Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial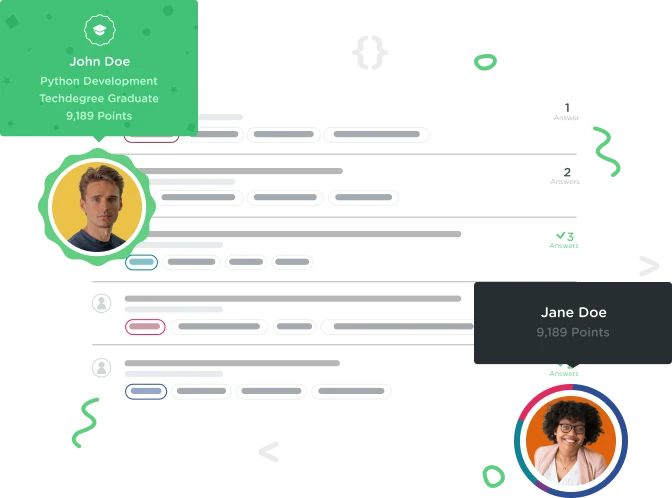

Alex Flores
7,864 PointsHere is my code with extra credit... minus the matching names
This took me longer than I'd like to admit, but I finally got it. The only thing I was able to complete is the matching names bonus, but I'm going to give that a shot now. I have a few questions in my comments, if anyone can answer them. Please let me know if you see anything I could have done better. Thanks for looking!
// declare my variables up here so that they are defined once the window opens.
var message = '';
var student;
var search;
var results;
// function to print html to the window
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
/*function to print the student report. I didn't include an argument for
this function.. Don't think I need to? */
function getStudentReport() {
var report = '<h2> Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
/* while loop to run the loop so long as the condition is true.
If the user cancels (null) or quits, the loop will break
*/
while (true) {
search = prompt('Search student records:');
results = [];
if (search === null || search.toLowerCase() === 'quit') {
break;
}
// for loop to cycle through the students array to match with the users search
for (var i = 0; i < students.length; i++) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase() ) {
results.push(student[i]);
message = getStudentReport(student);
print(message);
}
/* I created a results array to store the student records..
I tried using just a number value and doing results += 1 every
time there was a match, but this didn't work. Bonus points
the person that tells me why :) However, if the search didn't
have a match in students, nothing will be stored and this condition will pass.*/
if (results.length < 1) {
message = "<h2> Sorry, that person does not exist in our records. </h2>";
print(message);
}
}
}
EDIT: I just realized that if I type a wrong name after I have typed a correct name, it won't return the message stating that there are no students by that name.... I'm working on it, but any suggestions would be helpful.
EDIT x2: I figured it out. I started out trying to solve this by resetting the results variable after each event.
if (results.length < 1) {
message = "<h2> Sorry, that person does not exist in our records. </h2>";
print(message);
results.pop(student); /* also tried */ results = [];
}
but that wasn't working as I realized the logic behind it was flawed, because I want the variable to start out EACH time fresh, so it just made since to start it the while loop, because after it's loop it starts back at the while to check if it's still true. That's just the way I understand it so if I'm wrong, please let me know. If I've lost your interest at this point, sorry. I'm writing all of this out mostly for me :)
My formatting didn't work for EDIT x2?? Also, I just noticed how horrible it is to read my code. How come some comments are red and others green? Maybe a missed bracket?