Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial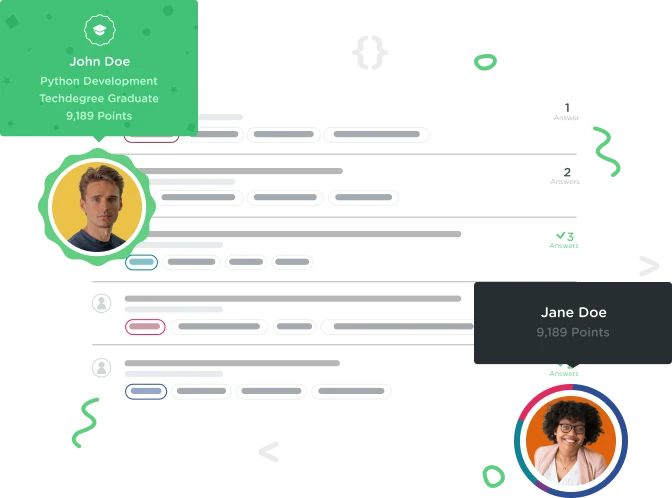
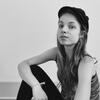
Kate Johnson
Python Development Techdegree Graduate 20,155 PointsHow can I optimize this code?
So I wrote this function to display feedback when a user clicks the button with the correct answer. However, it currently works where I have to set the correct answer in the quizQuestions array to the exact string of the correct answer from the answers object instead of the letter to the corresponding answer. I pasted the javaScript here. The quiz works for question 1 but not for other questions that have only the letter string as the correct answer. Does anyone have any ideas how to keep a letter as the correct answer rather than having the literal correct answer string? Thanks!
var quizQuestionsContainer = document.getElementById("quiz");
var quizResults = document.getElementById("results");
var resultsText = document.getElementById("results-text")
var quizQuestion = document.getElementById("quiz-question");
var buttonsContainer = document.getElementById("buttons-container");
var startButton = document.getElementById("start");
var questionCounter = 0;
var currentQuestion = {};
startButton.addEventListener("click", function() {
buttonsContainer.removeChild(startButton);
quizResults.removeChild(resultsText);
displayQuestion();
});
var setCurrentQuestion = function() {
var currentQuestion = quizQuestions[questionCounter];
return currentQuestion;
}
var displayQuestion = function() {
var currentQuestion = setCurrentQuestion();
for (letter in currentQuestion.answers) {
quizQuestion.innerText = currentQuestion.question
var optionButton = document.createElement("button")
optionButton.className = "option-button"
optionButton.textContent = currentQuestion.answers[letter];
buttonsContainer.appendChild(optionButton);
optionButton.addEventListener("click", optionButtonHandler);
}
};
var optionButtonHandler = function (event) {
var currentQuestion = setCurrentQuestion();
var userAnswer = event.target
console.log(userAnswer);
if (userAnswer.textContent === currentQuestion.correctAnswer) {
resultsText.innerText = currentQuestion.feedback
quizResults.appendChild(resultsText);
} else {
resultsText.innerText = "Whoops wrong answer!"
quizResults.appendChild(resultsText);
}
questionCounter ++;
}
// quiz questions
var quizQuestions = [
{
question: "Inside the HTML document, where do you place your JavaScript code?",
answers: {
a: "Inside the <script> element",
b: "Inside the <link> element",
c: "Inside the <head> element",
d: "In the <footer> element"
},
correctAnswer: "Inside the <script> element",
feedback: "Nice job! You place your JavaScript inside the <script> element of the HTML Document is correct."
},
{
question: "What operator is used to assign a value to a declared variable?",
answers: {
a: "Double-equal (==)",
b: "Colon (:)",
c: "Equal sign (=)",
d: "Question mark (?)"
},
correctAnswer: "c",
feedback: "Awesome! The correct way to assign a variable is with an equal sign(=)."
},
{
question: "What are the six primitive data types in JavaScript?",
answers: {
a: "sentence, float, data, bigInt, symbol, undefined",
b: "string, number, boolean, bigInt, symbol, undefined",
c: "sentence, int, truthy, bigInt, symbol, undefined",
d: "string, num, falsy, bigInt, symbol, undefined"
},
correctAnswer: "b",
feedback: "Stellar! JavaScript has a total of six primitive data types: string, number, boolean, bigInt, symbol, undefined."
},
1 Answer
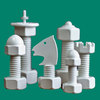
Steven Parker
231,008 PointsOne way to accomplish this would be to assign a value property to the buttons to identify them, and test that against the "correctAnswer" instead of the button text:
// in the displayQuestion function:
quizQuestion.innerText = currentQuestion.question; // note: do this only ONCE (out of loop)
for (letter in currentQuestion.answers) {
var optionButton = document.createElement("button")
optionButton.className = "option-button"
optionButton.textContent = currentQuestion.answers[letter];
optionButton.value = letter; // give the button a value
// ...
// then, in the optionButtonHandler:
if (userAnswer.value === currentQuestion.correctAnswer) { // value instead of textContent
For future questions, post the whole code, or make a snapshot of your workspace and post the link to it here.
Kate Johnson
Python Development Techdegree Graduate 20,155 PointsKate Johnson
Python Development Techdegree Graduate 20,155 Pointsawesome thank you for the help!