Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial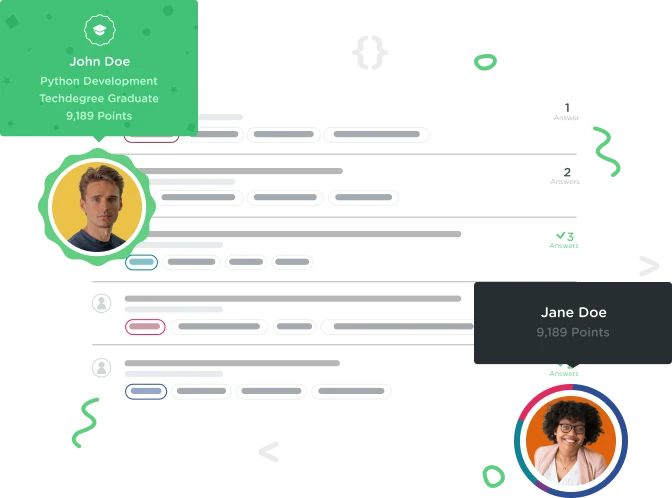
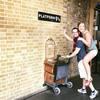
Tyler Nordmann
1,083 PointsHelp with Word Count. My function returns what I think is correct, but upon submission it fails.
def word_count(phrase):
space_index = []
key_list = []
phrase = phrase.lower()
count = 1
for letter in phrase:
upto = phrase[0:count]
if letter == " ":
space_index.append(len(upto)-1)
count += 1
count = 0
key_list = [phrase[0:space_index[0]]]
for num in space_index:
try:
nums = space_index[count:count+2]
key_list.append(phrase[nums[0]+1:nums[1]])
count += 1
except IndexError:
continue
key_list.append(phrase[space_index[len(space_index)-1]+1:len(phrase)])
counter = []
count= 0
for num in key_list:
counter.append(count)
count = 0
for item in key_list:
for thing in key_list:
if thing == item:
counter[count] += 1
count += 1
my_dict = dict(zip(key_list, counter))
return my_dict
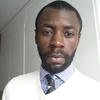
Marcus Sassi
4,394 PointsYou have done a great job, but try to simplify your code. Below you can find my code which works, please study it and see if you can gain anything from it. This code works but I also need find a way to simplify it even further.
myword ="I do not like it Sam I Am"
def word_count(sentence):
my_dict = {}
sentence = sentence.lower().split()
for sen in sentence:
if sen in my_dict:
count = my_dict[sen] + 1
my_dict[sen] = count
else:
my_dict[sen] = 1
return my_dict
Nikolay Nogin
22,154 PointsNikolay Nogin
22,154 PointsAs said Kenneth in the future courses, Maybe you need to look at editing? :) I mean, try to understand this code or find another solution here on forum, and you won't have to make a mountain out of a molehill: