Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial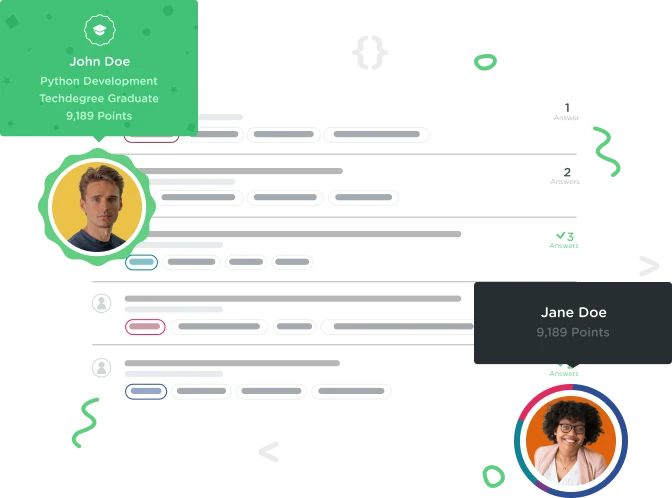

Robert Leonardi
17,151 PointsHere's my solution: both Jody comes up, and cleans up after quit.
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Jody',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
var answer = "";
do {
var html ="";
answer = prompt("Search student record: type student's name or 'Quit' to exit.");
for ( var studentDetail in students ){
if(answer.toUpperCase() === students[studentDetail].name.toUpperCase() ){
html += "<b>Student: " + students[studentDetail].name +"</b><br>";
html += "<ul>";
html += "<li>Track: "+students[studentDetail].track+"</li>";
html += "<li>Points: "+students[studentDetail].points+"</li>";
html += "<li>Achievements: "+students[studentDetail].achievements+"</li>";
html += "</ul>";
}
}
if(html === "" && answer.toUpperCase() !== "QUIT"){html += "<b>Student doesn't exist.</b><br>";}
print(html);
} while ( answer.toUpperCase() !== "QUIT" )
5 Answers

Robert Leonardi
17,151 PointsMy other alternative is to use
alert(message)
it will display immediately

Robert Leonardi
17,151 PointsHere's my updated version
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jody',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'Jody',
track: 'PHP Development1',
achievements: '552',
points: '20252'
},
{
name: 'Jody',
track: 'PHP Development2',
achievements: '553',
points: '20251'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Jody',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
var answer = "";var display_message ="";var count_answer=0;
while ( answer.toUpperCase() !== "QUIT" )
{
if(display_message != ""){alert(display_message);}
answer = prompt("Search student record: type student's name or 'Quit' to exit.");
if(display_message != ""){display_message =""};
count_answer=0;
for ( var studentDetail in students )
{
if(answer.toUpperCase() === students[studentDetail].name.toUpperCase() ){
count_answer ++;
display_message += "Student: " + students[studentDetail].name +"\n";
display_message += "Track: "+students[studentDetail].track+"\n";
display_message += "Points: "+students[studentDetail].points+"\n";
display_message += "Achievements: "+students[studentDetail].achievements+"\n\n";
}
}
if(count_answer>0){display_message = "Found "+count_answer+" answer(s) : \n\n" +display_message ;}
if(display_message === "" && answer.toUpperCase() !== "QUIT"){display_message += "Student doesn't exist.\n\n";}
}
print("Thank you for using this student search program.");
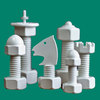
Steven Parker
231,007 PointsI'm guessing you're using an old browser.
On modern browsers, the page is not rendered until the script finishes. So by making your output empty in the loop, nothing will be seen in the browser at all.

Robert Leonardi
17,151 Pointsupdated firefox works fine. chrome doesn't.
however, i tried following teacher's instruction.. i changed mine
var answer = "";
while ( answer.toUpperCase() !== "QUIT" )
{
var html ="";
answer = prompt("Search student record: type student's name or 'Quit' to exit.");
for ( var studentDetail in students )
{
if(answer.toUpperCase() === students[studentDetail].name.toUpperCase() ){
html += "<b>Student: " + students[studentDetail].name +"</b><br>";
html += "<ul>";
html += "<li>Track: "+students[studentDetail].track+"</li>";
html += "<li>Points: "+students[studentDetail].points+"</li>";
html += "<li>Achievements: "+students[studentDetail].achievements+"</li>";
html += "</ul>";
}
}
if(html === "" && answer.toUpperCase() !== "QUIT"){html += "<b>Student doesn't exist.</b><br>";}
print(html);
}
to
function getStudent(student){
var report = "<b>Student: " + student.name +"</b><br>";
report += "<ul>";
report += "<li>Track: "+student.track+"</li>";
report += "<li>Points: "+student.points+"</li>";
report += "<li>Achievements: "+student.achievements+"</li>";
report += "</ul>";
return report;
}
while ( true )
{
var html ="";
answer = prompt("Search student record: type student's name or 'Quit' to exit.");
if (answer === null || answer.toLowerCase() === 'quit'){break;}
for (var i = 0;i < students.length ; i++){
student = students[i];
if (student.name.toUpperCase() === answer.toUpperCase())
{
message = getStudent(student);
print(message);
}
}
}
also didn't work at chrome... but works fine with firefox...
I have simplfied by straight using document.write(message) .
var answer = "";
while ( answer.toUpperCase() !== "QUIT" )
{
var html ="";
answer = prompt("Search student record: type student's name or 'Quit' to exit.");
document.write(answer);
}
still doesn't work . it seems the page doesn't load/render until everything finished.
is there any way to have it work on chrome?
thanks
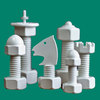
Steven Parker
231,007 PointsNice implementation. Now an even more elegant one (but it may require skills yet to be introduced in the course) would be to have all input and output through the page. You could have a text field in a form to enter the name, and a "search" button which would then populate the output page element.
But as long as the input is done using prompt, I agree that output via alert makes a lot of sense.

Robert Leonardi
17,151 Pointsthank you for the input. I will keep on going with the course . hopefully i can see what you are saying =)
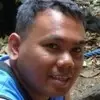
Dennis Amiel Domingo
17,039 PointsHi Robert,
Your modification is superb!
Just a favor please, can you explain these lines of code?
if(display_message != ""){alert(display_message);}
answer = prompt("Search student record: type student's name or 'Quit' to exit.");
if(display_message != ""){display_message =""};
I'm having a hard time determining how it prevents the results from stacking up. Thanks in advance!