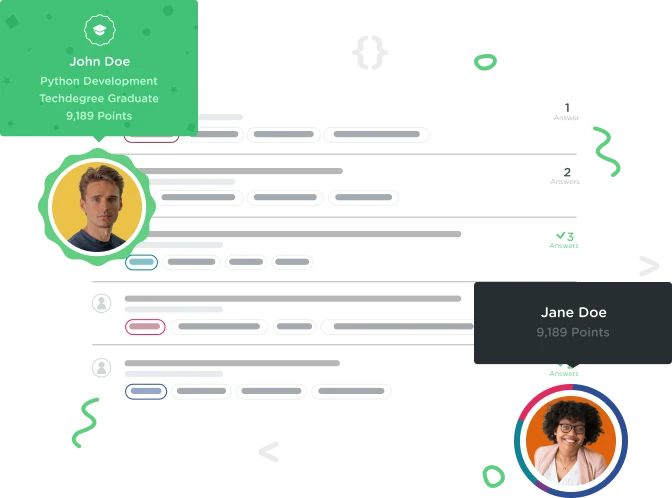

Dhanish Gajjar
20,185 PointsGenerics in Swift 3, Challenge 2
I tried a lot of different ideas. I also created a simple square function. But somehow I ended up with a square function that takes an array of Int and returns an array of Int.
But I think that is not correct. Can anyone guide me here.
In the editor define a function named map with two generic type parameters, T and U. The function takes two arguments - array of type [T], and transformation of type (T) -> U. The function returns an array of type U. The goal of the function is to apply the transformation operation on the array passed in as argument to return a new array containing the values transformed. For example given the array [1,2,3,4,5] as first argument, and a square function as the transformation argument, the result should be [1, 4, 9, 16, 25].
Thank you!
func map<T, U>(array: [T], transformation: (T) -> U) -> U {
}
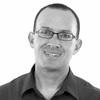
David Papandrew
8,386 PointsHi Dhanish, thanks for posting this. I was having trouble with this challenge too. I was trying to do the same thing as you (function that takes a generic array and returns a squared array). Your post clarified things for me when I realized I just needed to take a single value and return a single value. It should have been clear from the function signature, but I think the challenge could have provided a little more guidance on this matter!
2 Answers

Dhanish Gajjar
20,185 PointsDavid Papandrew I am glad it helped and you're right, it is a bit confusing, because the challenge explicitly mentions it accepts an array of type [T] , transformation of (T) -> U and the entire function returns an array of type U.
We immediately think that U represents an array, which means transformation also accepts an Int and returns an array. I went back and forth and it took time, but it compiled in the end.
I still don't know if this was the right thing to do, I am waiting on others to give their opinion here :)
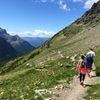
Stephen Wall
Courses Plus Student 27,294 PointsLooks like you already have a working answer but I found a cool little trick for iterating through the [T] array and appending to the [U] array.
func map<T, U>(array: [T], transformation: (T) -> U) -> [U] {
let results: [U] = []
array.forEach { item in
results.append(transformation(item))
}
return results
}
Dhanish Gajjar
20,185 PointsDhanish Gajjar
20,185 PointsFigured out! .... Phew!