Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial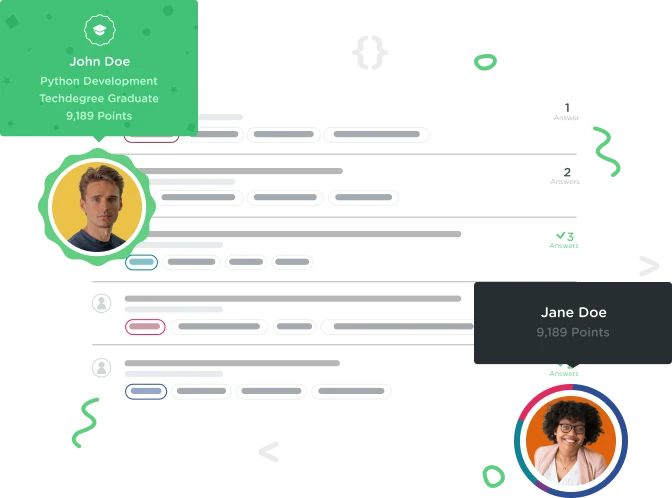
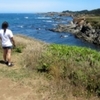
Nancy Melucci
Courses Plus Student 36,143 PointsGridView Picture Array Null Pointer Exception
This is a homework assignment. I can't figure out why I am getting a null pointer exception. By the way, I pretty much copied the code for the MainActivity from the textbook. I already found one typo. I am hoping there is another, because I can't figure out why this won't work.
It's an eight item gridview photo album. The top of the home screen is the grid view of pictures, the bottom is a single picture which is supposed to show when the user clicks on the selected picture.
I checked the XML for incorrect ids. What am I missing? The Java first, then the XML. Thanks.
MainActivity.java
package com.nancy.example.myphotoalbum;
import android.content.Context;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.view.ViewGroup;
import android.widget.AdapterView;
import android.widget.BaseAdapter;
import android.widget.GridView;
import android.widget.ImageView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
Integer[] myPhotos = {R.drawable.brainvessels, R.drawable.flowers, R.drawable.flowerstwo,
R.drawable.hannahandme, R.drawable.jellies, R.drawable.flowersthree, R.drawable.littledragon, R.drawable.pluto};
ImageView pic;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
GridView grid = (GridView) findViewById(R.id.photoGrid);
grid.setAdapter(new ImageAdapter(this));
final ImageView pic = (ImageView) findViewById(R.id.imgLarge);
grid.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent,
View view, int position, long id) {
Toast.makeText(getBaseContext(), "Select Picture " + (position + 1), Toast.LENGTH_LONG).show();
pic.setImageResource(myPhotos[position]);
}
});
}
public class ImageAdapter extends BaseAdapter {
private Context context;
public ImageAdapter(Context c) {
context = c;}
@Override
public int getCount () {
return myPhotos.length;
}
@Override
public Object getItem ( int position){
return null;
}
@Override
public long getItemId ( int position){
return 0;
}
@Override
public View getView ( int position, View convertView, ViewGroup parent){
pic = new ImageView(context);
pic.setImageResource(myPhotos[position]);
pic.setScaleType(ImageView.ScaleType.FIT_XY);
pic.setLayoutParams(new GridView.LayoutParams(250, 230));
return pic;
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:background="@drawable/photogradient"
android:orientation="vertical"
tools:context="com.nancy.example.myphotoalbum.MainActivity">
<GridView
android:layout_width="wrap_content"
android:layout_height="200dp"
android:id="@+id/photoGrid"
android:layout_alignParentTop = "true"
android:layout_alignParentLeft = "true"
android:layout_alignParentStart = "true"
android:numColumns = "auto_fit"
android:columnWidth = "120dp"
android:horizontalSpacing="5dp"
android:verticalSpacing="5dp"/>
<ImageView
android:layout_width="270dp"
android:layout_marginTop="10dp"
android:layout_height="300dp"
android:id="@+id/imgLarge"
android:layout_gravity="center_horizontal"
android:contentDescription="@string/imgLarge"/>
</LinearLayout>