Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial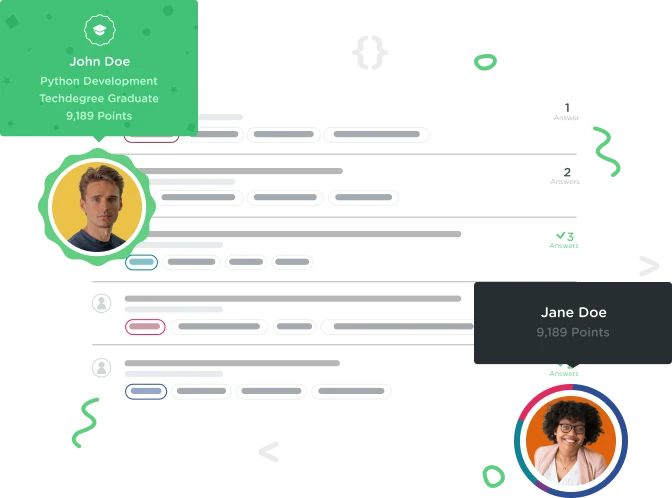

Nyong Otudor
2,332 PointsHow could I have shortened all this for this challenge with what we've learned so far ?
Is there anything I could've done to shorten this up with what we've learned up to this challenge ?
/*
This program will ask you a 5 questions about sounds and the animals these belong to
and will tell you how many questions you got right and your overall score once you're done
*/
var score = 0; //score tracker
var question = 5; //question tracker
//Ask these maggots the questions and turn all answers into lower case along with subtracting 1 after each question is answered from the 'question' variable
var q1 = prompt(' What animal makes a RUFF RUFF sound? ')
q1 = q1.toLowerCase();
question -= 1;
alert(" You got " + question + " left ");
var q2 = prompt(' What animal makes a QUACK QUACK sound? ');
q2 = q2.toLowerCase();
question -= 1;
alert(" You got " + question + " left ");
var q3 = prompt(' What animal makes a OINK OINK sound?');
q3 = q3.toLowerCase();
question -= 1;
alert(" You got " + question + " left ");
var q4 = prompt(' What animal makes a MOOOOO sound?');
q4 = q4.toLowerCase();
question -= 1;
alert(" You got " + question + " left ");
var q5 = prompt(' hat animal makes a MEOWWWW sound?');
q5 = q5.toLowerCase();
question -= 1;
alert(" You got " + question + " left ");
console.log(score); //make sure at this point the score is still the same
//Nest some if statements baby and ask if the answers to each question was right and update console with score after each validation
if ( q1 === 'dog') {
score = score + 1
console.log(score);
if ( q2 === 'duck') {
score = score + 1
console.log(score);
}if ( q3 === 'pig') {
score = score + 1
console.log(score);
}if ( q4 === 'cow') {
score = score + 1
console.log(score);
}if ( q5 === 'cat') {
score = score + 1
console.log(score);
}
}
//Tell the user how many questions they got right out of 5 using the score vairable since it's equivilent to how many questions there are
document.write(" You got " + score + " out of 5 question correct " );
//Now give out those medals
if ( score === 0 ) {
document.write(" <p>Your score is " + score + " and you deserve a squat shit of a medal for it </p>");
} else if ( score > 0 && score < 3 ) {
document.write(" <p>Your score is " + score + " and you deserve a bronze medal for it </p>");
} else if ( score > 3 && score < 5) {
document.write(" <p>Your score is " + score + " and you deserve a silver medal for it</p>");
} else if ( score === 5 ){
document.write("<h1> You have a perfect score of " + score + " you get a gold crown buddy");
}
2 Answers

Nyong Otudor
2,332 Pointsokay I haven't been taught in this course the " each " method, and i'll see if that nested || statement add 1 to the score for each true statement , thanks Gil !

Gil Huss
2,750 PointsGlad I could help :)
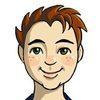
Alok Joshi
4,944 Points/*
This program will ask you a 5 questions about sounds and the animals these belong to
and will tell you how many questions you got right and your overall score once you're done
*/
var score = 0; //score tracker
var question = 0; //question tracker
var qa = [
{
question: "Question 1",
answer: "Answer"
},
{
question: "Question 1",
answer: "Answer"
},
{
question: "Question 1",
answer: "Answer"
},
{
question: "Question n",
answer: "Answer n"
}
];
//Ask these maggots the questions and turn all answers into lower case along with subtracting 1 after each question is answered from the 'question' variable
do {
var q = prompt(qa[question].question);
q = q.toLowerCase();
question += 1;
if ( q === qa[question].answer) {
score = score + 1
console.log(score);
}
} while( question <= qa.length );
//Tell the user how many questions they got right out of 5 using the score vairable since it's equivilent to how many questions there are
document.write(" You got " + score + " out of 5 question correct " );
//Now give out those medals
if ( score === 0 ) {
document.write(" <p>Your score is " + score + " and you deserve a squat shit of a medal for it </p>");
} else if ( score > 0 && score < 3 ) {
document.write(" <p>Your score is " + score + " and you deserve a bronze medal for it </p>");
} else if ( score > 3 && score < 5) {
document.write(" <p>Your score is " + score + " and you deserve a silver medal for it</p>");
} else if ( score === 5 ){
document.write("<h1> You have a perfect score of " + score + " you get a gold crown buddy");
}
This much you can reduce this code.
Gil Huss
2,750 PointsGil Huss
2,750 PointsI think you could shorten a lot of it. your vars can be shortened:
var q1 = prompt(' What animal makes a RUFF RUFF sound? '), q2 = prompt(' What animal makes a QUACK QUACK sound? '), q3 = prompt(' What animal makes a OINK OINK sound?'), q4 = prompt(' What animal makes a MOOOOO sound?'), q5 =prompt(' hat animal makes a MEOWWWW sound?') ;
[q1 && q2 && q3 && q4 && q5 += each().toLowerCase();] <-- not sure about this.
Here's a way to shorten your if() code, i'm just not sure about the score, if it counts them all or not, else you maybe need an ".each()" function to recognize them all.
if ( q1 === 'dog' || q2 === 'duck' || q3 === 'pig' || q4 === 'cow' || q5 === 'cat' ){ score += 1 console.log(score); }
I hope I helped you a bit!