Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial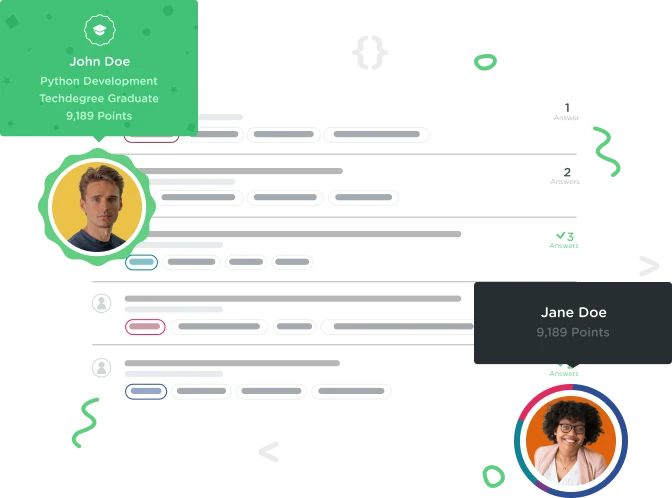

Rahul Raj
2,378 PointsHaving issue with for loop and iteration over an array in Ruby
Hello! I am on the code challenge "The For Loop" for the Ruby Loops section in the Learn Ruby track. The question reads as follows:
Use a for loop to print each item in the animals array to the screen using the puts method. Given code: animals = ["dog", "cat", "horse", "goat"]
My answer:
for item in ["dog", "cat", "horse", "goat"] puts "The item is "#{item}" end
This code ends up with an error saying, "The for loop was not used." I have tried multiple methods to fix the error, such as substituting the array items with just the array name, or changing the "puts" code, but nothing seems to work! Would love some help, thank you!
1 Answer
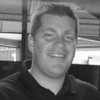
Mike Hickman
19,817 PointsHi Rahul
You have the right idea in your head, it's just not getting executed into code the right way. You're missing the do part that starts the block. Do + end will always go together. Let's walk through it:
- The array animals already holds ["dog", "cat", "horse", "goat"], so when you're setting up your for loop, you don't need to put the entire array there, just animals. You said you tried this already, which was the right way to do it. You were just missing the do keyword at the end of the first line.
- Think about naming as you write code in the future and what will be easy for you and others to remember. In this case, item works, but animal makes more sense.
- Starting the for loop:
for animal in animals do
<-- This is saying for each thing/animal/item in the animals array, let's reference each one as animal as we go through each item so we have an easy way to reference it. - Now that we're in the for loop, we just need to do what they ask.
puts animal
<-- since each item is getting referenced as animal now within our setup, that's a variable and you don't need to use #{interpolation} - end to finish it up
Full thing:
for animal in animals do
puts animal
end
It's one of those things where as you're starting, you'll miss little things like do or end. It's completely normal and happens to everyone. Just keep pushing through and don't get discouraged by little road blocks.
Cheers,
Mike