Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial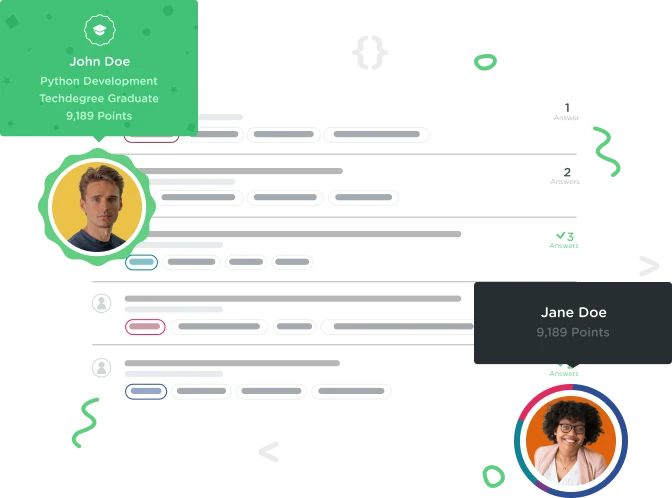
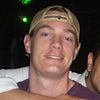
Joseph Rimar
17,101 PointsHere's my first Attempt. Any ideas on how to make it a little more efficient?
//Ask user questions
var questionOne = prompt("What is 10 times 5?");
var questionTwo = prompt("What is the capital of Virginia?");
var questionThree = prompt("How many weeks are there in a year?");
var questionFour = prompt("How old must you be to receive social security?");
var questionFive = prompt("Who was the first US President?");
var numberCorrect = 0;
//Store correct Answers
var answerOne = 50;
var answerTwo = "RICHMOND";
var answerThree = 52;
var answerFour = 65;
var answerFive = "WASHINGTON";
// Validate User Answers
if (answerOne === parseInt(questionOne)) {
numberCorrect ++;
}
if (answerTwo === questionTwo.toUpperCase()) {
numberCorrect ++;
}
if (answerThree === parseInt(questionThree)) {
numberCorrect ++;
}
if (answerFour === parseInt(questionFour)) {
numberCorrect ++;
}
if (answerFive === questionFive.toUpperCase()) {
numberCorrect ++;
}
//Set numberCorrect Appropriately
if (numberCorrect === 5) {
document.write("Congrats. You get a golden crown!");
} else if (numberCorrect === 4 || numberCorrect === 3) {
document.write("Good job! You've earned the silver crown.")
} else if (numberCorrect === 2 || numberCorrect === 1) {
document.write("Well. You've at least gotten a brass crown for trying!");
} else {
document.write("You'd better study up. You have zero correct answers!");
}
3 Answers
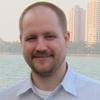
Ryan Field
Courses Plus Student 21,242 PointsFor me, I would do something like this to keep the Qs and As nice and together. :)
(function () {
var questions = [
{q: "What is 10 times 5?", a: "50"},
{q: "What is the capital of Virginia?", a: "RICHMOND"},
{q: "How many weeks are there in a year?", a: "152"},
{q: "How old must you be to receive social security?", a: "65"},
{q: "Who was the first US President?", a: "WASHINGTON"}
];
var numberCorrect = 0;
for (var i = 0, len = questions.length; i < len; i++) {
var answer = prompt(questions[i]["q"]).toUpperCase();
if (answer === answers[i]["a"]) {
numberCorrect++;
}
}
if (numberCorrect === 5) {
document.write("Congrats. You get a golden crown!");
} else if (numberCorrect > 2) {
document.write("Good job! You've earned the silver crown.")
} else if (numberCorrect > 0) {
document.write("Well. You've at least gotten a brass crown for trying!");
} else {
document.write("You'd better study up. You have zero correct answers!");
}
})();
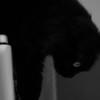
moonshine
8,449 PointsThis is a bit cleaner.
(function () {
var questions = [
"What is 10 times 5?",
"What is the capital of Virginia?",
"How many weeks are there in a year?",
"How old must you be to receive social security?",
"Who was the first US President?"
];
var answers = ["50", "RICHMOND", "152", "65", "WASHINGTON"];
var numberCorrect = 0;
for (var i = 0, len = questions.length; i < len; i++) {
var answer = prompt(questions[i]).toUpperCase();
if (answer === answers[i]) {
numberCorrect++;
}
}
if (numberCorrect === 5) {
document.write("Congrats. You get a golden crown!");
} else if (numberCorrect > 2) {
document.write("Good job! You've earned the silver crown.")
} else if (numberCorrect > 0) {
document.write("Well. You've at least gotten a brass crown for trying!");
} else {
document.write("You'd better study up. You have zero correct answers!");
}
})();
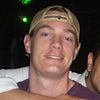
Joseph Rimar
17,101 PointsFloris. Thanks for the quick response. Your code is cleaner and more efficient. At the point of this challenge we haven't reached functions or loops yet so I think we were supposed to come up with a solution without using them. Thank you for your help and I'll definitely look to you for some advice on topics coming up!
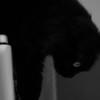
moonshine
8,449 PointsThe function just creates an enclosement. It prevents the window object from being cluttered with a bunch of new variables. Furthermore, the browser doesn't have to search through the entire window object each time it needs to access a variable.