Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial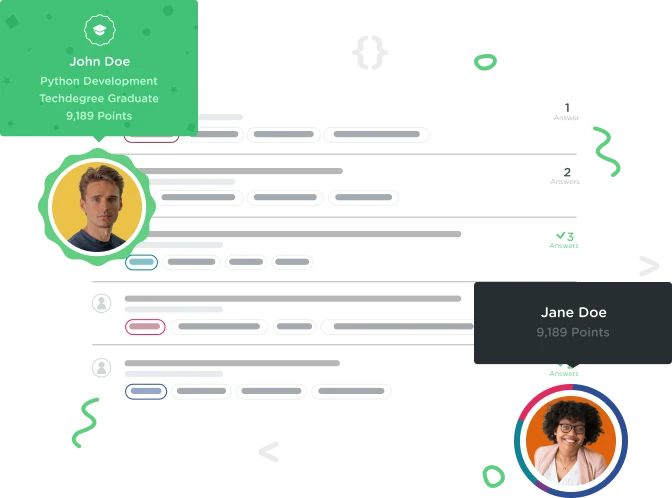

Tobias G
2,455 PointsGetting "[object Object]" when printing question and answer
I tried to convert the code I wrote for the previous quiz project as shown in this tutorial, using objects instead of two-dimensional arrays. The code works for only displaying how many answers you answered correctly, but when printing out the exact questions you answered right or wrong, using objects, you'll get "[object Object]" as the result on every question and answer printed.
How would I do to make the code show the object names?
Here's my code:
var questionList = [
{
question: "What color is the sky?",
answer: "Blue"
},
{
question: "What color is the sea?",
answer: "Blue"
}
];
var rightAnswers = [];
var wrongAnswers = [];
var html = "";
var question;
var answer;
var response;
var correctAnswers = 0;
function print(message) {
document.write(message);
}
for (i = 0; i < questionList.length; i += 1) {
question = questionList[i].question;
answer = questionList[i].answer;
response = prompt(question);
if (response === answer) {
correctAnswers += 1;
rightAnswers.push(questionList[i]);
} else {
correctAnswers += 0;
wrongAnswers.push(questionList[i]);
}
}
print("You got " + correctAnswers + " answers right!")
if ( correctAnswers >= questionList.length) {
print("You earned a gold medal!");
} else if (correctAnswers >= questionList.length / 2) {
print("You earned a silver medal!");
} else {
print("You earned a bronze medal!");
}
if (rightAnswers.length >= 1) {
print("<br />Right answers: " + rightAnswers.join(", "));
}
if (wrongAnswers.length >= 1) {
print("<br />Wrong answers: " + wrongAnswers);}
2 Answers
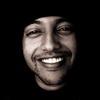
miikis
44,957 PointsHey Tobias,
You probably want to push the answer property of each question-object into rightAnswers and wrongAnswers; right now, you're pushing the entire object and that's what's causing the bug. Also, at the very end, you probably want to join()
wrongAnswers like you did rightAnswers.

Bryan Peters
11,996 Pointswhen you push the answers, push the answer property instead of the entire object, like this:
if (response === answer) {
correctAnswers += 1;
rightAnswers.push(questionList[i].answer);
} else {
correctAnswers += 0;
wrongAnswers.push(questionList[i].answer);
}
also you don't need the "correctAnswers += 0;" for wrong answers