Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial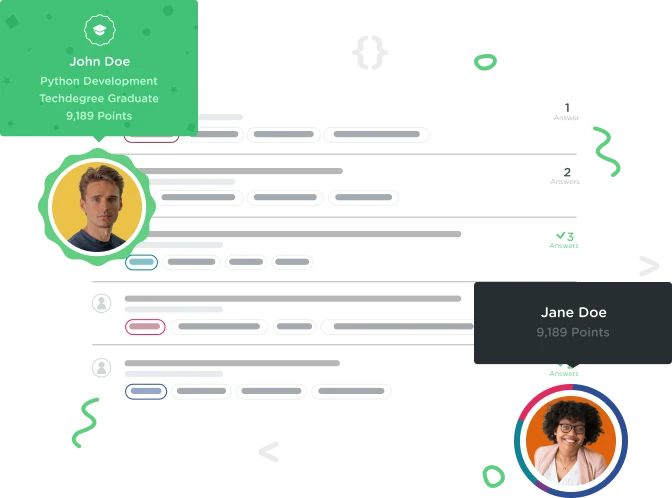

Jessica Orellanes
11,245 PointsHelp: Why is my code making empty list items?
My solution is different from Dave's. in fact it is not a solution since i was not able to make the ordered list correctly.
If you happen to know why i'm getting empty list items, please explain it to me.
thanks in advanced.
var quiz = [
['¿Cual es la capital de Venezuela? ', 'CARACAS'],
['¿Cual es la capital de Italia? ', 'ROMA'],
['¿Cual es la capital de España? ', 'MADRID']
];
var answer;
var html = '';
var right = 0;
var wrong = 0;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for ( i = 0 ; i < quiz.length; i +=1 ) {
answer = prompt(quiz[i][0]);
answer = answer.toUpperCase();
if (answer === quiz[i][1]) {
right += 1;
quiz[i].push(1);
} else {
wrong += 1;
quiz[i].push(0);
}
}
if (right > 0) {
html += '<p>Respuesta(s) correcta(s): ' + right + '<p/>';
html += '<ol>'
for ( i = 0 ; i < quiz.length; i +=1 ) {
if (quiz[i][2] === 1) {
html += '<li>' + ' ' + quiz[i][0] + ' ' + quiz[i][1] + '<li/>';
} else
html +='';
}
html += '</ol>'
}
if (wrong > 0) {
html += '<p>Respuesta(s) incorrecta(s): ' + wrong + '<p/>';
html += '<ol>'
for ( i = 0 ; i < quiz.length; i +=1 ) {
if (quiz[i][2] === 0) {
html += '<li>' + ' ' + quiz[i][0] + ' ' + '<li/>';
}else
html +='';
}
html += '</ol>'
}
print(html);
3 Answers
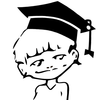
simhub
26,544 Pointshi Jessica, maybe it's just a typo
</p>
</li>
instead of
<p/>
<li/>

Yann Vanhalewyn
18,687 PointsHow about this?
var questions = [
{ question: '¿Cual es la capital de Venezuela? ', answer:'CARACAS' },
{ question: '¿Cual es la capital de Italia? ', answer: 'ROMA' },
{ question: '¿Cual es la capital de España? ', answer: 'MADRID' }
];
var answers = [];
// Prompt user for answers
questions.forEach(function(question) {
var answer = prompt(question.question);
answers.push({
originalQuestion: question.question,
validAnswer: question.answer,
userAnswer: answer,
isCorrect: (answer.toUpperCase() === question.answer)
});
});
// Separate the answers into a correct array and a false array
var correctAnswers = answers.filter(function(answer) { return answer.isCorrect; })
var falseAnswers = answers.filter(function(answer) { return !answer.isCorrect; })
// Transform the correct-list into html
var correctListItems = correctAnswers.map(function(answer) {
return "<li>" + answer.originalQuestion + " CORRECT </li>";
});
// Transform the false-list into html
var falseListItems = falseAnswers.map(function(answer) {
return "<li>" + answer.originalQuestion + " FALSE - You answered '" +
answer.userAnswer + "' instead of '" + answer.validAnswer + "'</li>";
});
// A helper for transforming an array of li tags into valid html list.
function wrapListItemsInListTags(listItemsArray) {
return "<ol>" + listItemsArray.join("\n") + "</ol>";
};
var outputHTML = "Correct answers (" + correctAnswers.length + ")" +
wrapListItemsInListTags(correctListItems) +
"False answers (" + falseAnswers.length + ")" +
wrapListItemsInListTags(falseListItems);
document.getElementById('output').innerHTML = outputHTML;
Don't forget some very useful Array.prototype functions like map
, filter
and join
. Try to be more explicit when accessing data. question.answer
is more readable than quiz[i][1]
:).

Yann Vanhalewyn
18,687 PointsOh, the title said 'please refactor my code' when I wrote my answer. If you're just looking for the bug, it's the <li/>
typos, like simhub said.

Jessica Orellanes
11,245 PointsThanks Yann,
I just wanted to call your attention on the error but the refactoring i'm interested the most now.