Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial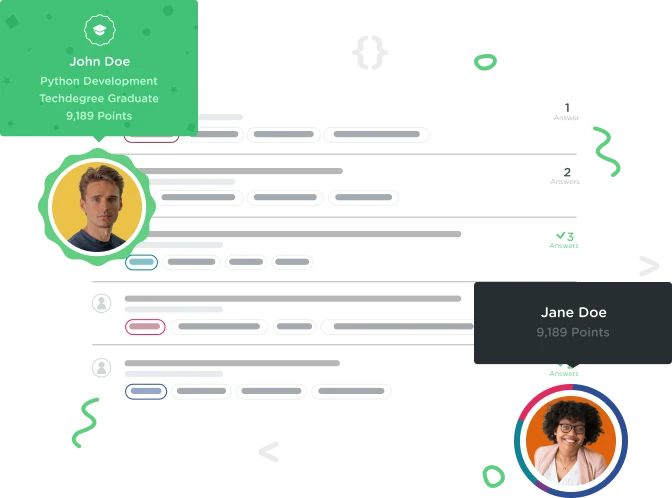

Oli Flemmer
13,394 PointsHaving problems with 'word_count' in python collections
I've spent quite a few hours on trying to debug my code, and even tested it on my own computer. When tested on my laptop it works, but when executed on the challenge page it doesn't. It returns ' Bummer! Hmm, didn't get the expected output. Be sure you're lowercasing the string and splitting on all whitespace'. In the code, I've made sure that I've vividly lowercased the string, and split the string on all whitespace. Even if there's a double space it'll still pick up on it and remove it.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
#lowercasing the string
string = string.lower()
#putting each word in the string into a list
ls = string.split(' ')
#list of valid letters
letter_list = ['a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z']
#empty dictionary
dic = {}
#Selecting each word in the list
for word in ls:
#selecting each letter in that word
for letter in word:
#if that 'letter' is not a letter then delete it
if not letter in letter_list:
lw = list(word)
lw.remove(letter)
word = ''.join(lw)
#if the word isn't in the dictionary then add it
if word not in dic and not word == '':
dic.update({word: 1})
#if it is in the diictionary then add one to it's value
elif word in dic:
dic[word] = dic[word] + 1
return dic
1 Answer
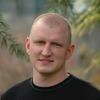
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsHey Oli Flemmer
It looks like you just about got it. Except there are two issues with your code.
- You must split on ALL whitespace. Currently you are only splitting on spaces using
string.split(' ')
Check Python Docs: Split make sure you read the line that talks about:
If sep is not specified or is None
- You are filtering out all characters that arent the letters a thru z. But it didnt ask for you to do that.
#selecting each letter in that word
for letter in word:
#if that 'letter' is not a letter then delete it
if not letter in letter_list:
lw = list(word)
lw.remove(letter)
word = ''.join(lw)
You dont really need this line at all because I believe in the tests for this challenge, other characters are being tested and counted. And if you filter them out it wont return the right result.
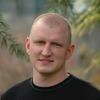
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsThe same applies for you for the String Split you are doing. But in addition to that, the challenge tells you that the function must return
a dictionary. Your function has no return
so it implicitly returns a None
by default. You are instead printing the dictionary but you need to return it. :)
austin bakker
7,105 Pointsaustin bakker
7,105 PointsI am having the same problem , here i my peace of code