Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial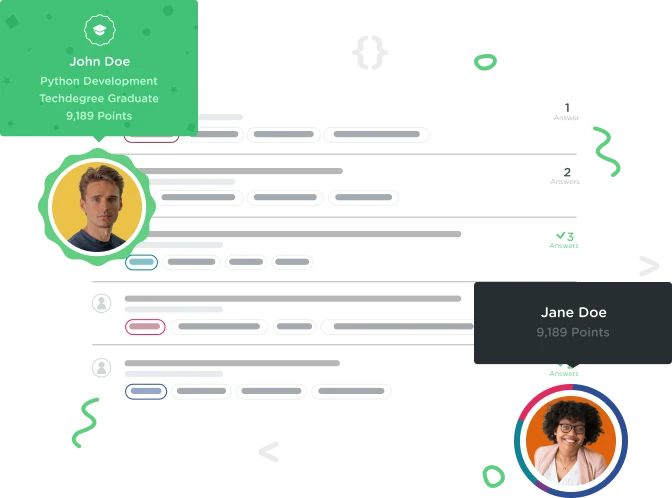
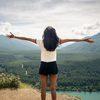
aroshinemunasinghe
5,649 PointsHelp! My app doesn't want to run after runOnUiThred(..)
Here are my code, and I dont understand why it doesnt want to run please help!
package com.treehouse.stormy;
import ...
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather mCurrentWeather;
@BindView(R.id.timeLabel)
TextView mTimeLabel;
@BindView(R.id.temperatureLabel)
TextView mTemperatureLabel;
@BindView(R.id.humidityValue)
TextView mHumidityValue;
@BindView(R.id.precipValue)
TextView mPrecipValue;
@BindView(R.id.summaryLabel)
TextView mSummaryLabel;
@BindView(R.id.iconImageView)
ImageView mIconImageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.bind(this);
String apiKey = "8dee957bc90d6604dceb3ac2b2d02895";
double latitude = 37.8267;
double longitude = -122.423;
String forecastURL = "https://api.forecast.io/forecast/" + apiKey + "/" + latitude + "," + longitude;
if (isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder().url(forecastURL).build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
updateDisplay();
}
});
} else {
alterUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
} catch (JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
} else {
Toast.makeText(this, getString(R.string.network_unavailable_message), Toast.LENGTH_LONG).show();
}
Log.d(TAG, "Main YI code is running!");
}
private void updateDisplay() {
mTemperatureLabel.setText(mCurrentWeather.getTemperature() + "");
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException { //jag tror för att omformattera till json koder??
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From JSON: " + timezone);
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setTimeZone(timezone);
Log.d(TAG, currentWeather.getFormattedTime());
return new CurrentWeather(); // man kan också ropa null, denna betyder att man skapar nytt tomt object
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
private void alterUserAboutError() {
AlterDialogFragment dialog = new AlterDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
4 Answers
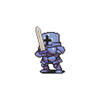
Judah Devasahayam
Full Stack JavaScript Techdegree Graduate 22,610 PointsTry changing this "return new CurrentWeather();" to this "return currentWeather;"
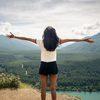
aroshinemunasinghe
5,649 Pointshere is the error:
FATAL EXCEPTION: main Process: com.treehouse.stormy, PID: 4680
java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference at com.treehouse.stormy.MainActivity.updateDisplay(MainActivity.java:103) at com.treehouse.stormy.MainActivity.access$200(MainActivity.java:26) at com.treehouse.stormy.MainActivity$1$1.run(MainActivity.java:79) at android.os.Handler.handleCallback(Handler.java:739) at android.os.Handler.dispatchMessage(Handler.java:95) at android.os.Looper.loop(Looper.java:148) at android.app.ActivityThread.main(ActivityThread.java:5417) at java.lang.reflect.Method.invoke(Native Method) at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:726) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:616)
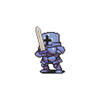
Judah Devasahayam
Full Stack JavaScript Techdegree Graduate 22,610 PointsYou don't need to use the parenthesis after "return currentWeather; ". Remember currentWeather is an instance not a method.
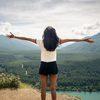
aroshinemunasinghe
5,649 PointsAHHH sorry! ofc currentWeather with no () hehee
But it didn't work it says: java.lang.NullPointerException: Attempt to invoke virtual method 'void android.widget.TextView.setText(java.lang.CharSequence)' on a null object reference
private void updateDisplay() {
mTemperatureLabel.setText(mCurrentWeather.getTemperature() + "");
}
at public class MainActivity extends AppCompactActivity{ at
public void onResponse(Call call, Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
updateDisplay();
}
});
} else {
alterUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
} catch (JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
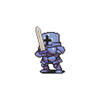
Judah Devasahayam
Full Stack JavaScript Techdegree Graduate 22,610 PointsDoes your getTemperature() have any value? or did you change its value from double to int?
public int getTemperature() {
double celsius = (mTemperature - 32.0) * 0.5555;
return (int)Math.round(celsius);
}
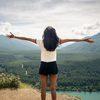
aroshinemunasinghe
5,649 Pointsaha, well my getTemperature was
public double getTemperature() {
return mTemperature;
}
So after when I changed getTemperature it stopped again. Same warning Fatal Exception; Nullpointerexception there updateDisplay() ...
here is MainActivity.java , after I changed currentWeather..
package com.treehouse.stormy;
import ...
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather mCurrentWeather;
@BindView(R.id.timeLabel)
TextView mTimeLabel;
@BindView(R.id.temperatureLabel)
TextView mTemperatureLabel;
@BindView(R.id.humidityValue)
TextView mHumidityValue;
@BindView(R.id.precipValue)
TextView mPrecipValue;
@BindView(R.id.summaryLabel)
TextView mSummaryLabel;
@BindView(R.id.iconImageView)
ImageView mIconImageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.bind(this);
String apiKey = "8dee957bc90d6604dceb3ac2b2d02895";
double latitude = 37.8267;
double longitude = -122.423;
String forecastURL = "https://api.forecast.io/forecast/" + apiKey + "/" + latitude + "," + longitude;
if (isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder().url(forecastURL).build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
updateDisplay();
}
});
} else {
alterUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
} catch (JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
} else {
Toast.makeText(this, getString(R.string.network_unavailable_message), Toast.LENGTH_LONG).show();
}
Log.d(TAG, "Main YI code is running!");
}
private void updateDisplay() {
mTemperatureLabel.setText(mCurrentWeather.getTemperature() + "");
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException { //jag tror för att omformattera till json koder??
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From JSON: " + timezone);
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setTimeZone(timezone);
Log.d(TAG, currentWeather.getFormattedTime());
return currentWeather; // man kan också ropa null, denna betyder att man skapar nytt tomt object
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
private void alterUserAboutError() {
AlterDialogFragment dialog = new AlterDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
Here is CurrentWeather.java* , changed getTemperature() :
public class CurrentWeather {
private String mIcon;
private long mTime;
private double mTemperature;
private double mHumidity;
private double mPrecipChance;
public String getTimeZone() {
return mTimeZone;
}
public void setTimeZone(String timeZone) {
mTimeZone = timeZone;
}
private String mTimeZone;
public String getSummary() {
return mSummary;
}
public void setSummary(String summary) {
mSummary = summary;
}
private String mSummary;
public String getIcon() {
return mIcon;
}
public void setIcon(String icon) {
mIcon = icon;
}
public int getIconId() {
//clear-day, clear night...
int iconId = R.drawable.clear_day;
if (mIcon.equals("clear-day")) {
iconId = R.drawable.clear_day;
} else if (mIcon.equals("clear-night")) {
iconId = R.drawable.clear_night;
} else if (mIcon.equals("rain")) {
iconId = R.drawable.rain;
} else if (mIcon.equals("snow")) {
iconId = R.drawable.snow;
} else if (mIcon.equals("sleet")) {
iconId = R.drawable.sleet;
} else if (mIcon.equals("wind")) {
iconId = R.drawable.wind;
} else if (mIcon.equals("fog")) {
iconId = R.drawable.fog;
} else if (mIcon.equals("cloudy")) {
iconId = R.drawable.cloudy;
} else if (mIcon.equals("partly-cloudy-day")) {
iconId = R.drawable.partly_cloudy;
} else if (mIcon.equals("partly-cloudy-night")) {
iconId = R.drawable.cloudy_night;
}
return iconId;
}
public long getTime() {
return mTime;
}
public String getFormattedTime() {
SimpleDateFormat formatter = new SimpleDateFormat("h:mm a");
formatter.setTimeZone(TimeZone.getTimeZone(getTimeZone()));
Date dateTime = new Date(getTime() * 1000);
String timeString = formatter.format(dateTime);
return timeString;
}
public void setTime(long time) {
mTime = time;
}
public int getTemperature() {
double celsius = (mTemperature - 32.0) * 0.5555;
return (int) Math.round(celsius);
}
public void setTemperature(double temperature) {
mTemperature = temperature;
}
public double getHumidity() {
return mHumidity;
}
public void setHumidity(double humidity) {
mHumidity = humidity;
}
public double getPrecipChance() {
return mPrecipChance;
}
public void setPrecipChance(double precipChance) {
mPrecipChance = precipChance;
}
}
and last AlterDialogFragment.java :
/** DENNA KLASSEN HANDLAR OM NÄR ANDRIOD FÅR ERROR SÅ DET KOMMER UPP EN RUTA, KOLLA TREEHOUSE Configuering the Alert Dialog**/
public class AlterDialogFragment extends DialogFragment {
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
Context context = getActivity();
AlertDialog.Builder builder = new AlertDialog.Builder(context)
.setTitle(context.getString(R.string.error_title))
.setMessage(context.getString(R.string.error_message))
.setPositiveButton(context.getString(R.string.error_ok_button_text), null); //null för att det ska inte hända nåt låt appen rulla typ
AlertDialog dialog = builder.create();
return dialog;
}
}
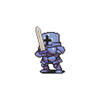
Judah Devasahayam
Full Stack JavaScript Techdegree Graduate 22,610 Pointsadd this to getPercipChance()
public double getPrecipChance() {
double precipPercentage = mPrecipChance *100;
return (int)Math.round(precipPercentage);
}
aroshinemunasinghe
5,649 Pointsaroshinemunasinghe
5,649 PointsHi sorry for very late message it didnt work it said error: cannot find symbol method currentWeather()
Judah Devasahayam
Full Stack JavaScript Techdegree Graduate 22,610 PointsJudah Devasahayam
Full Stack JavaScript Techdegree Graduate 22,610 Pointsdon't add '()' to currentWeather.
return currentWeather;