Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial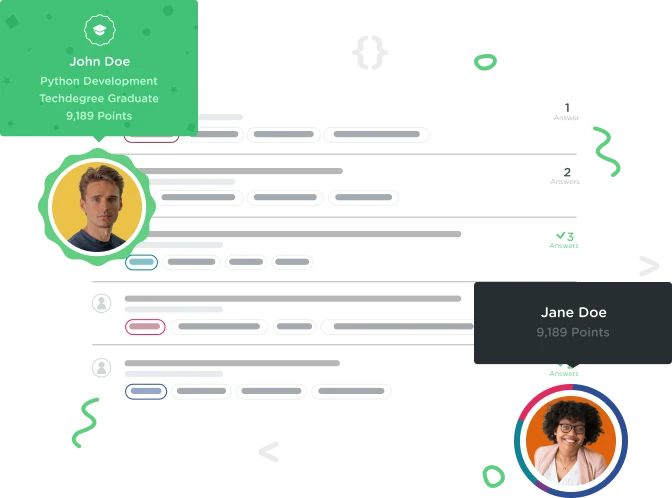
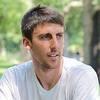
Dennis Stephens
2,864 PointsHelp with code challenge 2/2: set intersection
Great work! OK, let's create something a bit more refined. Create a new function named covers_all that takes a single set as an argument. Return the names of all of the courses, in a list, where all of the topics in the supplied set are covered. For example, covers_all({"conditions", "input"}) would return ["Python Basics", "Ruby Basics"]. Java Basics and PHP Basics would be exclude because they don't include both of those topics.
I am receiving and error for checking the intersection between a str and set which I understand. However, I cannot seem to figure out how to pass the values as a set. Please help, thank you
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(inp):
new_list = []
for item in COURSES:
if COURSES[item] & inp:
new_list.append(item)
return new_list
def covers_all(inp):
new_list = []
for course in COURSES:
for item in COURSES[course]:
if item & inp:
new_list.append(course)
return new_list
3 Answers
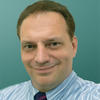
Mark Jones
Full Stack JavaScript Techdegree Graduate 29,362 PointsI got the challenge to pass with this
def covers_all(arg):
course_list = []
for item in COURSES.items():
if not arg - item[1]:
course_list.append(item[0])
return course_list

Jason Anello
Courses Plus Student 94,610 PointsHi Dennis,
For this second one, you can keep the exact same structure as you have for the first one but you're going to handle the if
condition a little differently.
For the first one, it was enough to make sure the intersection had at least one topic in it in order to append the course.
In the second one, you want to make sure that the intersection is equal to the set that was passed in.
Also, this is known as a superset which gives you another way to solve it.
You could either do a.issuperset(b)
and this will check if set a contains everything that is in set b. In other words, if set a is a superset of set b.
Alternatively, it can be written as a >= b
.

Rob Delwo
1,799 PointsI think you mean issubset not issuperset
if topic.issubset(COURSES[course]):

Jason Anello
Courses Plus Student 94,610 PointsHi Rob,
You could use either one.
In your example, you're checking if the topic set passed in is a subset of the course topics.
But you could also check if the course topics are a superset of the topics passed in.
if COURSES[course].issuperset(topic):
Either way should work out depending on how you'd like to think about the problem.
In general, if A is a subset of B, then B is a superset of A.

Swapnil Paralkar
3,359 PointsI get this error on python 3.6
TypeError: unsupported operand type(s) for &: 'str' and 'str'
def covers_all(inp):
new_list = []
for course in COURSES:
for item in COURSES[course]:
if item & inp:
new_list.append(course)
return new_list