Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial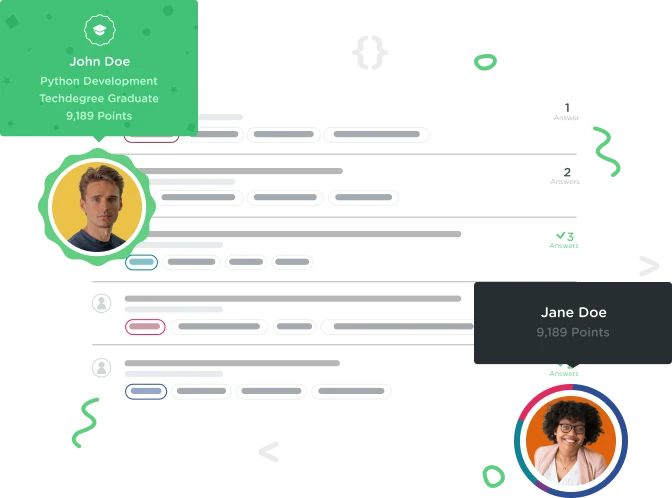

thomas kaplan
1,509 Pointshaving trouble completing this can someone show me the solution?
?
var secret = prompt("What is the secret password?");
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers

Thomas Fildes
22,687 PointsHi Thomas,
Basically you just have to switch round a couple of things in the code. It asks for a do/while loop which will better the code. All that needs to be done is to type do and then cut and paste the code block that currently runs after the while function. Then you can remove the prompt from the variable secret because you will be modifying it inside the do while loop. Here is the code below if you are unsure:
var secret;
do {
secret = prompt("What is the secret password?");
}
while ( secret !== "sesame" )
document.write("You know the secret password. Welcome.");
Happy Coding!

Erik Nuber
20,629 Pointsvar secret = prompt("What is the secret password?");
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
you just need to reword it to fit a do while...
var secret;
do {
secret = prompt("What is the secret password?");
} while ( secret !== "sesame" )
document.write("You know the secret password. Welcome.");
The do while loop means whatever in the loop will happen at least one time. For this reason, we only need to declare the variable secret outside the loop but not actually do the prompt. This is because then the prompt would appear twice before the loop actually tried to see if secret = "seasame"