Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial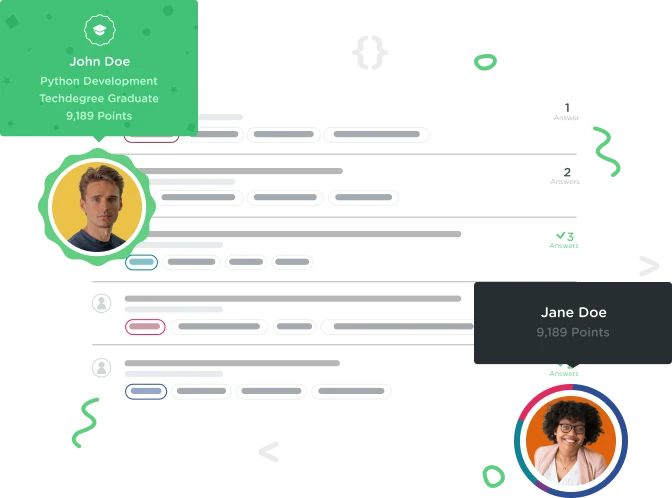

Máté Végh
25,607 PointsHow can I improve my code?
Hi,
I'm looking for parts in my code that can be improved, but not sure what more I could do. Here's my script:
var student;
var search;
var result = '';
var studentExist = false;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('Search student records: type a name or type "quit" to exit');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i++) {
student = students[i];
if (search.toLowerCase() === student.name.toLowerCase()) {
result += getStudentReport(student);
print(result);
studentExist = true;
}
}
result = '';
if (!studentExist) {
result = 'No student named "' + search + '" was found.';
print(result);
}
result = '';
studentExist = false;
}
8 Answers
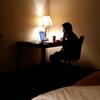
Michael Bianchi
2,488 PointsLook at that. You just reduced all that code down to 12 lines and it's much more efficient. Less calculations = better user experience.
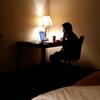
Michael Bianchi
2,488 PointsThere are a few things I'd do, but I would need to see the associated html file for the most accurate assessment.
For your own ease later, initiate your first 4 variables to something nullifying. This will help you debug later.
In your first if statement, in your for loop, check if the StudentExist variable is true first. This is more efficient (easier process) and will be better for the user too because they get the possible result faster.
I don't know your interface or html code, but it looks like it may be a good idea to nest your last if statement inside of the for loop.
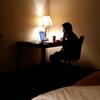
Michael Bianchi
2,488 PointsHowever, I'd like to say that your code is very clean overall and even though there is always room for improvement, you will only benefit marginally from my suggestions because it is quite sound.

Máté Végh
25,607 PointsThanks for your comment! There is a link after my code, so you can test it quickly on CodePen.
I'd really appreciate if you'd demonstrate what you think, because I don't get your points.
- What do you mean by nullifying a variable?
- Could you explain it?
- I think it wouldn't work as it should.
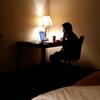
Michael Bianchi
2,488 PointsI'm coming from mostly a C/C++ background and it is a good idea to always initiate your variables to something, especially null or 0. I don't see how it would affect your code negatively because it's already waiting for user input, and would over-ride upon entering those values anyway.
Demonstration:
var student; var search; var result = ''; var studentExist = false;
You initiated the last two variables here, but not the first two.
Now! I didn't see your link to Code Pen initially and I just had a look at it now. There are some things that are going to make your work a heck of a lot easier than making a student variable every time you have to enter new information - consider making a "class" so to speak, or a function. Here's what I mean:
Right now you have all your student data stored in an array like so: <script> var students = [ { name: 'Dave', track: 'Front End Development', achievements: 158, points: 14730 }, etc... </script> Try this instead: <script> function student(name, track, achievements, points){ this.name = name; this.track = track; this.achievements = achievements; this.points = points; } // This is your student object class, and can be re-used to suit whatever dynamic need you have. </script> To initiate, or use, a student object, you do this: <script> var Dave = new student("Dave", "Full Stack", 1568, 39); </script>
You did a great job, though. Is this your first search program?

Máté Végh
25,607 PointsWhen the task was to make an array with at least five student objects, the first thing I wanted to do is a function that creates the student objects inside the array, but I couldn't figure it out.
I've updated my "students.js" with your object class solution and it looks like this:
var Dave = new student('Dave', 'Front End Development', '158', '14730');
var Jody = new student('Jody', 'iOS Development with Swift', '175', '16375');
var Jordan = new student('Jordan', 'PHP Development', '55', '2025');
var John = new student('John', 'Learn WordPress', '40', '1950');
var Trish = new student('Trish', 'Rails Development', '5', '350');
var students = [Dave, Jody, Jody, Jody, Jordan, John, Trish];
function student(name, track, achievements, points) {
this.name = name;
this.track = track;
this.achievements = achievements;
this.points = points;
}
It works just like before but it's more reusable now. I'm wondering if there was a way to have the student variables instantly inside the array, so when I create a new student object, I don't need to add it separately to the students array too. I think it would be great. Let me know if you've got something for that!
And yes, this is my first search program and I think I made about 80% of it by myself.
Many thanks for your help! I'll leave the discussion open for a few days before I give the best answer to see if I can get more comments from others too.

Máté Végh
25,607 PointsI figured it out!
var students = [
new student('Dave', 'Front End Development', '158', '14730'),
new student('Jody', 'iOS Development with Swift', '175', '16375'),
new student('Jordan', 'PHP Development', '55', '2025'),
new student('John', 'Learn WordPress', '40', '1950'),
new student('Trish', 'Rails Development', '5', '350')
];
Woohoo!
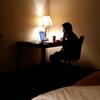
Michael Bianchi
2,488 PointsAwesome job!
In c++ there is the vector class where you can use push and pop commands that will add to or take away from an array. Let me see what I can dig up about javascript in the same regard. I'm sure there is a solution for it.
Just a quick note - I presume that points and numbers in your application (if it were fully-functional) would be subject to change upon interaction and probably shouldn't be string data types, but number data types (in other words, the quotes should not be in quotes for your numerical variables =D).

Máté Végh
25,607 PointsThanks! You are right, those should be numbers instead of strings.
How about this:
var students = [];
var Dave = new student('Dave', 'Front End Development', 158, 14730);
var Jody = new student('Jody', 'iOS Development with Swift', 175, 16375);
var Jordan = new student('Jordan', 'PHP Development', 55, 2025);
var John = new student('John', 'Learn WordPress', 40, 1950);
var Trish = new student('Trish', 'Rails Development', 5, 350);
function student(name, track, achievements, points) {
this.name = name;
this.track = track;
this.achievements = achievements;
this.points = points;
students.push(this);
}
It works!
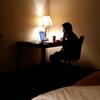
Michael Bianchi
2,488 PointsMmhmm... Now gimme that best answer award =D
Kidding, of course. It looks highly professional and easy to read as English. Can you re-link your updated Code Pen for this? I want to help you test it further and see what else we can optimize. I'm kind of having fun with it now. =D

Máté Végh
25,607 PointsSir, you deserved the Best Answer award! You've already got it :D
Here is the up to date version: http://codepen.io/anon/pen/JoBNpm
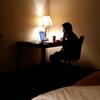
Michael Bianchi
2,488 PointsCoolness!
Okay, so from a programmer standpoint, you've done your job. But from the user-standpoint, it may not be finished yet. What I mean is the prompt is persistent. It looks well and readable in my browser, on a 15 inch laptop, but that persistent prompt wouldn't be easy to read on small devices. Once your program finds a student, all prompt messages should probably go away.
The "issue" is somewhere here (even though you've really already completed the challenge):
while (true) {
search = prompt('Search student records: type a name or type "quit" to exit');
if (search === null || search.toLowerCase() === 'quit') {
``` }```
```for (var i = 0; i < students.length; i++) {```
```student = students[i];```
``` if (search.toLowerCase() === student.name.toLowerCase()) {```
``` result += getStudentReport(student);```
``` print(result);```
``` studentExist = true;```
``` }```
``` }```
``` result = '';```
```if (!studentExist) {```
``` result = 'No student named "' + search + '" was found.';```
```print(result);```
```}```
``` result = '';```
```studentExist = false;```
```}```
I think the solution might be to change the initial while loop's condition. I'm playing around with it now in Notepad++.
P.S: I understand that typing 'quit' will exit the prompt but there's no suggestion of it. It should be more intuitive.
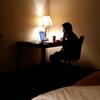
Michael Bianchi
2,488 PointsI made a mistake, there is obvious suggestion of it. I was wrong. Forget everything I just said about that. Would you consider turning this into a useful project, perhaps to use in a small bookstore or public library system?

Máté Végh
25,607 PointsActually I don't have any further plans with it, but if I wanted to make it further, the next step would be to turn the prompt dialog box to a form input to make it more user friendly.
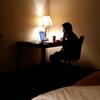
Michael Bianchi
2,488 PointsI would do the same thing. And I believe prompts and alert boxes can be styled in css. I recommend playing with it more. What track are you taking? I'm doing PHP Development.

Máté Végh
25,607 PointsYeah, I definitely will play more with it!
You are right, they can be styled in CSS, but first you need to overwrite the default functions for the dialog boxes.
I'm doing the Front End Development track. I'm almost done with it. After this I think I will take the Wordpress Development track.
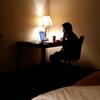
Michael Bianchi
2,488 PointsWell when you're done, I'd like to work with you as a team to find a gap in the market where our skills can offer a solution. I'm mostly doing back-end things so I believe we would make a formidable team and then split the profits, of course. I'll put my email in my profile if you're interested.

Máté Végh
25,607 PointsSounds great, but my time is very limited at the moment to be honest. I'll keep that in mind as an opportunity and hit you up when I'm ready for some really cool project.
Máté Végh
25,607 PointsMáté Végh
25,607 PointsCool, thanks for everything!