Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial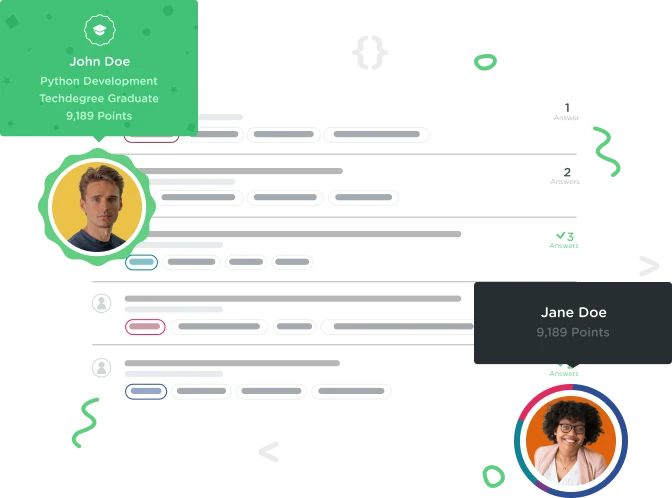
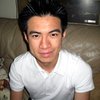
jason chan
31,009 PointsHow do I fix my chat to show previous strings?
<style>
body {
font-family: arial,sans-serif;
margin:0;
background-color: #f2f2f2;
}
#header {
width: 100%;
height: 60px;
background-color: grey;
box-shadow: 0px 4px 2px #333;
}
#header>h1 {
width:1024px;
margin:0px auto;
color:white;
padding:12px;
}
#container{
width:1024px;
height: 500px;
margin:0px auto;
margin-top: 20px;
background-color:white;
border:1px solid #333;
overflow: scroll;
}
#controls{
width:1024px;
margin:0px auto;
}
textarea{
resize:none;
width: 940px;
}
#send{
font-size: 24px;
position: absolute;
}
.username{
color:blue;
font-weight: bold;
}
.bot{
color:red;
font-weight: bold;
}
</style>
<script>
var username = "";
function send_message(message){
var prevState = $("#container").html();
if (prevState.length > 3) {
prevState = prevState + "<br>";
}
$("#container").html(prevState + username + message);
$("#container").html("<span class = 'bot'>Chatbot: </span>" + message);
}
function get_username() {
send_message("Hello, what's your name?");
}
function ai(message) {
if (username.length < 3){
username = message;
send_message("Nice to meet you " + username + ", how are you doing?");
}
if (message.indexOf("how are you")>=0){
send_message("Thanks, I am good!");
}
if (message.indexOf("good")>=0){
send_message("I'm fine too!");
}
if (message.indexOf("time")>=0){
var date = new Date();
var h = date.getHours();
var m = date.getMinutes();
send_message("Current time is: "+h+":"+m);
}
if (message.indexOf("ratchet")>=0){
send_message("no ratchets here");
}
if (message.indexOf("today")>=0){
var today = new Date();
var day = today.getDate();
var month = today.getMonth();
var year = today.getFullYear();
send_message("Today date is " + month +"/"+ day + "/" + year);
}
}
$(function(){
get_username();
$("#textbox").keypress(function(event){
if (event.which == 13){
if ($("#enter").prop("checked") ) {
$("#send").click();
event.preventDefault();
}
}
});
$("#send").click(function(){
var username = "<span class = 'username'>You: </span>";
var newMessage = $("#textbox").val();
$("#textbox").val("");
$("#container").scrollTop($("#container").prop("scrollHeight"));
ai(newMessage);
});
});
</script>
<div id="header">
<h1>jquery chatbox 1.0</h1>
</div>
<div id="container">
</div>
<div id="controls">
<textarea name="" id="textbox" placeholder="enter text here"></textarea>
<button id="send">send</button>
<br>
<input checked type="checkbox" id="enter">
<label for="">send on enter</label>
</div>
How do i fix this so my previous statements show on my chat box?
2 Answers
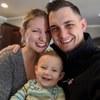
Zac Mackey
11,392 PointsHi Jason!
I've made a couple changes to your code so I put them in a shareable workspace.
Look at the JS folder and the script.js file. I've commented the changes I've made. I didn't fix all the issue (i.e. the username not displaying correctly) but you can at least see all the messages posted.
Your first issue was you were using .html() instead of .append() in your send_message function which caused the messages to be overwritten each time a new one was passed as the argument.
Also, since your first function was designed for the Chatbot I set up a second function specifically for the user. You could have this pass the username assigned during the chat sequence, but I didn't touch that part just yet.
Anyway, I left notes next to my changes, if you have any questions let me know. I tested it out and now all messages can be seen in the chatbox, both from the AI and the user, including messaged which do not get a response from the AI.
Hope that helps!
EDIT*** When you open the workspace I shared you'll just need to "Fork" it by clicking in the upper right corner and save it under your workspaces. You can then open it and preview the application in the browser.
Or you can just copy and paste into your favorite text editor, whichever works best for you.
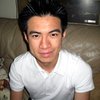
jason chan
31,009 PointsThank you very much. You fixed my script.