Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial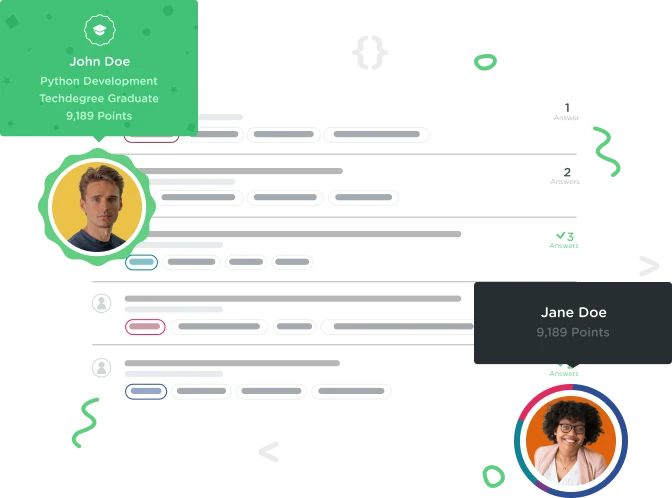
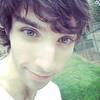
Alphonse Cuccurullo
2,513 PointsGuys what did i do differently thats making the loop not work properly.
def print_hello(number_times)
i = 0
while number_times < 0
puts "hello"
i += 1
end
end
answer = 0
while answer < 5
print "How many times do you want to print 'hello'? Enter a number greater than 5 to exit) "
answer = gets.chomp.to_i
print_hello(answer)
end
its not printing "Hello" the amount of times.
3 Answers
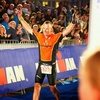
Steve Hunter
57,712 PointsYou're incrementing i
but testing number_times
for being less than 0.
Do you want to test
i < number_times
then stop the loop?
My guess, and I don't have a workspace open, is something like this:
def print_hello(number_times)
i = 0
while i < number_times
puts "hello"
i += 1
end
end
answer = 0
while answer < 5
print "How many times do you want to print 'hello'? Enter a number greater than 5 to exit) "
answer = gets.chomp.to_i
print_hello(answer)
end
Let me know how you get on.
Steve.

Mike Magarino
11,639 PointsHi Alphonse,
Here is the code it appears you are using:
def print_hello(number_times)
i = 0
while number_times < 0
puts "hello"
i += 1
end
end
answer = 0
while answer
print "How many times do you want to print 'hello'? Enter a number greater than 5 to exit) "
answer = gets.chomp.to_i
print_hello(answer)
end
If I have that correctly, then it appears your issue lies within the print_hello method. There you perform a comparison: number_times less-than 0. You can expect the user to always input a number larger than 0 when prompted to do so, therefore the answer variable will usually be a value greater-than 0. The answer variable is then passed to the print_hello method, which checks if number_times less-than 0, which it never will be, therefore the while loop is skipped and puts "hello" is never evaluated.
Try changing the code inside the print_hello method, and that should solve your issue.
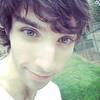
Alphonse Cuccurullo
2,513 PointsWhats the point in making two while loops here if you dont mind me asking?

Mike Magarino
11,639 PointsYou'd have to ask Jason exactly why he decided to use 2 while loops for this lesson, but I believe he was simply using repetition as a learning tool. The same end result can be achieved using different code, and without the need for 2 while loops. But, using it twice helps you understand how they work.
There's another nice lesson in there about one of Ruby's core philosophies: There's more than one way to do the same thing. Feel free to produce the same results with totally different code.
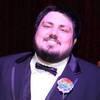
Claudiu Bancos
Front End Web Development Techdegree Graduate 22,468 PointsInstead of
while number_times > 0
write
while i < number_times
That should fix your problem.
Alphonse Cuccurullo
2,513 PointsAlphonse Cuccurullo
2,513 PointsHey man thanks for responding, your code worked tho im not gonna lie im still at a loss. Like the while loop with gets.chomp i get however the while loop withing the print_hello method i dont get. I am havibg trouble seeing how both while loops are synergizing with eachother. Like couldnt i have just put print "hello" in the define method an worked with it through the while loop on he bottom?
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Alphonse,
Apologies for the delay in responding - I was busy last night on other things.
OK, so there's two whle loops. One controls user input; as soon as the user enters something greater than 5, the program terminates. That's a bit of a weird way of doing it, but hey, that's what we've got.
The other while loop is inside the
print_hello
method. That method takes one parameter which is the user input (as long as it wasn't more than 5!); so it is a number from 1 to 4, we hope. That is callednumber_times
inside this method.Inside the method, we create an index or counter, called
i
and set it to zero. We then enter awhile
loop and incrementi
each time the loop is repeated. That's thei += 1
bit. The condition that maintains the loop isi
being less thannumber_times
. As soon ai
becomes equal tonumber_times
, the loop terminates and we return back to the place in code where the method was called from, i.e. right back in the first loop!!Let's walk this through. The program starts and the user is prompted for a number. He enters "3". That string has its whitespace removed (
chomp
) and is converted into an integer(to_i
). We then call theprint_hello
method passing in the user's answer (3) as a parameter. Execution jumps into the method wherenumber_times
is assigned the value 3 which was passed across. We seti
to zero and enter thewhile
loop ifi
is less thannumber_times
. At the moment,i
is zero andnumber_times
is 3 - the loop excutes. Inside the loop, we print "hello" to the screen, add one toi
and repeat. Now,i
is equal to 1 which is still less than three so we print "hello" to the screen, add one toi
and repeat. Now,i
is 2; we do that all again. Now,i
is 3 which is NOT less thannumber_times
- the loop stops, we exit theprint_hello
method and fall back to the first while loop which goes round again and prompts the user for a number.There are much nicer ways of doing this, in reality, but that wouldn't teach you about
while
loops. This is designed to teach you that, not to write pretty code, just yet. For example, if the user entered 7 at the first prompt, theprint_hello
method would be called, "hello" would be printed 7 times, then the loop would exit so this really isn't working nicely at all but it demonstrateswhile
loops well as a concept, not as an implementation.I hope that helps!
Steve.