Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial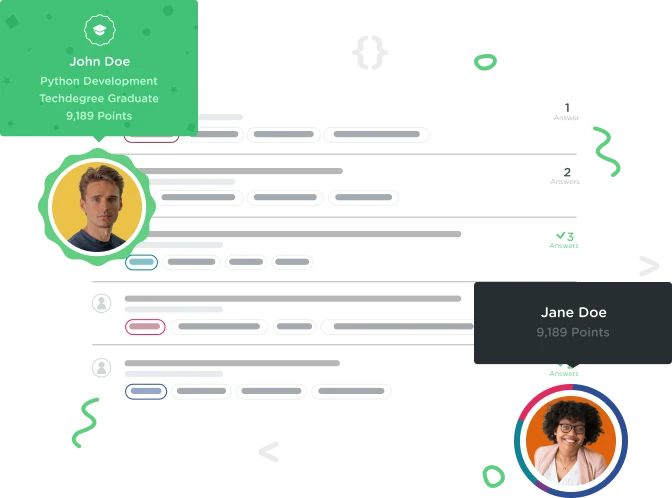
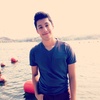
Luis Villarreal
10,040 PointsHere is my solution to the final challenge. Feel free to comment.
var student = '';
var html = '';
var search;
var message = '';
var MoreThenOneStudentWithSameName = 0;
//Prints out Student Final Result
function print(message){
var html = document.getElementById('output');
if(MoreThenOneStudentWithSameName >= 2){
html.innerHTML += message; //Prints more then one student with same name
} else {
html.innerHTML = message; //prints onely one student
}
}
//Student Report is Created
function getStudentReport( student){
var report = '<h2><b>Student: ' + student.name + '</b></h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
report += '<p>Points: ' + student.points + '</p>';
return report;
}
//Main Program
while(true){
MoreThenOneStudentWithSameName = 0; //Restart counter
search = prompt('Search Student Recods: type a name [Jody] or type "quit" to exit.'); //ask for student data.
search = search.toLowerCase();
//End the program if nothing is typed or they write quit.
if(search === null || search === 'quit' || search === ''){
break;
}
//Loop to check student records with the name provided
for(var i = 0; i < students.length; i+=1){
student = students[i];
if(student.name.toLowerCase() === search){
MoreThenOneStudentWithSameName += 1;
message = getStudentReport(student);
print(message);
}
}
//Why check for MoreThenOneStudentWithSameName? since, MoreThenOneStudentWithSameName never got executed we conclude that the loop ended with no result.
if(MoreThenOneStudentWithSameName === 0){
message = (search.charAt(0).toUpperCase() + search.slice(1) + ' is not in our system.');//Capitalize first letter in the name and display message.
print(message);
}
}
// End of Main Program
docgunthrop
12,445 Pointsdocgunthrop
12,445 PointsMy solution is functionally similar, but quicker and dirtier (accomplishes the minimum required functionality):
One thing to note is that you declared the variable "html" twice in your function. the first as a global string and again inside the nested print function. I'd suggest changing the name of one or the other to minimize confusion and potential errors in the intended functionality of your code.