Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial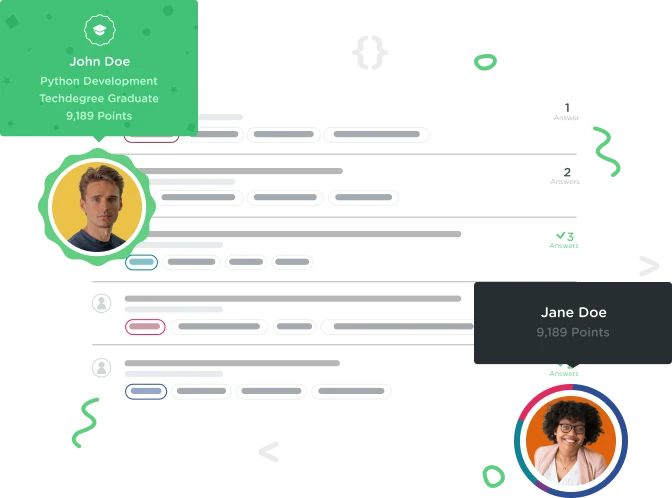
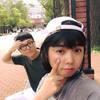
Minwook Jeong
2,453 PointsHelp me out the challenge question
Quenstion:
Alright, last step but it's a big one. Make a while loop that runs until start is falsey. Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99. If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number. Finally, decrement start by 1. I know it's a lot, but I know you can do it!
really confusing how I should express this question with Python language..
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
while start == False:
return not num%2
num = random.randint(1, 99)
if num %2 == 0:
print("{} is even".format(num))
else:
print("{} is odd".format(num))
2 Answers
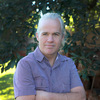
Christopher Shaw
Python Web Development Techdegree Graduate 58,248 PointsYou should not put the while loop inside the function, the function should have been left as it was. You just call the function as you iterate the the while loop to determine if the random number is odd or even.
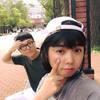
Minwook Jeong
2,453 Pointsimport random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
if num %2 == 0:
print("{} is even".format(num))
else:
print("{} is odd".format(num))
return not num % 2
while start:
number = random.randint(1, 99)
even_odd(number)
start -= 1
Here is my solution, I referred to what Christopher advised me
def even_odd(num) is a sole tool to judge whether the random number selected by Python is odd or even, it doesnt have any function of loop so as Christopher I put while loop out of even_odd(num)
Then, we need to think about which loop we are going to use, Is it For Loop? or While Loop? I cannot explain it logically but I went with While Loop (based on how we use 'start' before)
while start: -> we set up the ending point of loop as start, while loop will end till the value is False which is 0
so while loop would begin from 5, and stop at 1 since we set up the decrement -1
Thus, it will provide 5 value
Minwook Jeong
2,453 PointsMinwook Jeong
2,453 Pointshelped me a lot Thanks!
Khaleel Yusuf
15,208 PointsKhaleel Yusuf
15,208 PointsCan you please explain further? I don't understand. Thank you.