Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial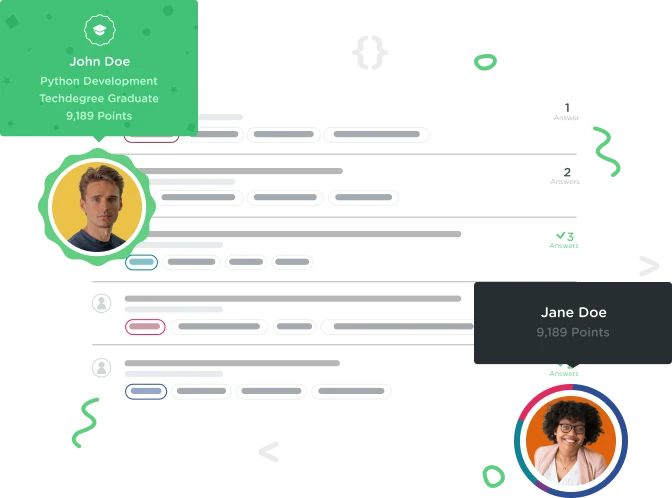

Christopher Kell
3,499 PointsHaving trouble solving this code challenge
Create a new function named just_right that takes a single argument, a string.
If the length of the string is less than five characters, return "Your string is too short".
If the string is longer than five characters, return "Your string is too long".
Otherwise, just return True.
so im not sure if str.len is an actual function.
def just_right(x):
x=str.len(5)
if just_right < x:
return "Your string is too short"
if just_right>x:
return "Your string is too long"
else:
return True
1 Answer
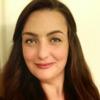
Jennifer Nordell
Treehouse TeacherHi Christopher! You've got a few things going on here. First, you've got two if statements. What you should have are an if statement, an elif statement, and an else statement. Also, you're comparing just_right
to the value of 5. But just_right
is the name of the method you're defining. What you should be comparing is the length of x
to 5. But you've now overwritten the value of x coming into the function. Take a look:
def just_right(x):
if len(x) < 5:
return "Your string is too short"
elif len(x) > 5:
return "Your string is too long"
else:
return True
This defines a function named just_right
which receives one string named x
. If the length of that string is less than 5 we return that the string is too short. Otherwise, if the string length is greater than 5 we return that it's too long. But if it's not either of those (meaning it is exactly equal to 5) then we return True. Indicating, of course, that the string length is "just right".
Hope this helps!
Christopher Kell
3,499 PointsChristopher Kell
3,499 PointsThank you very much! I'm not sure why I didn't even think of that.