Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial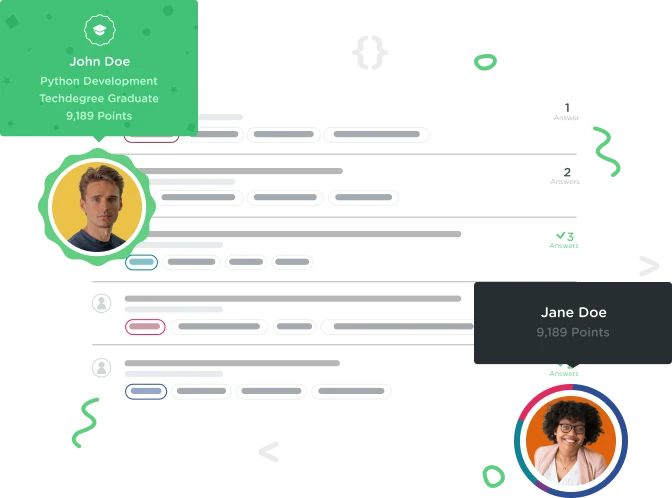

Mykiel Horn
4,014 PointsHow do I break out of this for loop?
I am trying to use break in this for loop when it reaches its current item "STOP".
def loopy(items):
# Code goes here
for thing in items:
print(thing)
else thing == "STOP":
break
2 Answers

Afloarei Andrei
5,163 PointsIn you'r "for" loop you have to do "if" and "else". "if the current item from items is == to the string "STOP", break the loop" "else print current item from items"

Ben Moore
22,588 PointsMykiel, your original syntax isn't structured properly. You are trying to break the loop for a specific reason. Because you are looking for 'STOP', you need to use the break with the 'if' clause. Otherwise, any combination will never fail the 'if' and move on to the 'else'. The argument provided to the 'loopy' function always passes in a specific order from 'if', then 'else', for each item in items.
Your updated syntax is still wrong. You want to replace 'print(items)' with 'print(things)' and remove the original 'print(things)' altogether above the 'if' statement. Your current syntax is printing each 'thing' before breaking. So, it will print 'STOP' before it breaks the loop.
items = ["STOP"] def loopy(items): # Code goes here for thing in items: print(thing) if items == "STOP" : break else: print(items)
Should instead try using something like the following:
items = ['stop', 'Stop', 'Go', 'GO', 'Hold', 'You shall not pass', 'STOP', 'Whoops!']
def loopy(items):
for item in items:
if item == 'STOP':
break
else:
print(item)
loopy(items)
> stop
> Stop
> Go
> GO
> Hold
> You shall not pass
Keep in mind it is better practice to use singular ('item') and plural ('items') forms when using loops and for other individuals of a set/series usages. It makes it clearer that the item is part of the list of items.
Also note that if you are wanting to catch all instances of 'stop'/'STOP'/'Stop'/etc. (with only those four characters in order, regardless of capitalization), you could make this one change:
if item.upper() == 'STOP':
OR use this variant:
if item.lower() == 'stop':
Mykiel Horn
4,014 PointsMykiel Horn
4,014 Pointsitems = ["STOP"] def loopy(items): # Code goes here for thing in items: print(thing) if items == "STOP" : break else: print(items)
It keeps saying it didn't find the right items being printed. How can this not be right? Im confused.