Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial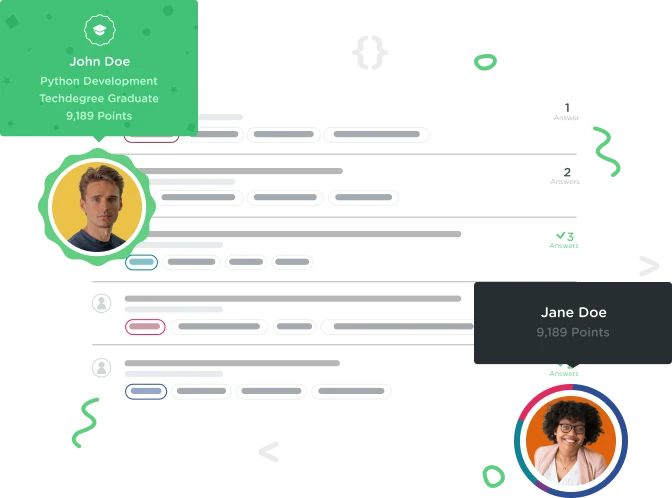

Austin Calvelage
Front End Web Development Techdegree Student 3,706 PointsHelp!
I cannot get it to document.write to work when you score 2 or 4 points. And does the rest of this look okay?
//Starting points
var points = 0;
/* question + answer.
If correct +1 pt and display alert
if wrong display alert and +0pt
*/
var answer1 = "COLUMBUS";
alert('Welcome to my Quiz! Try to do your best. Good LUCK!');
var question1 = prompt('What is the capitol of Ohio?');
if (question1.toUpperCase() === answer1) {
answer1 = true
points += 1;
alert('Good Job! You are correct. You have ' + points + ' points.');
} else {
alert('Sorry, that\'s incorrect! You have ' + points + ' points.');
}
var answer2 = 365;
var question2 = prompt('How many days are in a year?')
if (parseInt(question2) === 365) {
answer2 = true
points += 1;
alert('Good Job! You are correct. You have ' + points + ' points.');
} else {
alert('Sorry, that\'s incorrect! You have ' + points + ' points.');
}
var answer3 = "RUBY"
var question3 = prompt('What programing language is named after a gem?');
if (question3.toUpperCase() === answer3) {
answer3 = true
points += 1;
alert('Good Job! You are correct. You have ' + points + ' points.');
} else {
alert('Sorry, that\'s incorrect! You have ' + points + ' points.');
}
var answer4 = 50
var question4 = prompt('How many states are there in America?')
if (parseInt(question4) === answer4) {
answer4 = true
points += 1;
alert('Good Job! You are correct. You have ' + points + ' points.');
} else {
alert('Sorry, that\'s incorrect! You have ' + points + ' points.');
}
var answer5 = "BLUE";
var question5 = prompt('What is the color of the sky?');
if (question5.toUpperCase() === answer5) {
answer5 = true
points += 1;
alert('Good Job! You are correct. You have ' + points + ' points.');
} else {
alert('Sorry, that\'s incorrect! You have ' + points + ' points.');
}
/*
Reward System
5pts = Golden
3-4pts = silver
1-2pts = bronze
0pts = no medal
*/
var gold = 5;
var silver = 3 || 4;
var bronze = 1 || 2;
var loser = 0;
if (points === gold) {
document.write('<p>You are awarded the Golden Medal for answering ' + points + ' of the 5 questions right!</p>');
} else if (points === silver) {
document.write('<p>You are awarded the Silver Medal for answering ' + points + ' of the 5 questions right!</p>');
} else if (points === bronze) {
document.write('<p>You are awarded the Bronze Medal for answering ' + points + ' of the 5 questions right!</p>');
} else if (points === loser) {
document.write('<p>Sorry. Losers don\'t get any medals. You got ' +points + ' of the 5 questions right.</p>');
}
2 Answers
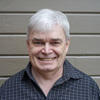
Dale Severude
Full Stack JavaScript Techdegree Graduate 71,350 PointsThat would work, but has excessive lines of code. I would combine some of it as follows to reduce redundant lines of code using document.write.
} else if (points === 4 || points === 3 ) {
} else if (points === 2 || points === 1 ) {
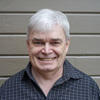
Dale Severude
Full Stack JavaScript Techdegree Graduate 71,350 PointsHi Austin, This var declaration is not allowed, that is why you never see 4 or 2 used, only 3 and 1 are getting set. You will have to use a conditional statement to set these.
var silver = 3 || 4;
var bronze = 1 || 2;

Austin Calvelage
Front End Web Development Techdegree Student 3,706 PointsSo like something like this would be okay?
if (points === 5) {
document.write('<p>You are awarded the Golden Medal for answering ' + points + ' of the 5 questions right!</p>');
} else if (points === 4) {
document.write('<p>You are awarded the Silver Medal for answering ' + points + ' of the 5 questions right!</p>');
} else if (points === 3) {
document.write('<p>You are awarded the Silver Medal for answering ' + points + ' of the 5 questions right!</p>');
} else if (points === 2) {
document.write('<p>You are awarded the Bronze Medal for answering ' + points + ' of the 5 questions right!</p>');
} else if (points === 1) {
document.write('<p>You are awarded the Bronze Medal for answering ' + points + ' of the 5 questions right!</p>');
} else if (points === 0) {
document.write('<p>Sorry. Losers don\'t get any medals. You got ' + points + ' of the 5 questions right.</p>');
}