Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial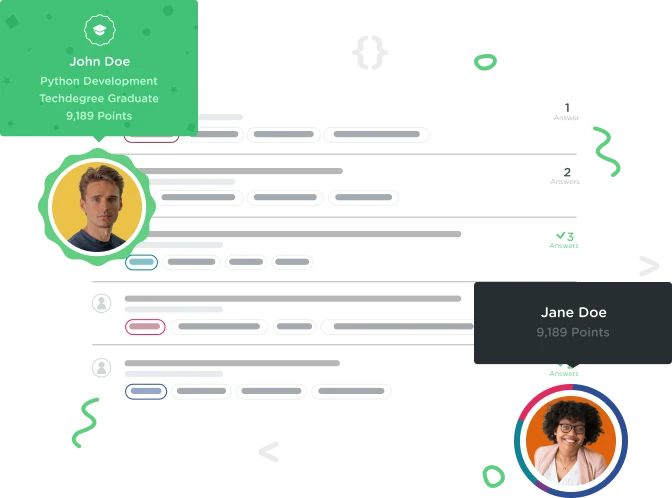

abdi ali
10,920 Pointshelp
Using the loop construct, add the current value of number to the numbers array. Inside of the loop, add 1 to the number variable. If the numbers array has more than 3 items, use the break keyword to exit the loop.
numbers = []
number = 0
numbers.push(number)
loop do
number = number + 1
if numbers > 3
break
end
end
# write your loop here
1 Answer
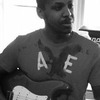
Samuel Webb
25,370 PointsFirst: You should be pushing number
into the numbers
array inside of the loop. You have to push the value onto the end during every iteration.
Second, you should be looking for the numbers
array to contain more than 3 items, not the number
variable to be greater than 3. You would accomplish this with numbers.count > 3
or numbers.length > 3
. They're the same thing.
My code looks like the following. I had to use ==
instead of >
because of the order of events:
numbers = []
number = 0
number += 1
loop do
numbers.push(number)
if numbers.count == 3
break
end
end
Samuel Webb
25,370 PointsSamuel Webb
25,370 PointsIf you decide to do your
push
after theif
statement, you'll have to change from==
to>