Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial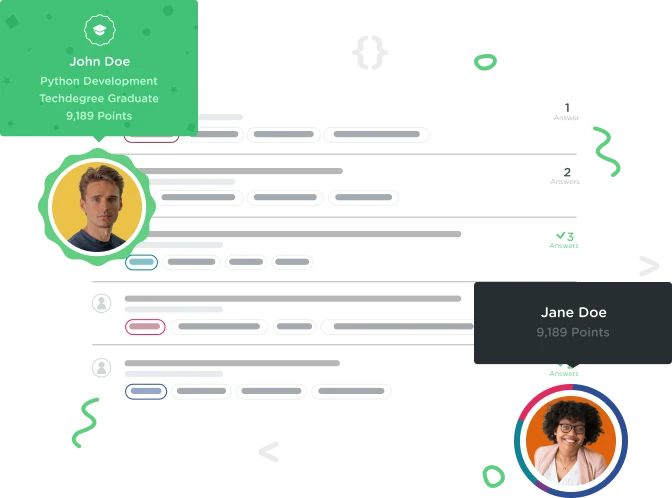
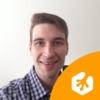
Frederick Pukay
Python Web Development Techdegree Student 22,222 PointsHelp with Teacher Stats
I'm really stuck on challenge 4 and 5 for Teacher Stats. I'm not quite sure how to go about solving the last 2 problems. Can you give me some advice as to where I may be going wrong?
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(dict):
return len(dict)
def num_courses(dict):
count = 0
for value in dict.values():
for course in value:
count += 1
return count
def courses(dict):
value_list = []
for value in dict.values():
value_list.extend(value)
return value_list
# Task 4 of 5 (problem with my code)
def most_courses(dict):
dict_count = {}
for key in dict.keys():
count = 0
for value in dict.values():
for course in value:
count += 1
dict_count[key] = count
key_max = max(dict_count.keys(), key=(lambda k: dict_count[k]))
return key_max
# Task 5 of 5 (problem with my code)
def stats(dict):
list_count = []
for key in dict.keys():
count = 0
for value in dict.values():
count = 0
for course in value:
count += 1
list_count.append([key, count])
return list_count
2 Answers
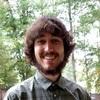
Zachary Jackson
Treehouse Project ReviewerTo add some additional trouble shooting tips in addition to what Brandon Oakes said, whenever you are having trouble with a challenge like this, one thing I like to do is put all of the code into something like workspaces. You are able to send the function various parameters like dictionaries of various sizes and lengths and see what happens. Print things out to the console or use the python debugger to see what values are. (Because you are using lambdas I presume you know about the debugger. If not you can find more information here: https://teamtreehouse.com/library/the-python-debugger )
For the two functions most_courses and stats you showed above. I would check out what is happening around the count and what the dictionary's keys and values are doing. Anytime you need both a key and value from a dictionary it is usually best to get them both at once using for key, value in dictionary.items():
This way you won't have to worry about getting the wrong key, value pair.
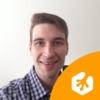
Frederick Pukay
Python Web Development Techdegree Student 22,222 PointsThanks Zachary. Your explanation make solving 5 of 5 very easy.
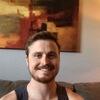
Brandon Oakes
Python Web Development Techdegree Student 11,501 PointsYou are very close and strong work incorporating lambda single expression functions. One way to do it with a little less code is shown below. First make a variable that you can compare the initial iteration too (count). Now iterate through each key/value pair and take the length of the value(which are list in our case) and if the length is greater than count, change count to that specific length and store the name as key_max. After the iteration is complete the name with the highest length will be stored in key_max and returned. Hopefully this helps clear things up for you.
# Task 4 of 5 (problem with my code)
def most_courses(dict):
# iterate each key/value pair
count = 0
for key in dict:
if len(dict[key]) > count:
count = len(dict[key])
key_max = key
return key_max
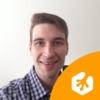
Frederick Pukay
Python Web Development Techdegree Student 22,222 PointsThank you Brandon. I appreciate you showing me a way to do this with less code :)
Frederick Pukay
Python Web Development Techdegree Student 22,222 PointsFrederick Pukay
Python Web Development Techdegree Student 22,222 PointsThink I just figured out 4 of 5. I checked the Markdown cheatsheet but not sure how to format the code below for this.
def most_courses(dict): new_dict = {} count = 0 for key in dict.keys(): for value in dict.values(): count += 1 new_dict[key] = count key_max = max(new_dict.keys(), key=(lambda k: new_dict[k])) return key_max