Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial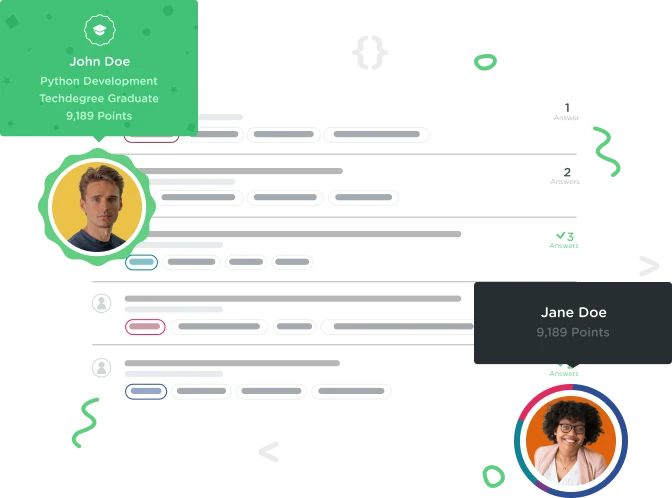
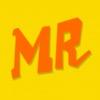
Manny Rothman
6,653 PointsHelp with Swift 3 switches
In the editor we have a dictionary that contains a three letter country code as a key and that country's capital city as the associated value.
We also have three empty arrays, europeanCapitals, asianCapitals, and otherCapitals. The goal is to iterate through the dictionary and end up with just the names of the capital cities in the relevant array.
For example, after you execute the code you write, europeanCapitals will have the values ["Vaduz", "Brussels", "Sofia"] (not necessarily in that order).
To do this you're going to use a switch statement and switch on the key. For cases where the key is a European country, append the value (not the key!) to the europeanCapitals array. For keys that are Asian countries, append the value to asianCapitals and finally for the default case, append the values to otherCapitals.
What am I doing wrong?
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
// Enter your code below
switch world {
case "BEL", "LIE", "BGR": var europeanCapitals.append("Brussels", "Vaduz", "Sofia")
case "IND", "VNM": var asianCapitals.append("New Delhi", "Hanoi")
default: var otherCapitals.append("Washington D.C.", "Mexico City", "Brasilia")
}
// End code
}
5 Answers
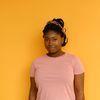
Marilyn Magnusen
14,084 Points var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
// Enter your code below
switch key {
case "BEL", "LIE", "BGR": europeanCapitals.append(value)
case "IND", "VNM": asianCapitals.append(value)
default: otherCapitals.append(value)
}
// End code
}
```
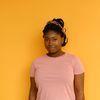
Marilyn Magnusen
14,084 PointsThis is my first time posting a reply, forgive the indentation.
These are the steps I took:
- the result of all this is that we want each of the arrays to store the relevant values, so europeanCapitals will have :Brussels, Vaduz, Sofia asianCapitals will have New Dehli, Hanoi, otherCapitals will have Washing DC, Mexico City, Brasilia
to do this
- the "for" statement has already been written out for us - leave this alone
- switch key (not value). The question specifically says "To do this you're going to use a switch statement and switch on the key"
- for the case, we can enter the keys in the array for example, "BEL" "LIE" "BGR"
- You don't need to explicitly write out the values as the compiler will compute this for us. But once the values are obtained, we do need to tell the compiler to append (add) these values to the relevant array. We do this using the .append method eg "europeanCapitals.append(value)"
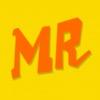
Manny Rothman
6,653 PointsAbdullah Ahmad , I updated my switch statement to be this:
switch world {
case "BEL", "LIE", "BGR": europeanCapitals.append(value)
case "IND", "VNM": asianCapitals.append(value)
default: otherCapitals.append(value)
}
I'm still getting an error:
swift_lint.swift:23:10: error: expression pattern of type 'String' cannot match values of type '[String : String]'
case "BEL", "LIE", "BGR": europeanCapitals.append(value)
^~~~~
swift_lint.swift:23:17: error: expression pattern of type 'String' cannot match values of type '[String : String]'
case "BEL", "LIE", "BGR": europeanCapitals.append(value)
^~~~~
swift_lint.swift:23:24: error: expression pattern of type 'String' cannot match values of type '[String : String]'
case "BEL", "LIE", "BGR": europeanCapitals.append(value)
^~~~~
swift_lint.swift:24:10: error: expression pattern of type 'String' cannot match values of type '[String : String]'
case "IND", "VNM": asianCapitals.append(value)
^~~~~
swift_lint.swift:24:17: error: expression pattern of type 'String' cannot match values of type '[String : String]'
case "IND", "VNM": asianCapitals.append(value)
^~~~~

Abdullah Ahmad
1,097 PointsIt looks good but for your switch statement, we are switching for the "key" not "world so that should start like "switch key" not "switch world. Here's the way I did it if you wanna reference it. There are multiple ways to do it like he said in the video so yeah.
for (key, value) in world { switch key { case "BEL", "LTE", "BGR": europeanCapitals.append(value) case "IND", "VNM": asianCapitals.append(value) case "USA", "MEX", "BRA": otherCapitals.append(value) default:print("Not found") } }

Linus Karlsson
7,402 PointsI am new to Swift.
How the h*** am I supposed to figure this out by myself after these few videos?!?
Should I feel stupid or should I blame the teacher in this case?
(Sorry for bad language)

Jens Aamand Øvig
1,776 PointsIve found that just typing it all works.. switch key { case "BEL": europeanCapitals.append("Brussels") case "LIE": europeanCapitals.append("Vaduz") case "BGR": europeanCapitals.append("Sofia") case "USA": otherCapitals.append("Washington D.C") case "Mex": otherCapitals.append("Mexico City") case "BRA": otherCapitals.append("Brasilia") case "IND": asianCapitals.append("New Delhi") default: asianCapitals.append("Hanoi") }}

Eddie Flickinger
3,513 PointsI've literally had to lookup the last 3 or 4 solutions completely. The teachers voice is hard to listen to, but what he covers are relatively easy concepts. The problems are not. Should I be struggling this much?

Richard Then
10,690 Pointsfor (key, value) in world {
switch key { case "BEL", "LIE", "BGR": europeanCapitals.append*(value)*
(key, value) is this standard when trying to refer to a key/value? and then switch key will only refer to halve of the contents in the parentheses(keys).
I didn't know that was even possible! I don't think Pasan went over this...
My coding was a mess trying to separate key/value ([String:String] to [String])!
Abdullah Ahmad
1,097 PointsAbdullah Ahmad
1,097 PointsI'm still new to coding as well so I apologize if this explanation is bad but you are on the right track from what I can see. The only things I see that should be corrected is, don't rewrite "var" because those variables have already been declared just call them by their name like "europeanCapitals", "asianCapitals" & "otherCapitals" NOT "var europeanCapitals" Also just call it asianCapitals.append(value) instead of listing the values there.