Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial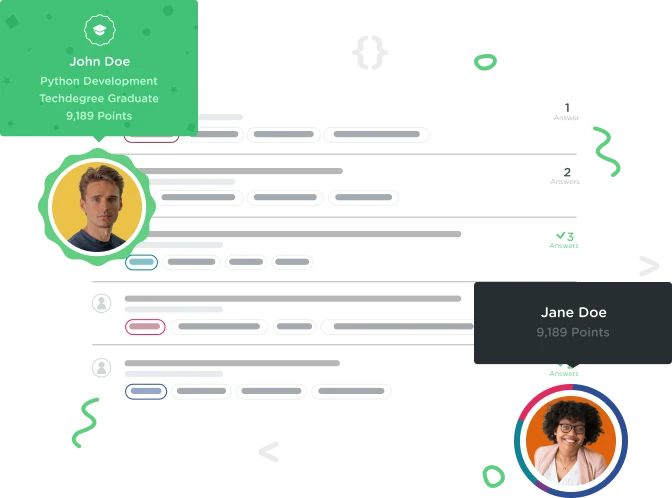
3 Answers
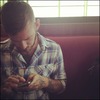
Erik McClintock
45,783 PointsMatthew,
To better understand this, let's break it down step by step, as they do in the challenge:
1) "You've got the $.get() method and first argument in place. The second argument to the $.get() method is a callback function. Pass an anonymous function as the second argument to the method. Don't forget to set a parameter for that function to catch the server's incoming response."
Initial code, prior to any editing from us:
$.get("footer.html");
From the video, you may recall that this is a function prepared for you in jQuery that allows for you to make AJAX calls a bit more simply. This particular function accepts two arguments: the first being the URL to which you're sending your request, and the second being the anonymous function that you want to run when the call is made. Here, in the first task of the challenge, they are simply asking that you insert your anonymous function into its proper place as the second argument, and to give it the appropriate parameter. Thus, we end up with the following code:
$.get("footer.html", function(response){
});
2) "Inside the anonymous function, use jQuery to select the footer div. It has an ID of 'footer'."
Here, they are asking you to access the footer div from your DOM that has an ID of footer. We know from plain ol' JavaScript where/how to write code inside of a function body (which is made easier to keep track of when you split the code onto multiple lines, as I've done in these examples), and from these videos (and/or perhaps from previous jQuery training, such as the jQuery courses that Treehouse provides) we know how to select HTML elements in jQuery, so we need to simply put the two together. First, we need to get inside the body of the anonymous function that we've declared:
$.get("footer.html", function(response){
//this is the inside of our anonymous function
});
And then we need to select the div with the ID of "footer":
$.get("footer.html", function(response){
//select the div with the ID of "footer"
$("#footer");
});
3) "Use jQuery's html() method to insert the server response into the footer of the page."
Lastly, we will use another jQuery method that we've learned in the videos of this course, the .html() method, to alter the HTML content inside of the $("#footer") div that we've just gained access to. Again, from these videos or from previous experience, we know how to call a function on an element in jQuery, so we will first do that:
$.get("footer.html", function(response){
//call the .html() method on the $("#footer") div
$("#footer").html();
});
And then we will pass in the argument that we want to set as the actual value of that HTML, in this case, the information contained in our "response" variable:
$.get("footer.html", function(response){
//pass the information contained in our "response" variable into the .html() method
$("#footer").html(response);
});
Once we've done all of these steps, we have a working AJAX call.
I hope that breaking it down into smaller steps like this has helped you to understand what they're asking. As always, if you're having difficulties, I'd recommend against moving forward until you've gone back however many videos/exercises you need to to truly grasp the material first. You'll better serve yourself for it!
Happy coding!
Erik
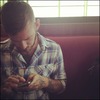
Erik McClintock
45,783 PointsMatthew,
In order for us to help you, you'll need to:
a) post the code that you've tried that isn't working, and b) tell us what specifically you need assistance with
Bring these two things to the topic and we'll get you back on the right track in no time!
Erik
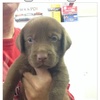
Matthew Clark
7,255 Pointsvar callback = ; $.get("footer.html", { #footer; }, function(response) { });